as_ref() and as_mut()
In Rust as_ref()
and its mutable counterpart as_mut()
are commonly used with Option
and Result
types in Rust. These methods are used to obtain references to the values contained within these types without taking ownership of the values. Here’s a brief explanation of their common use cases:
as_ref()
with Option
:
- It allows you to obtain an
Option<&T>
whereT
is the type of the value contained in theOption
. - Useful when you want to work with the value inside the
Option
without transferring ownership or when you want to chain multiple operations on theOption
without moving it.
as_mut()
with Option
:
- Similar to
as_ref()
, but it provides a mutable reference (Option<&mut T>
) to the value. - Useful when you need to modify the value inside the
Option
.
as_ref()
and as_mut()
with Result
:
- Similar to their use with
Option
, you can obtain references to the value or error contained in aResult
without taking ownership. - Useful when you want to inspect or manipulate the result without consuming it.
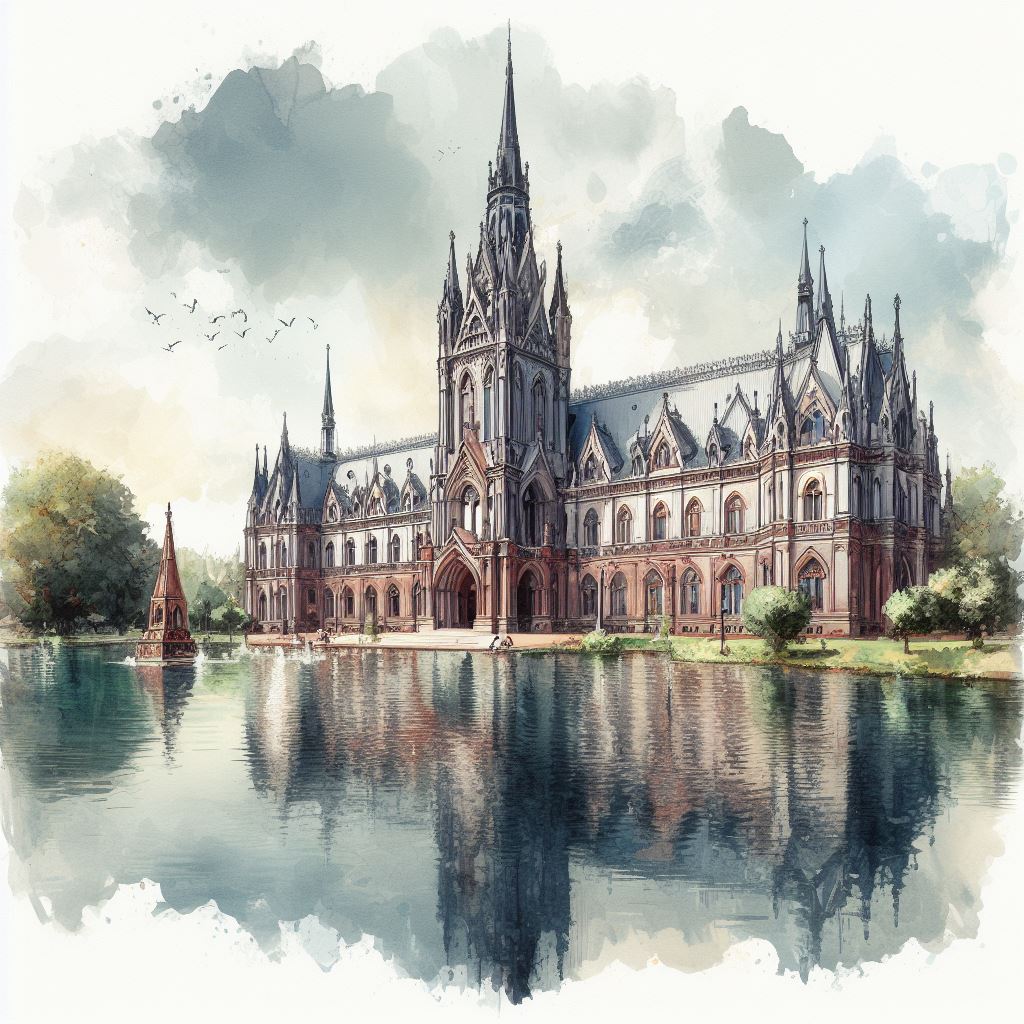
These methods are particularly helpful when you want to work with the values inside Option
and Result
without moving or altering the original values, which is a common use case in Rust’s ownership system.
struct MyStruct {
url: Option<String>,
}
impl MyStruct {
fn new(url: Option<String>) -> Self {
MyStruct { url }
}
fn print_url(&self) {
if let Some(url) = self.url.as_ref() {
println!("The URL is: {}", url);
} else {
println!("No URL found.");
}
}
}
fn main() {
// Create an instance of MyStruct with a Some value.
let my_instance_with_url = MyStruct::new(Some("https://example.com".to_string()));
my_instance_with_url.print_url();
// Create an instance of MyStruct with None value.
let my_instance_without_url = MyStruct::new(None);
my_instance_without_url.print_url();
}