Passing closures into functions
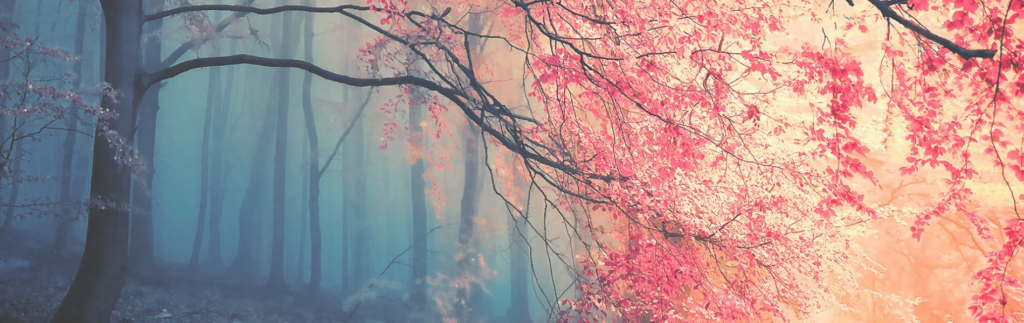
Passing closures into functions in Rust is a powerful and flexible feature that enables various use cases. Here are some common use cases for passing closures as arguments to functions:
- Custom Sorting:
You can pass a closure to functions likesort
orsort_by
to define custom sorting criteria. This allows you to sort a collection based on specific attributes or complex comparisons.
let mut numbers = vec![3, 1, 2, 4];
numbers.sort_by(|a, b| a.cmp(b)); // Sort in ascending order
- Filtering:
Functions likefilter
andfilter_map
in Rust’s iterators accept closures to filter elements from a collection based on specific conditions.
let numbers = vec![1, 2, 3, 4, 5];
let even_numbers: Vec<_> = numbers.iter().filter(|&&x| x % 2 == 0).collect();
- Mapping:
You can use closures with functions likemap
ormap_err
to transform elements in a collection.
let numbers = vec![1, 2, 3];
let squared_numbers: Vec<_> = numbers.iter().map(|&x| x * x).collect();
- Callbacks:
Closures are often used for callback functions, such as event handlers or callbacks for asynchronous operations. For example, when dealing with async I/O, you can pass closures as callbacks to handle completed tasks.
async fn fetch_data(callback: impl Fn(String)) {
let data = fetch_some_data().await;
callback(data);
}
fetch_data(|data| println!("Received data: {}", data)).await;
- Configuration:
You can use closures to pass configuration parameters to functions. For instance, a function that performs some computation might accept a closure that configures certain aspects of the computation.
fn compute_with_config(config: impl FnOnce(&mut ComputationConfig)) {
let mut computation_config = ComputationConfig::default();
config(&mut computation_config);
// Perform computation using the configured parameters
}
compute_with_config(|config| {
config.set_option(true);
config.set_precision(5);
});
- Memoization:
Closures can be used to implement memoization (caching) of expensive function results. You can pass a closure that calculates a value, and the function can cache the result for subsequent calls.
fn memoize<F, R>(func: F) -> impl FnMut(i32) -> R
where
F: Fn(i32) -> R,
R: Clone,
{
let mut cache = std::collections::HashMap::new();
move |arg| match cache.entry(arg) {
std::collections::hash_map::Entry::Occupied(entry) => entry.get().clone(),
std::collections::hash_map::Entry::Vacant(entry) => {
let result = func(arg);
entry.insert(result.clone());
result
}
}
}
These are just a few examples of how passing closures as arguments can make your code more flexible and expressive in Rust. Closures enable you to encapsulate behavior and provide it as a parameter, making your functions more generic and reusable.
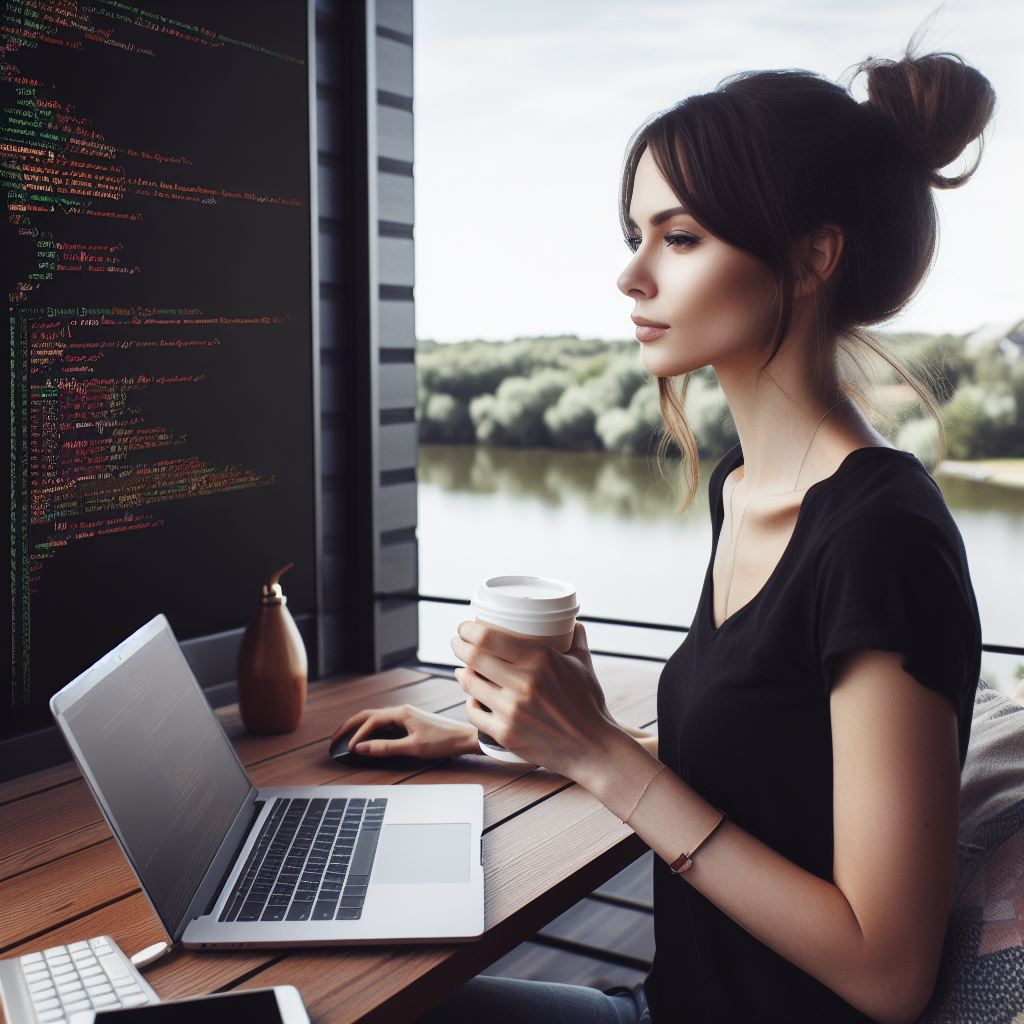
can you pass a normal function into a function?
Yes, you can pass a normal function (also called a “function pointer”) as an argument to another function in Rust. Function pointers are a way to refer to functions directly and can be used like closures. Here’s an example of passing a normal function into another function:
// Define a function that takes two i32 arguments and returns their sum
fn add(x: i32, y: i32) -> i32 {
x + y
}
// Define a function that takes a function pointer as an argument
fn calculate(operation: fn(i32, i32) -> i32, x: i32, y: i32) -> i32 {
operation(x, y)
}
fn main() {
// Pass the 'add' function as an argument to 'calculate'
let result = calculate(add, 10, 5);
println!("Result of addition: {}", result); // Output: Result of addition: 15
}
In this example:
- We define a function
add
that takes twoi32
arguments and returns their sum. - The
calculate
function takes three arguments: a function pointeroperation
that represents a function with the signaturefn(i32, i32) -> i32
, and twoi32
valuesx
andy
. Insidecalculate
, we call theoperation
function withx
andy
and return the result. - In the
main
function, we pass theadd
function as an argument tocalculate
, and it successfully callsadd
to perform addition.
So, yes, you can pass normal functions (function pointers) into other functions in Rust, just like you can with closures, which makes Rust a versatile language when it comes to handling functions as first-class citizens.
which is more idiomatic? function into a function or closure into a function?
Whether to use a function or a closure as an argument to another function in Rust depends on the specific context and requirements of your code. Both approaches are idiomatic and have their use cases:
- Function Pointers (Normal Functions):
- Use Case: Function pointers are often preferred when you have a standalone, reusable function that you want to pass around. They are more suitable for functions that have a clear and fixed signature.
- Advantages: Function pointers are more explicit and can be easier to read and understand in some cases. They are also compatible with older Rust code and libraries that may not use closures.
- Example: Passing a standard library or external crate function like
std::cmp::min
or a custom function.
fn calculate(operation: fn(i32, i32) -> i32, x: i32, y: i32) -> i32 {
operation(x, y)
}
- Closures:
- Use Case: Closures are more flexible and are a good choice when you need to define behavior on the spot, capture variables from the surrounding scope, or have complex, one-time operations.
- Advantages: Closures are often more concise and expressive, especially for short, context-specific functions. They can capture variables from their surrounding scope, making them versatile for callbacks and custom behavior.
- Example: Defining a custom sorting criteria for a particular
sort
operation.
let custom_sort = |a: &i32, b: &i32| b.cmp(a);
vec.sort_by(custom_sort);
In summary, both function pointers and closures are idiomatic in Rust, and the choice between them should be based on your specific needs. If you have a reusable, well-defined function with a fixed signature, a function pointer may be more appropriate. On the other hand, if you need a flexible, one-time behavior or want to capture variables from the surrounding scope, closures are a good choice. Ultimately, Rust’s expressiveness allows you to make the most appropriate choice for your use case.