Default trait in Rust
In Rust, the Default
trait provides a way to set default values for different types. A default value is like a starting point when you don’t have a specific value in mind.
std::default::Default
Read the Rust Documentation : https://doc.rust-lang.org/stable/std/default/trait.Default.html
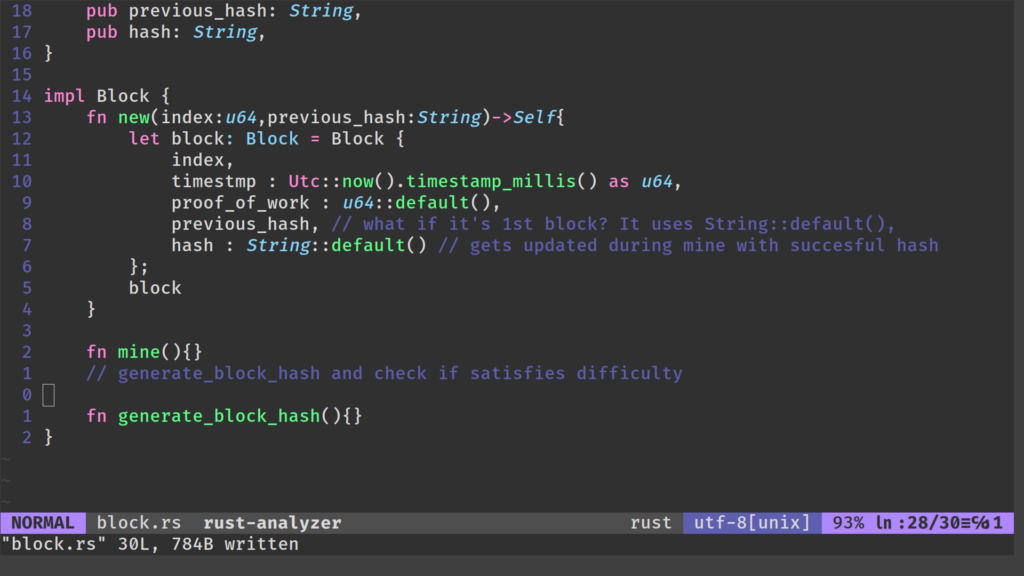
Use case for Default()
We use a temporary value provided by default and later it gets replaced after a successful block hash satisfies the proof of work.
Primitive types like integers, floating-point numbers, and booleans have default values that are defined by the Default
trait. Here’s how it works with some common primitive types:
#![allow(unused)]
fn main() {
let i: i8 = Default::default();
let (x, y): (Option<String>, f64) = Default::default();
let (a, b, (c, d)): (i32, u32, (bool, bool)) = Default::default();
println!("i: {}", i);
println!("x: {:?}", x);
println!("y: {}", y);
println!("a: {}", a);
println!("b: {}", b);
println!("c: {}", c);
println!("d: {}", d);
}
i: 0
x: None
y: 0
a: 0
b: 0
c: false
d: false
The Default
trait provides a way to set default values for different types. A default value is like a starting point when you don’t have a specific value in mind.
For example, think of a new car. When you buy a car, it doesn’t have any specific features like color or options assigned to it yet. It starts as a “default” car, and you can customize it later.
In Rust, when you create a variable or structure, you can use Default
to set initial values. If you have a Car
structure, you might use the Default
trait to set the initial values for the car’s color, model, and other features to reasonable default values, like “gray” for color and “basic” for the model.
Here’s how you could use it in code:
use std::default::Default;
struct Car {
color: String,
model: String,
// Other car properties...
}
impl Default for Car {
fn default() -> Car {
Car {
color: String::from("gray"),
model: String::from("basic"),
// Set other properties to their default values
}
}
}
fn main() {
let my_car: Car = Default::default(); // Create a new car with default values
println!("{:}", my_car.color);
println!("{:}", my_car.model);
}
Summary
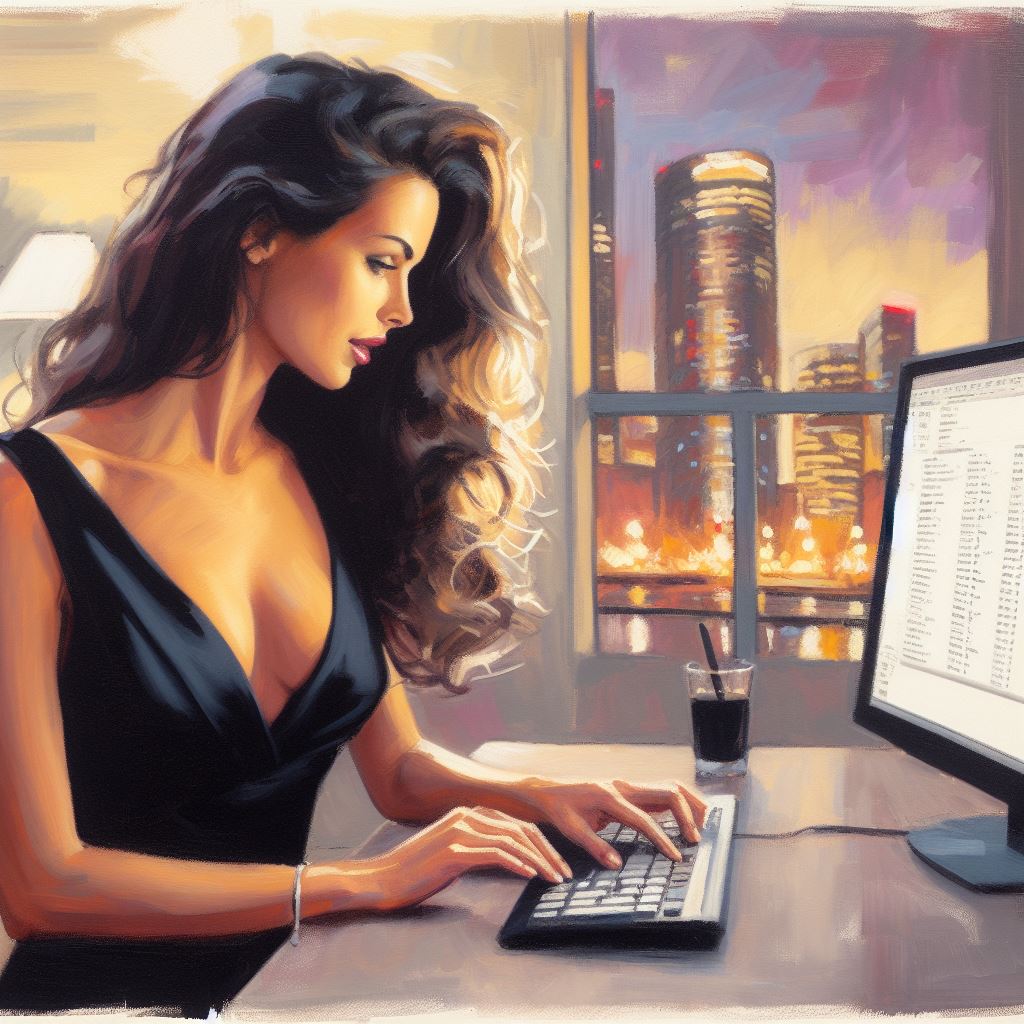
In Rust, “default” often refers to the default values and behavior for primitive data types and custom structs. Here’s a summary of “default” in Rust, specifically with regards to primitives and structs:
Primitives:
- Primitives have default values that are automatically assigned if a value is not explicitly specified.
- Common primitive data types include integers, floating-point numbers, booleans, and characters.
- Default values for primitives are as follows:
- Integers: 0
- Floating-point numbers: 0.0
- Booleans: false
- Characters: ‘\0’ (null character)
Custom Structs:
- Custom structs, defined by users, can also have default values determined by the struct’s implementation.
- Structs must implement the
Default
trait to specify default values. - You can derive the
Default
trait for a struct using the#[derive(Default)]
attribute, which will assign default values to all fields. - Alternatively, custom implementations of the
Default
trait can be provided for fine-grained control over default values for each field.