Async – join! macro
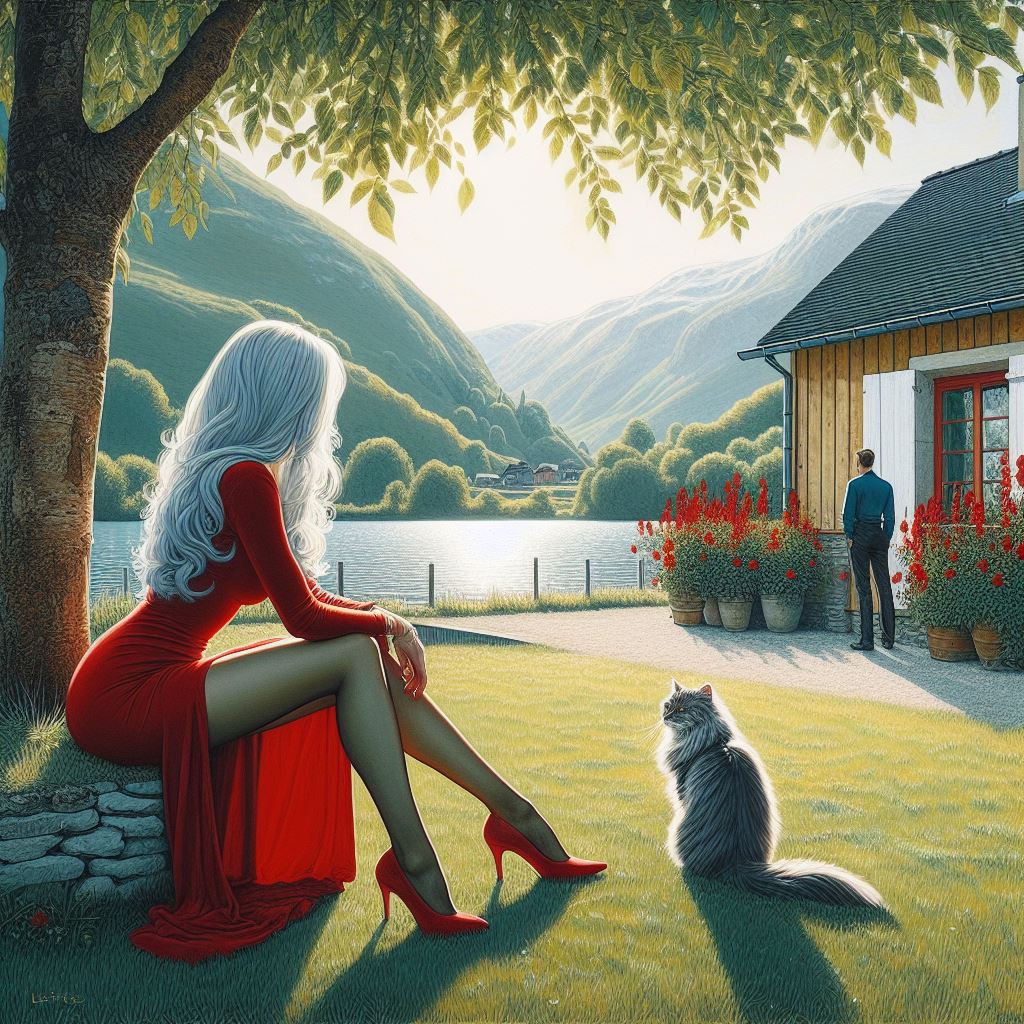
run multiple asynchronous tasks concurrently and wait for all of them to complete
The join!
macro is particularly useful when you need to run multiple asynchronous tasks concurrently and wait for all of them to complete before proceeding further. It’s commonly used in scenarios where you have independent asynchronous operations that can execute simultaneously, and you want to optimize the overall execution time by running them concurrently.
Here are some situations where you might want to use join!
:
- Independent Operations: When you have multiple asynchronous tasks that do not depend on each other’s results and can execute concurrently.
- Parallelism: When you want to take advantage of the parallelism provided by async/await in Rust. By using
join!
, you can ensure that multiple tasks are executed concurrently, potentially improving performance. - Waiting for Multiple Results: When you need to wait for the completion of multiple asynchronous tasks and collect their results before proceeding further in your program.
- Synchronization: When you want to synchronize the execution of multiple tasks and ensure that they all complete before proceeding to the next step.
In contrast, there are situations where you might not need to use join!
:
- Sequential Operations: If your asynchronous tasks need to be executed sequentially, one after the other, you don’t necessarily need
join!
. You can simply await each task one by one. - Dependent Operations: If the execution of one asynchronous task depends on the result of another, you may not be able to use
join!
directly. In such cases, you might need to chain your async/await calls to ensure proper sequencing of operations. - Error Handling:
join!
collects results from all tasks and returns them together, which can make error handling more complex, especially if you have tasks that can fail independently. In such cases, you might prefer handling errors individually as they occur.
In summary, join!
is a powerful tool for running multiple asynchronous tasks concurrently and waiting for all of them to complete. It’s especially useful in scenarios where you can take advantage of parallelism and need to synchronize the execution of independent tasks. However, it’s essential to consider the specific requirements of your program and whether join!
is the best fit for your use case.
use tokio::time::{sleep, Duration}; // Import sleep and Duration from tokio
async fn fetch_data_from_server() -> String {
// Simulate fetching data from a server by waiting for some time
println!("Fetching data from server...");
sleep(Duration::from_secs(2)).await;
"Data from server".to_string()
}
async fn process_data(data: String) {
// Simulate processing the data by waiting for some time
println!("Processing data: {}", data);
sleep(Duration::from_secs(3)).await;
println!("Data processed successfully");
}
#[tokio::main] // Use the tokio runtime for async operations
async fn main() {
// Use the join! macro to run both async functions concurrently
let fetch_task = fetch_data_from_server();
let process_task = process_data("Sample data".to_string());
// Wait for both tasks to complete
let (data_from_server, _) = tokio::join!(fetch_task, process_task);
println!("All tasks completed successfully");
}