Learn SurrealDB
Described as “A scalable, distributed, collaborative, document-graph database, for the realtime web” – written in Rust
Let’s take a look, and see if it’s easy to learn and suitable for an upcoming project.
SurrealDB Rust SDK – Example Code
Features:
https://surrealdb.com/features
You can test here :
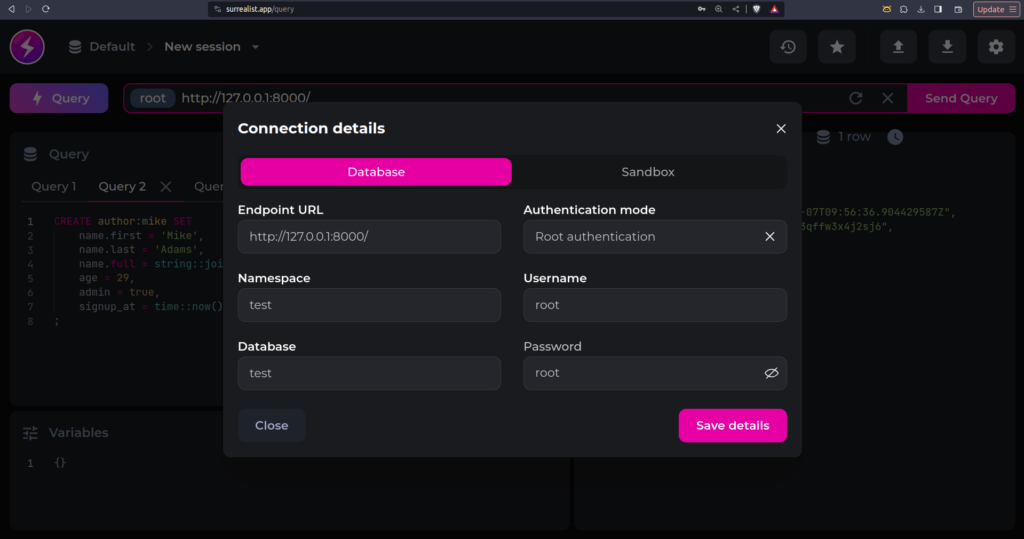
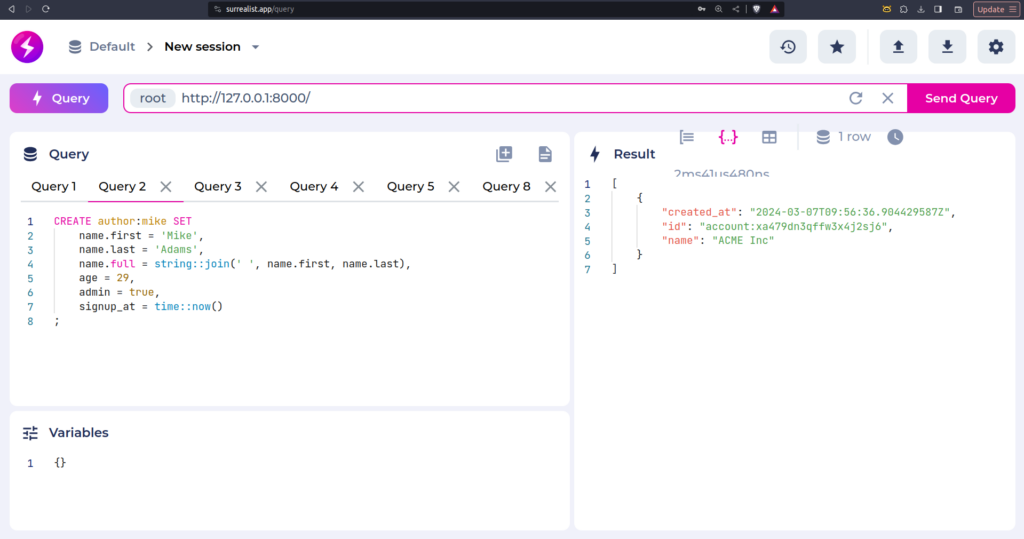
Install
https://surrealdb.com/docs/surrealdb/installation/overview
curl -sSf https://install.surrealdb.com | sh
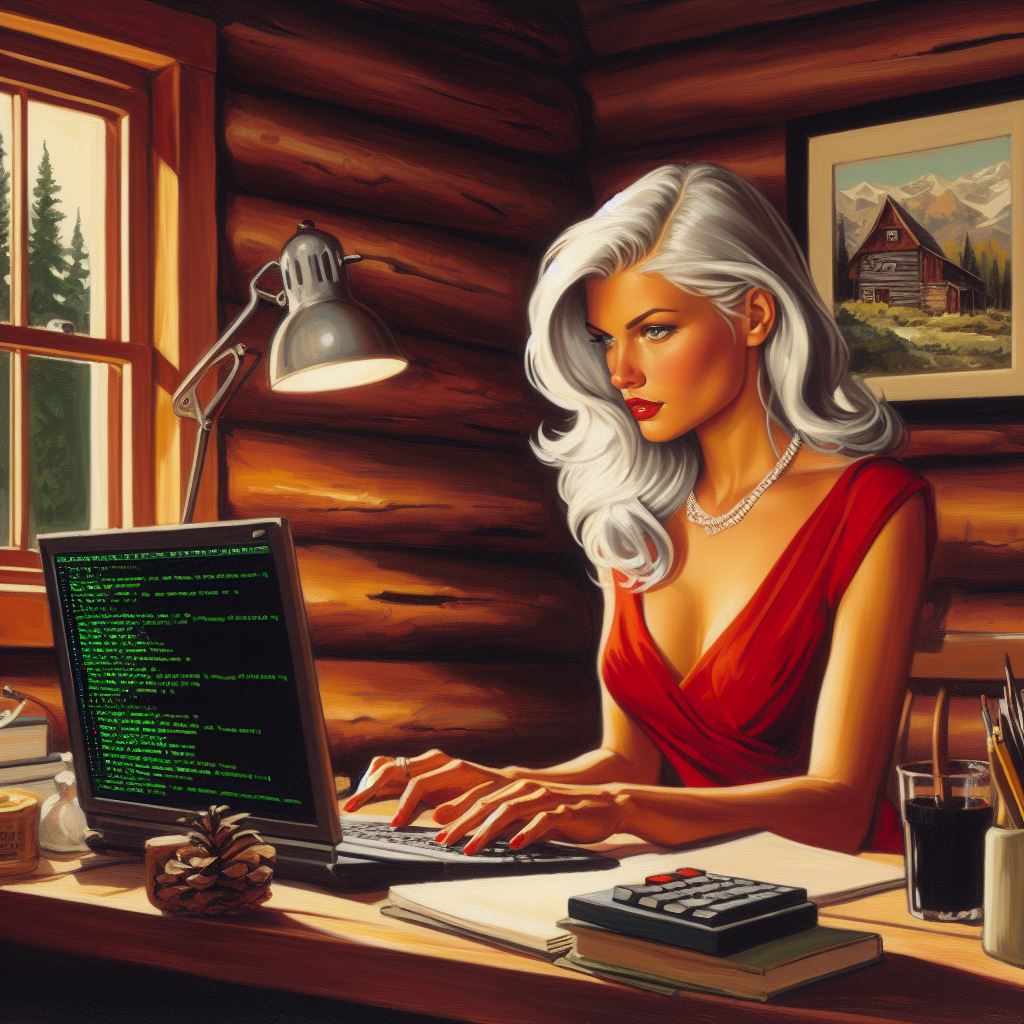
Start the database
surreal start --log info --user root --pass root file:mydatabase.db
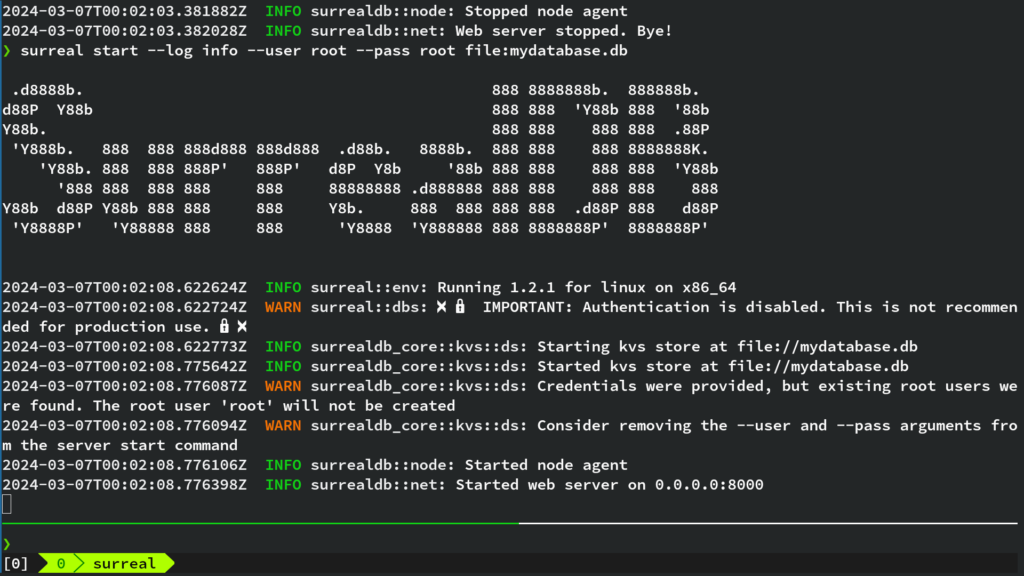
Add a ‘record’ to the Database
CREATE account SET
name = 'ACME Inc',
created_at = time::now()
;
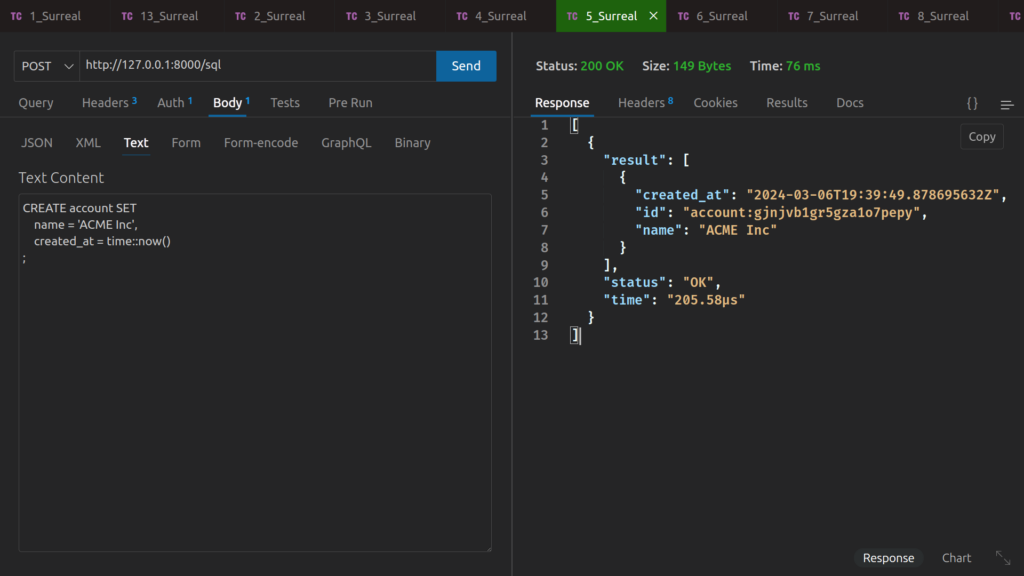
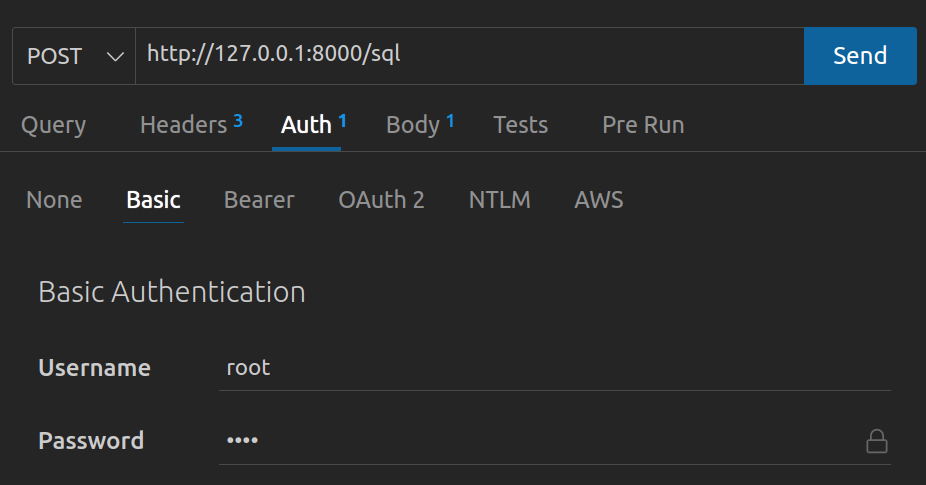
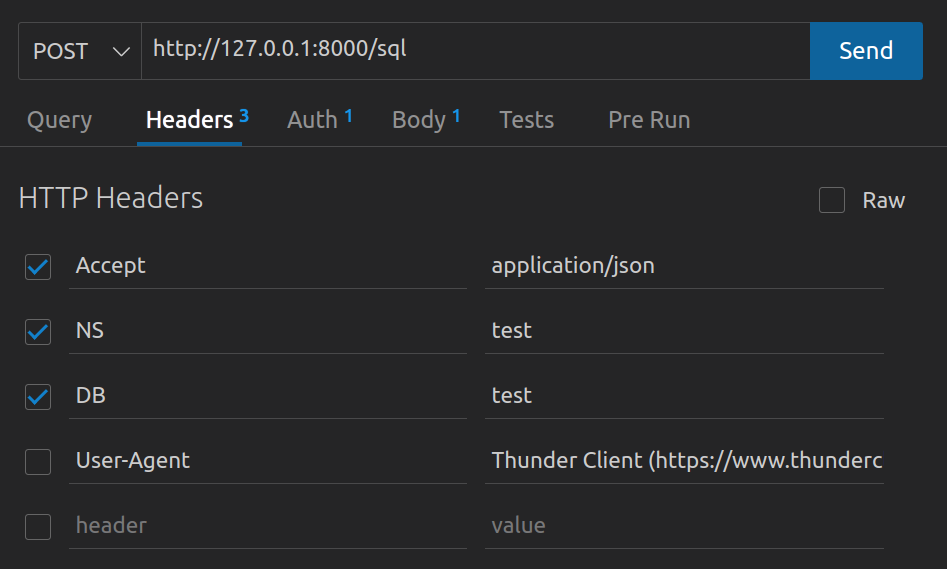
Select from
SELECT * FROM article WHERE author.age < 30 FETCH author, account;
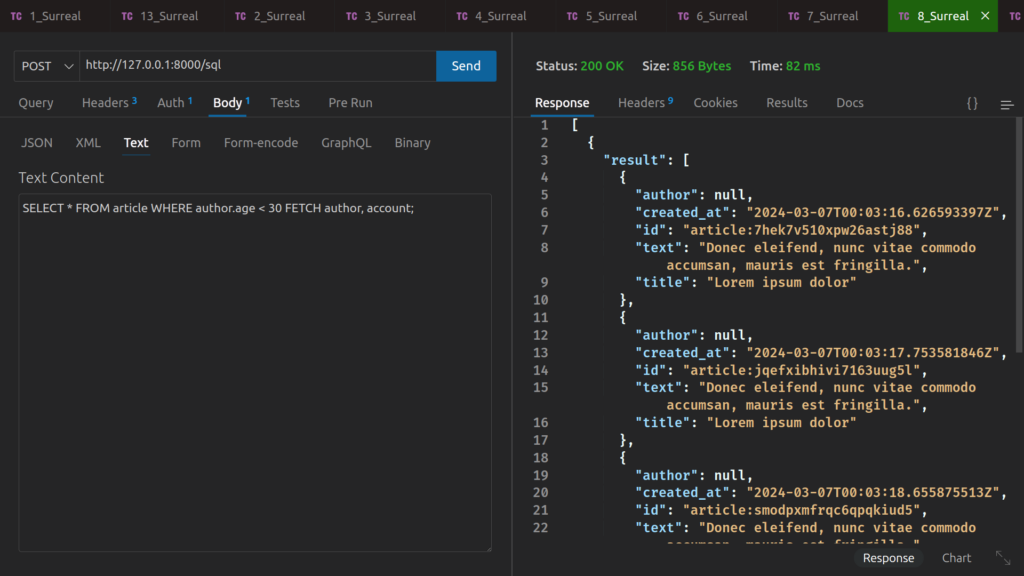
SELECT count() FROM article GROUP BY count;
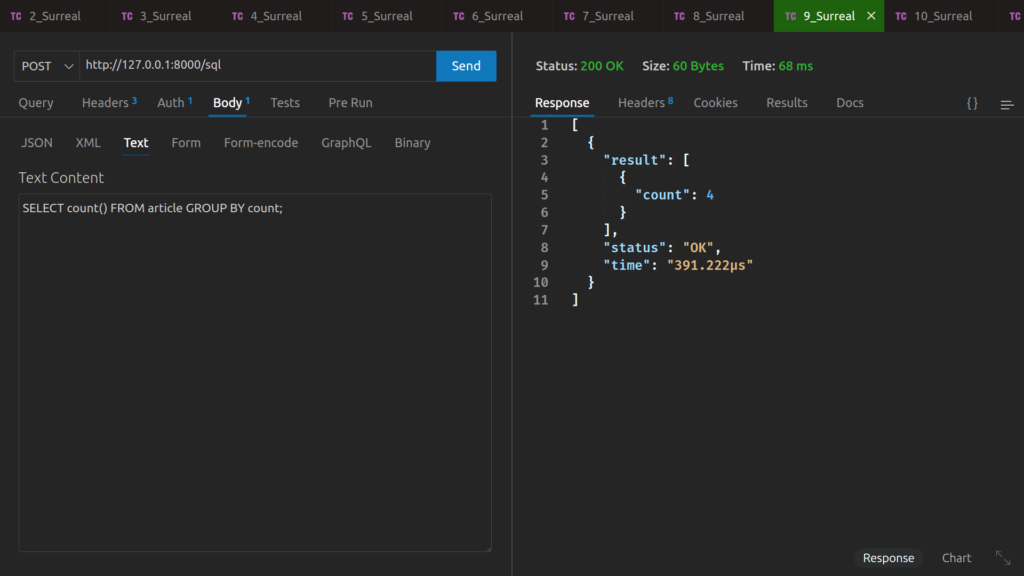
SELECT array::group(name) FROM author GROUP ALL;
[
{
"result": [
{
"array::group": [
{
"first": "Mike",
"full": "Mike Adams",
"last": "Adams"
},
{
"first": "Mike_id",
"full": "Mike_id Adams",
"last": "Adams"
}
]
}
],
"status": "OK",
"time": "595.39µs"
}
]
SELECT id FROM author WHERE name.first = 'Mike';
[
{
"result": [
{
"id": "author:mike"
}
],
"status": "OK",
"time": "147.037µs"
}
]
SELECT * FROM article WHERE author.age < 30 FETCH author, account;
[
{
"result": [
{
"author": null,
"created_at": "2024-03-07T00:03:16.626593397Z",
"id": "article:7hek7v510xpw26astj88",
"text": "Donec eleifend, nunc vitae commodo accumsan, mauris est fringilla.",
"title": "Lorem ipsum dolor"
},
{
"author": null,
"created_at": "2024-03-07T00:03:17.753581846Z",
"id": "article:jqefxibhivi7163uug5l",
"text": "Donec eleifend, nunc vitae commodo accumsan, mauris est fringilla.",
"title": "Lorem ipsum dolor"
},
{
"author": null,
"created_at": "2024-03-07T00:03:18.655875513Z",
"id": "article:smodpxmfrqc6qpqkiud5",
"text": "Donec eleifend, nunc vitae commodo accumsan, mauris est fringilla.",
"title": "Lorem ipsum dolor"
},
{
"author": null,
"created_at": "2024-03-06T23:51:11.776807515Z",
"id": "article:tc5w56rka3gfbb2r2f09",
"text": "Donec eleifend, nunc vitae commodo accumsan, mauris est fringilla.",
"title": "Lorem ipsum dolor"
}
],
"status": "OK",
"time": "258.375µs"
}
]
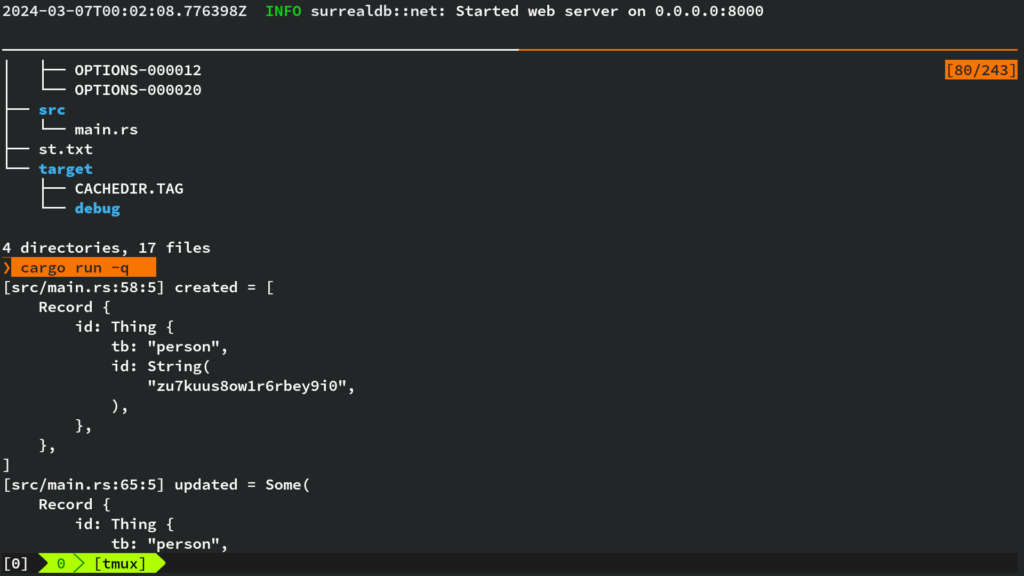
use serde::{Deserialize, Serialize};
use surrealdb::engine::remote::ws::Ws;
use surrealdb::opt::auth::Root;
use surrealdb::sql::Thing;
use surrealdb::Surreal;
#[derive(Debug, Serialize)]
struct Name<'a> {
first: &'a str,
last: &'a str,
}
#[derive(Debug, Serialize)]
struct Person<'a> {
title: &'a str,
name: Name<'a>,
marketing: bool,
}
#[derive(Debug, Serialize)]
struct Responsibility {
marketing: bool,
}
#[derive(Debug, Deserialize)]
struct Record {
#[allow(dead_code)]
id: Thing,
}
#[tokio::main]
async fn main() -> surrealdb::Result<()> {
// Connect to the server
let db = Surreal::new::<Ws>("127.0.0.1:8000").await?;
// Signin as a namespace, database, or root user
db.signin(Root {
username: "root",
password: "root",
})
.await?;
// Select a specific namespace / database
db.use_ns("test").use_db("test").await?;
// Create a new person with a random id
let created: Vec<Record> = db
.create("person")
.content(Person {
title: "Founder & CEO",
name: Name {
first: "Tobie",
last: "Morgan Hitchcock",
},
marketing: true,
})
.await?;
dbg!(created);
// Update a person record with a specific id
let updated: Option<Record> = db
.update(("person", "jaime"))
.merge(Responsibility { marketing: true })
.await?;
dbg!(updated);
// Select all people records
let people: Vec<Record> = db.select("person").await?;
dbg!(people);
// Perform a custom advanced query
let groups = db
.query("SELECT marketing, count() FROM type::table($table) GROUP BY marketing")
.bind(("table", "person"))
.await?;
dbg!(groups);
Ok(())
}