Lazy Iterators in Python
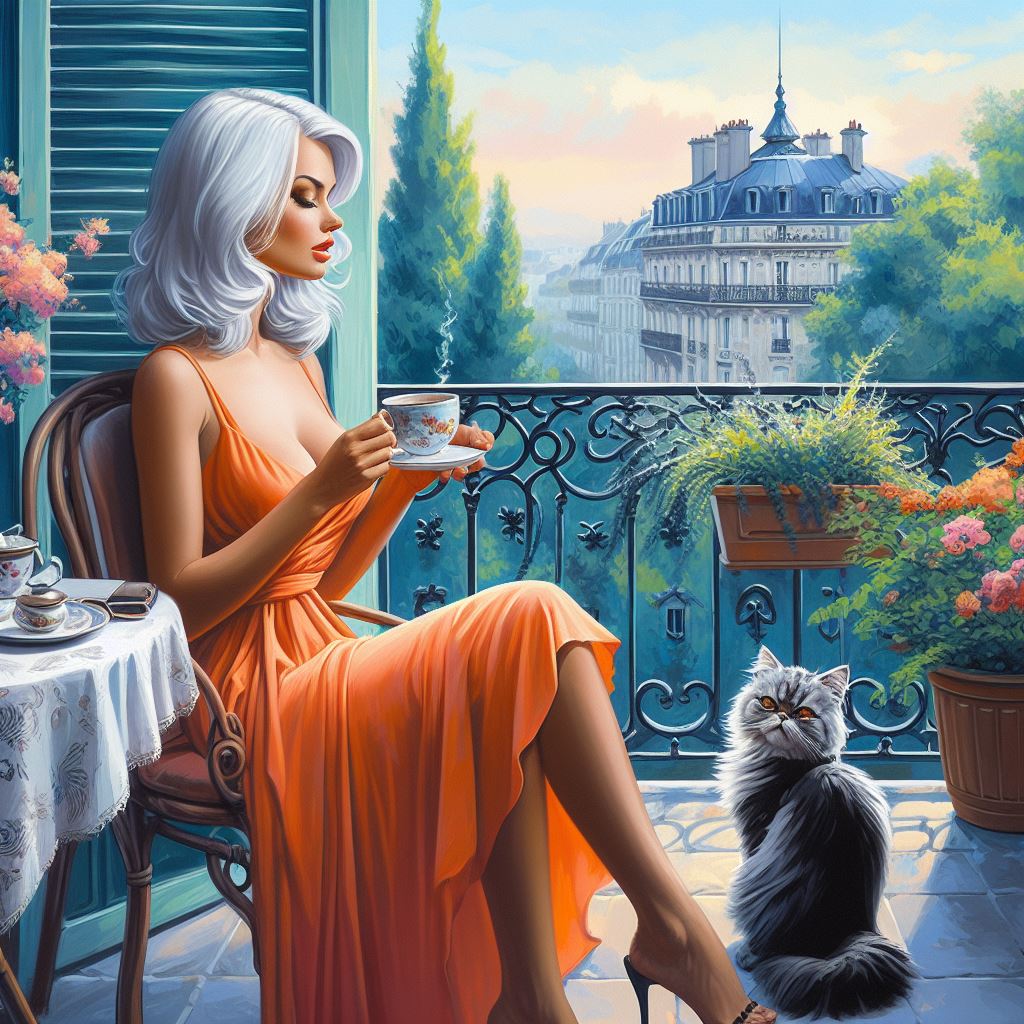
A lazy iterator in Python is often implemented using a generator expression, denoted by (...)
.
https://www.youtube.com/watch?v=9jEvIAsYr5w
A generator expression is similar to a list comprehension but produces values lazily, one at a time, as they are requested. Let’s break down the concept and create a simple example.
Generator Expression Syntax:
A generator expression is enclosed in parentheses and has a similar syntax to a list comprehension.
lazy_iterator = (expression for item in iterable if condition)
Key Points:
- Lazy Evaluation: The values are generated one at a time as the iterator is iterated over, instead of creating a complete list in memory.
- Similar Syntax: It shares a similar syntax with list comprehensions, but it uses parentheses
(...)
instead of square brackets[…]
.
Now, let’s create a simple example to illustrate the concept:
# Lazy iterator using a generator expression
lazy_iterator = (x**2 for x in range(5))
# Iterate over the lazy iterator
for value in lazy_iterator:
print(value)
Lazy Iterator with Filtering:
# Lazy iterator with filtering
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
filtered_lazy_iterator = (x for x in numbers if x % 2 == 0)
# Iterate over the lazy iterator
for even_number in filtered_lazy_iterator:
print(even_number)
Here, the lazy iterator generates only the even numbers from the list numbers
based on the filtering condition.
Remember, using lazy iterators can be particularly beneficial when working with large datasets, as it allows for more efficient memory usage by generating values on-the-fly.
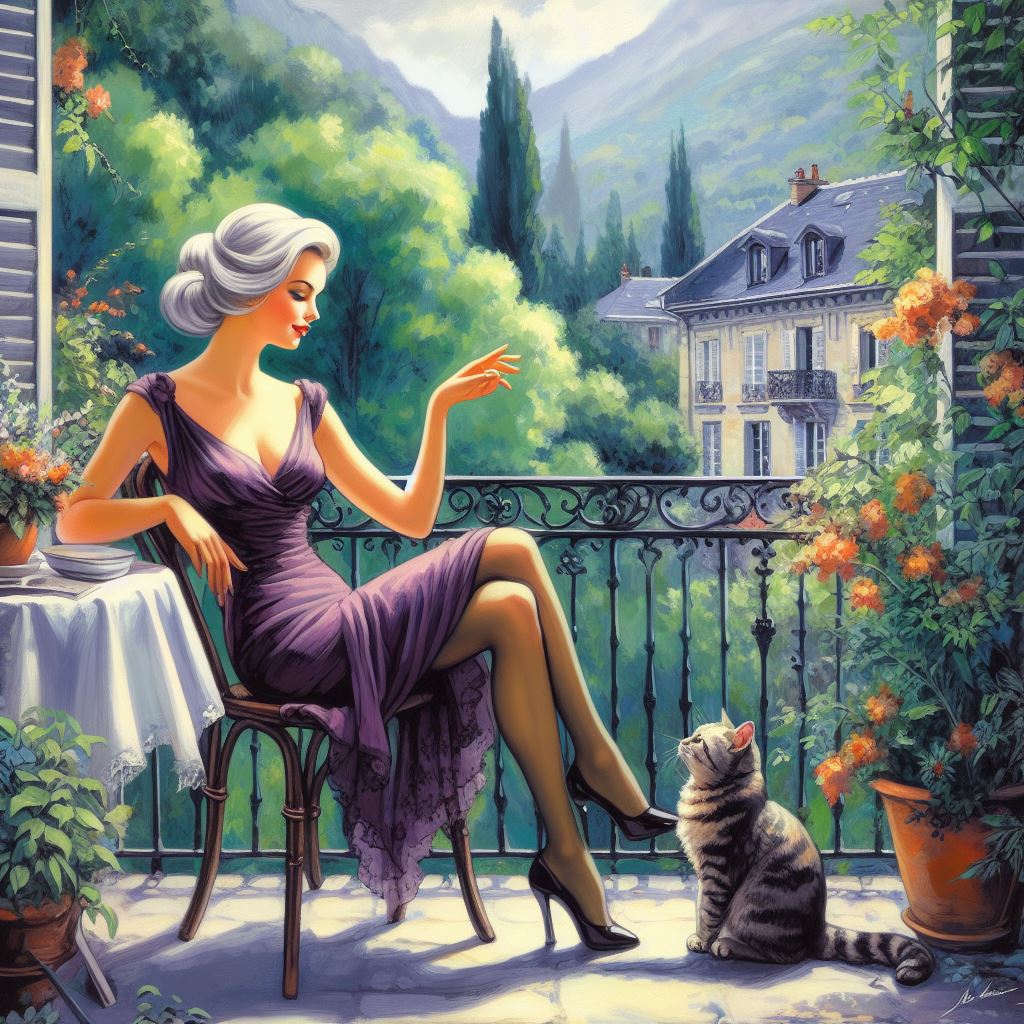
While lazy iterators (generator expressions) have their advantages, they are not always the best choice. The decision between using a lazy iterator and a list comprehension depends on the specific use case, requirements, and trade-offs. Here are some considerations:
Advantages of Lazy Iterators:
- Memory Efficiency: Lazy iterators generate values on-the-fly, which can be more memory-efficient, especially when dealing with large datasets. They don’t create a complete list in memory.
- Efficient for Streaming Data: Lazy iterators are suitable for scenarios where data is streamed or processed sequentially. They allow you to generate and process values one at a time.
- Better for Infinite Sequences: Lazy iterators are well-suited for infinite sequences or scenarios where you don’t know the size of the dataset in advance.
Advantages of List Comprehensions:
- Eager Evaluation: List comprehensions evaluate the entire expression and produce a list. This can be advantageous when you need random access to elements, as the list is already in memory.
- Performance for Small Datasets: For small datasets, list comprehensions might perform equally well or better than lazy iterators. The overhead of generating values on-the-fly might not be significant for smaller collections.
- Easier to Debug: List comprehensions can be easier to debug and inspect since they create a concrete list. You can print the list and examine its contents.
Use Cases:
- Lazy Iterators: Use them when dealing with large datasets, streaming data, or infinite sequences. They are suitable for scenarios where memory efficiency is critical.
- List Comprehensions: Use them when you need to create a complete list in memory, require random access to elements, or for smaller datasets where the overhead of lazy evaluation might not be justified.
In practice, it’s common to choose the approach that best fits the specific requirements and constraints of your application. Both lazy iterators and list comprehensions are powerful tools, and the choice depends on the nature of the problem you’re solving.
# Use Lazy Iterator (Generator Expression) for Large Dataset
lazy_iterator = (x**2 for x in range(10**6))
# Use List Comprehension for Smaller Dataset or Random Access
squares_list = [x**2 for x in range(100)]
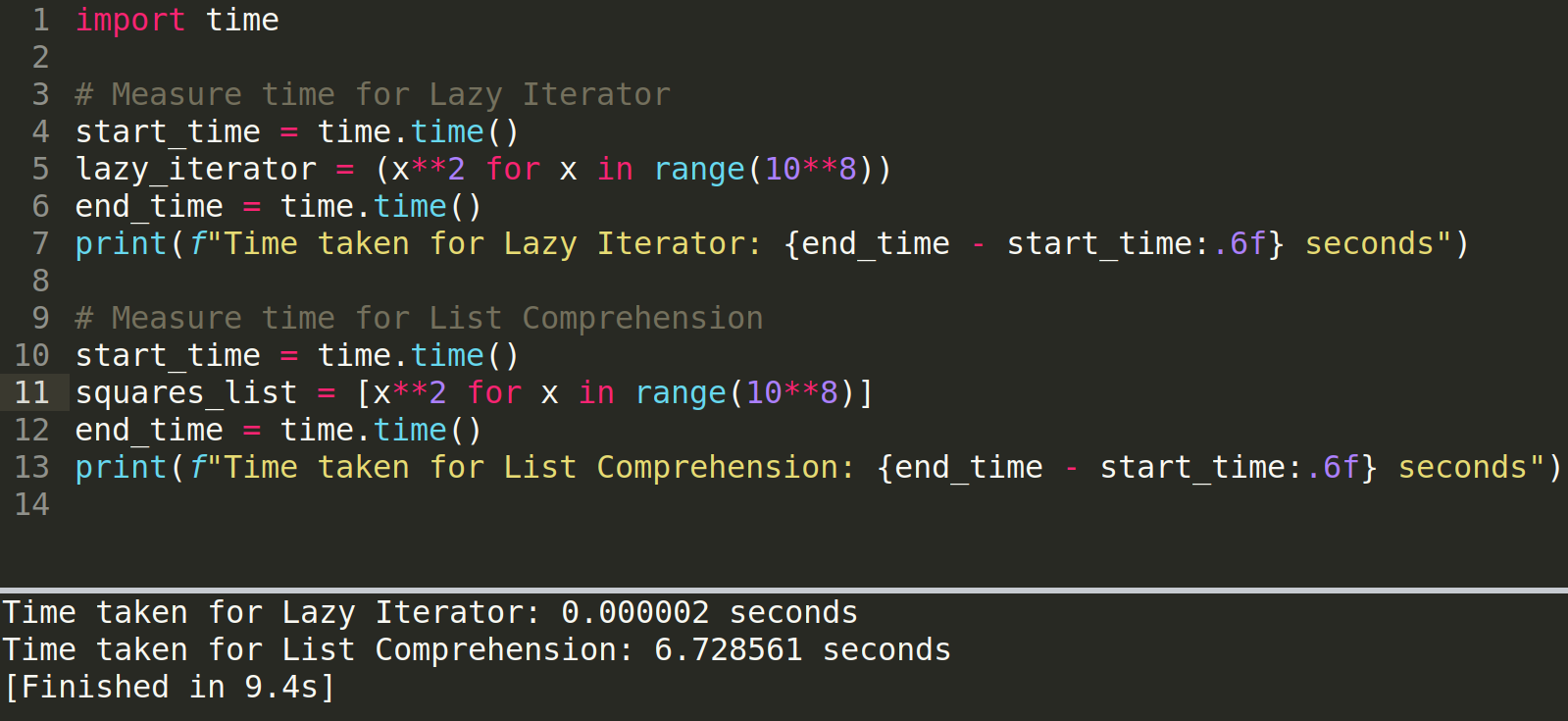