Using AI to write Unit Tests in Rust
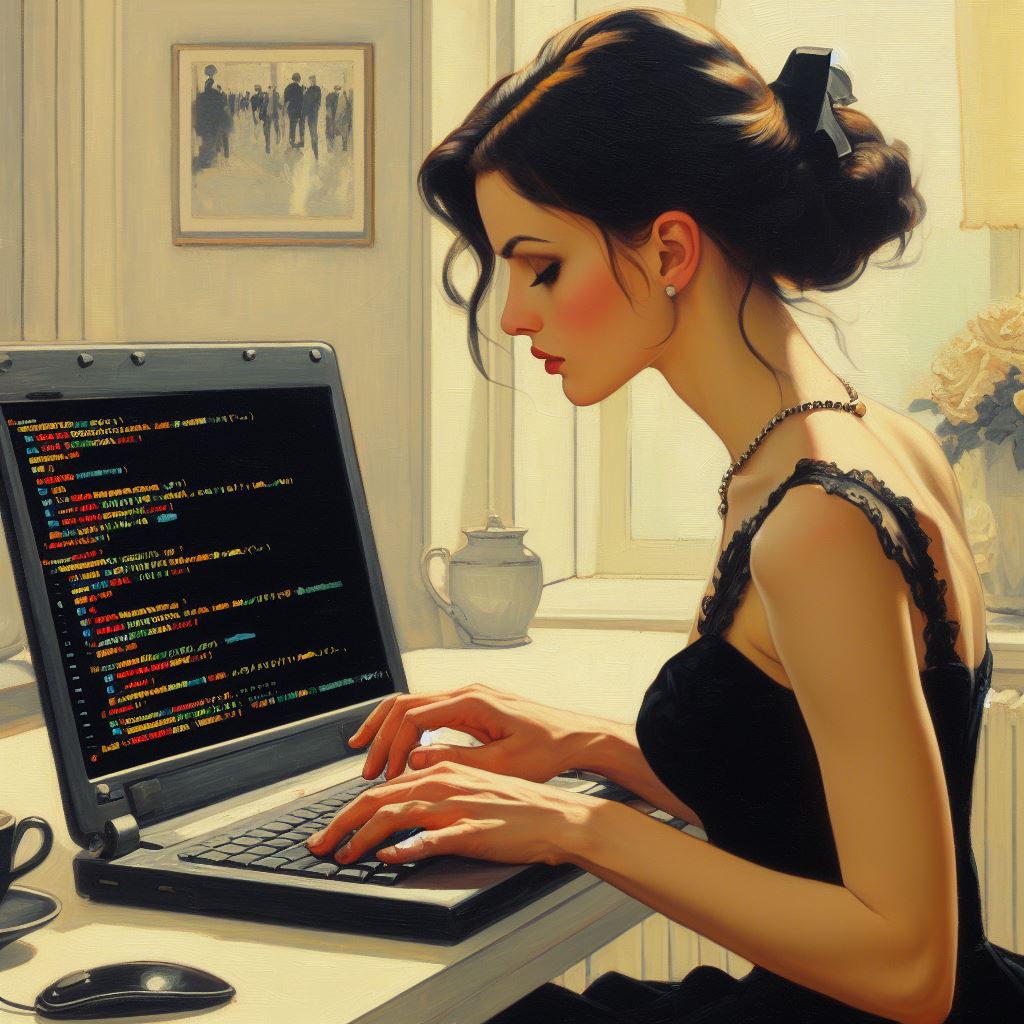
Why write unit tests?
Unit tests are essential for software development because they ensure that individual components of code work correctly. They help identify and fix bugs early, provide documentation for code behaviour, and enable safe code changes by preventing regressions. Ultimately, unit tests enhance code reliability, maintainability, and overall software quality.
Webdock – Fast Cloud VPS Linux Hosting
Can ChatGPT write unit tests as well as a human?
No, ChatGPT cannot write unit tests as well as a human. While it can generate code and understand programming concepts, it lacks the ability to comprehensively evaluate the specific requirements and design of a software component to create effective unit tests. Writing good unit tests often requires a deep understanding of the code, its intended functionality, and potential edge cases, which AI like ChatGPT currently can’t fully grasp without human guidance. (As answered by ….ChatGPT itself!).
Can ChatGPT write unit tests that I can learn from?
To be fair, it appears to have done a decent job here?
These are the tests for the code used in the “Builder” example
#[cfg(test)]
mod tests {
use super::*;
#[test]
fn test_new_pizza_builder() {
let builder = PizzaBuilder::new("medium");
assert_eq!(builder.size, "medium");
assert!(!builder.cheese);
assert!(!builder.pepperoni);
assert!(!builder.mushrooms);
}
#[test]
fn test_add_cheese() {
let mut builder = PizzaBuilder::new("small");
builder.add_cheese();
assert!(builder.cheese);
}
#[test]
fn test_add_pepperoni() {
let mut builder = PizzaBuilder::new("large");
builder.add_pepperoni();
assert!(builder.pepperoni);
}
#[test]
fn test_add_mushrooms() {
let mut builder = PizzaBuilder::new("medium");
builder.add_mushrooms();
assert!(builder.mushrooms);
}
#[test]
fn test_build_pizza() {
let pizza = PizzaBuilder::new("large")
.add_cheese()
.add_pepperoni()
.build();
assert_eq!(pizza.size, "large");
assert!(pizza.cheese);
assert!(pizza.pepperoni);
assert!(!pizza.mushrooms);
}
}
- We create a test module using
#[cfg(test)]
and import the necessary items from the main module usinguse super::*;
. - We write individual tests for each function, such as
test_new_pizza_builder
,test_add_cheese
,test_add_pepperoni
, andtest_add_mushrooms
. In these tests, we create instances ofPizzaBuilder
, call the relevant methods, and use assertions to check the expected behavior. - We also test the
build
function in thetest_build_pizza
test, where we create a completePizza
object and verify its attributes.
You can run the tests using the cargo test
command in your Rust project, and it will execute these test functions and report the results.
Do tests increase the size of the compiled binary file?
When you build your Rust project using cargo build
, it compiles the main code and the unit tests into separate artifacts. The tests are compiled into a test harness binary that is used to run the tests. This binary is separate from your application’s main binary.
So, in most cases, unit tests do not directly add to the binary size of your application. The compiled test code is kept separate and is only used during testing.