Automate Docx creation with Rust
Using Docx-rs we can write some code and compile it to allow us to create Word document (Docx) files using text and images that the user is able to specify either via command line or via a script (e.g Python).
How does it work?
Using compiled Rust code, and a Rust crate called docx-rs you can build a binary executable file (“exe” in windows) that you can call from the command line or run via a (Python/bash/etc) script which you can use to pass the input parameters, eg the paragraph text, the headline, and the filename. If you are new to Rust you will need to install “rustup” as per these steps.
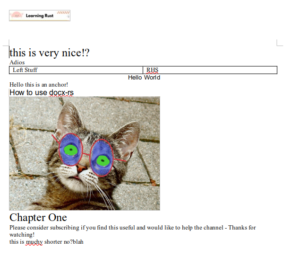
Catdocx allows you to create Word/Docx files without needing “Word”
You can script it or run via terminal (powershell)
Compile the source code using “cargo build” – you will need to install rustup to do this**
The video shows the full code and usage:
If you want to skip ahead, here is the code : https://github.com/RGGH/dcx
So some of my subscribers to my Python360 channel have in the past asked about having headers on alternate pages, and how easy it is to do hyperlinks using some of the Python modules that create Docx (Word Documents).
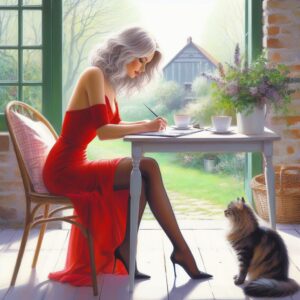
Create Word Docx files automatically
Introduction
I wanted to replicate the code I did with the Python projects in Rust. Docx-rs had some very nice examples so I was able to build up a document based on 6 or 7 of the examples and then add Clap to parse the user input.
So before we look at the Rust code, here’s the Python code that you could use once you’ve compiled “Catdocx”
import subprocess
# Define the arguments
args = [
"cargo", "run", "--",
"-t", "moo.txt",
"-l", "this is my headline nice eh?"
]
# Call the Rust binary using subprocess.run()
result = subprocess.run(args, capture_output=True, text=True)
# Print the output
print("stdout:")
print(result.stdout)
print("stderr:")
print(result.stderr)
# Check if the command was successful
if result.returncode == 0:
print("Success!")
else:
print("Error:", result.returncode)
The Design
I chose to handle the options in main.rs, but apart from that, most of the code is in controller.rs
You can see that I take the headline and paragraph text from the user, the filename can be specified as well, otherwise it will overwrite itself each time you run it.
The paragraph text is read from a plaintext file that you supply.
To customize this you can make your own file called cat.jpg
(it doesn’t have to be of a cat, just make sure it has that file name!)
You can also edit the banner jpg file or supply your own.
This is a starting point for what is essentially the old “Mail Merge” from the old days, except with Rust this could be run without needing M$ Office/M$ Word or whatever it’s called these days.
Improvements
One idea I have is to make it convert the docx file to a pdf as well (as an option).
Thanks for reading, please comment on the YouTube video if you like it or have any questions!
How do I compile someone else’s Rust code?
Compiling Rust code you found on the internet is pretty straightforward. Here’s a step-by-step guide:
1. Install Rust
First, you need to have Rust installed on your computer. The recommended way is to use rustup
, which is the Rust toolchain installer.
- Go to the Rust website and follow the instructions to install
rustup
. - Alternatively, you can run the following command in your terminal:
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
- Follow the on-screen instructions to complete the installation.
2. Set Up Your Project
Once Rust is installed, you can set up a new Rust project. Open a terminal and run:
cargo new my_project
This creates a new directory called my_project
with a basic Rust project structure.
3. Copy the Code
Copy the Rust code you found on the internet. If it’s a small snippet, you can replace the contents of src/main.rs
in your project directory with the copied code. If it’s a more complex project, follow the structure provided by the source.
4. Navigate to Your Project Directory
In your terminal, navigate to your project directory:
cd my_project
5. Compile the Code
To compile your Rust code, use the cargo build
command:
cargo build
This will compile your project and generate an executable file in the target/debug
directory.
6. Run the Code
To run your compiled code, use the cargo run
command:
cargo run
This will compile (if necessary) and run your project.
Example
Let’s say you found the following Rust code snippet on the internet:
fn main() {
println!("Hello, world!");
}
Here’s how you compile and run it:
- Create a new project:
cargo new my_project
cd my_project
- Replace the contents of
src/main.rs
with the snippet:
fn main() {
println!("Hello, world!");
}
- Compile and run:
cargo run
You should see:
Compiling my_project v0.1.0 (path_to_your_project)
Finished dev [unoptimized + debuginfo] target(s) in 1.0 secs
Running `target/debug/my_project`
Hello, world!
And that’s it! You’ve successfully compiled and run Rust code you found on the internet.