Factorial example in Rust
Whilst it’s not the best use of recursion it’s a common tutorial and interview example. As Al Sweigart says “The Recursive Factorial Algorithm Is Terrible” ~ https://inventwithpython.com/recursion/chapter2.html#
Nevertheless, as a learning exercise, it’s a good way to get your feet wet.
One of they key concepts to understand is “stack unwinding” – which you can see a visualization of recursion here
But first, let’s look at the recursion example written in Rust – video:
Rust Factorial
fn main() {
println!("{}", factorial(5));
}
fn factorial(x: i32) -> i32 {
if x <= 1 {
return 1;
}
x * factorial(x - 1)
}
Python Tutor visualization of the recursion
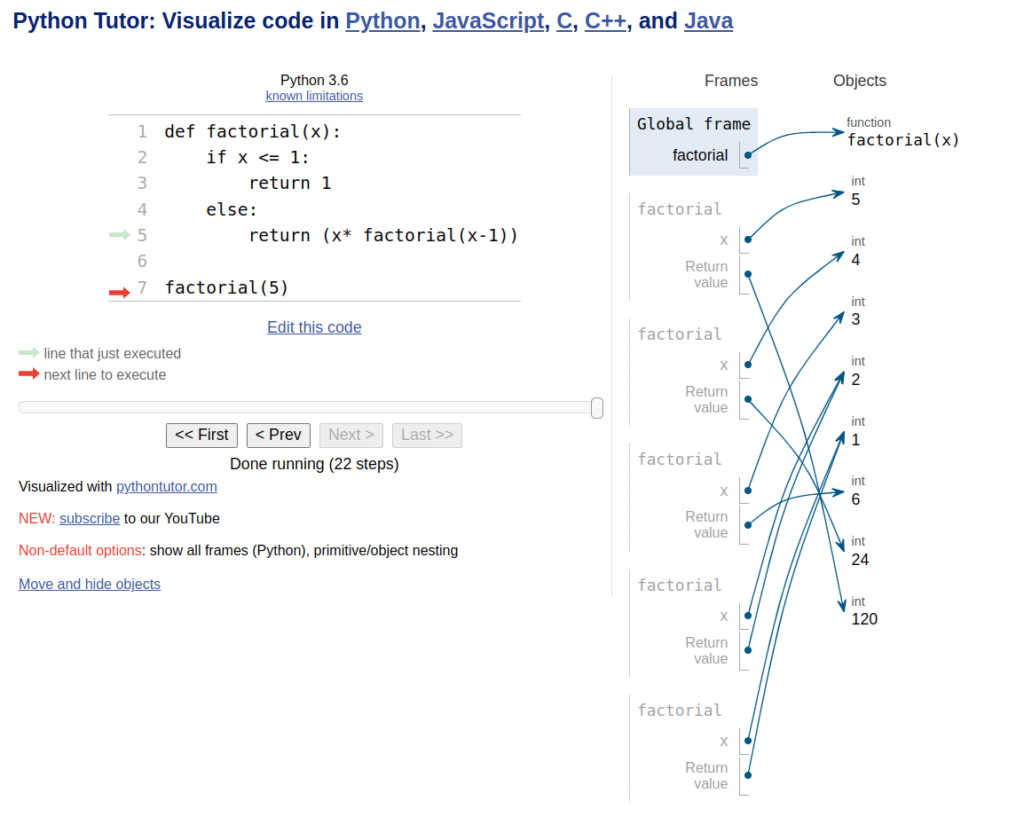
Python version of the Rust code:
def factorial(x):
if x <= 1:
return 1
return (x* factorial(x-1))
print(factorial(5))
For more on Recursion, this is a great resource : https://inventwithpython.com/recursion/