Learning Rust and improving with Codewars solutions
One of the frustrations with learning from some text books is that they set vague exercises at the end of a chapter without being explicit in the nature of the task and therefore no solution either….
For example “Write a function to convert text to ascii”
Codewars
Replace With Alphabet Position
I’ve started to use Codewars as a tool to help brush up on the “cleverest” ways to solve a problem. The term “cleverest” being based upon peer recognition.
I’ve picked this example : https://www.codewars.com/kata/546f922b54af40e1e90001da/rust
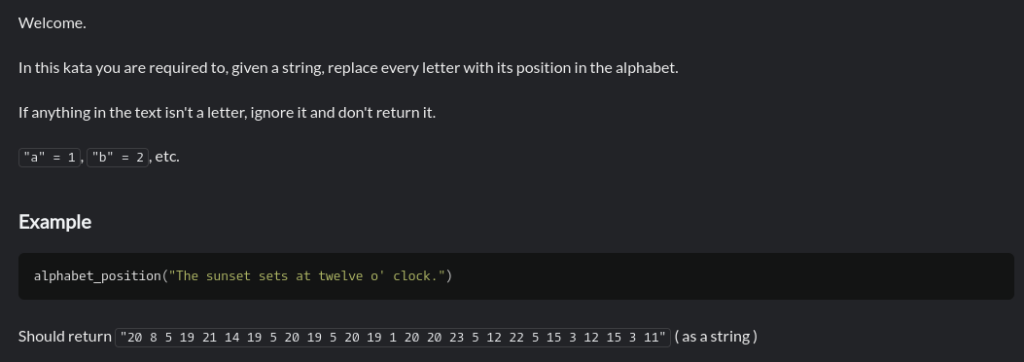
My attempt…(Which does work)
fn alphabet_position(text: &str) -> String {
let alphabet = String::from("abcdefghijklmnopqrstuvwxyz");
let mut retvec: Vec<u8> = Vec::new();
for c in text.to_ascii_lowercase().chars() {
let f = alphabet.find(c);
let res = match f {
Some(v) => v as u8 + 1,
_ => 0,
};
if res > 0 {
retvec.push(res);
};
}
let stuff_str: String = retvec
.iter()
.map(ToString::to_string)
.collect::<Vec<String>>()
.join(" ");
stuff_str
}
The ‘Cleverest’ solution:
Here is the top rated solution.
So even from first glance I can see there was no need to create the “alphabet” on line 2, I could have used filter and map with closures…and used “chars” to create an iterable rather than a for loop. Much more idiomatic as well?
Why the u32 – 96 ?
Once the char is lower case, you can subtract 96 to find it’s corresponding numeric value giving a = 1, b =2, and so on.
Check out the ASCII reference
Even with my long winded solution I worked out that to remove the quotes around each of the numbers you can use
".join(" ")
fn alphabet_position(text: &str) -> String {
text.to_lowercase()
.chars()
.filter(|c| c >= &'a' && c <= &'z')
.map(|c| (c as u32 - 96).to_string())
.collect::<Vec<String>>()
.join(" ")
}
Check out the code in the Rust Playground here
After solving this I was able to learn by checking the ‘best’ solution, and I’m going to try the next one which arguably is more difficult, especially in Rust.
The next Rust challenge – “The Supermarket Queue”
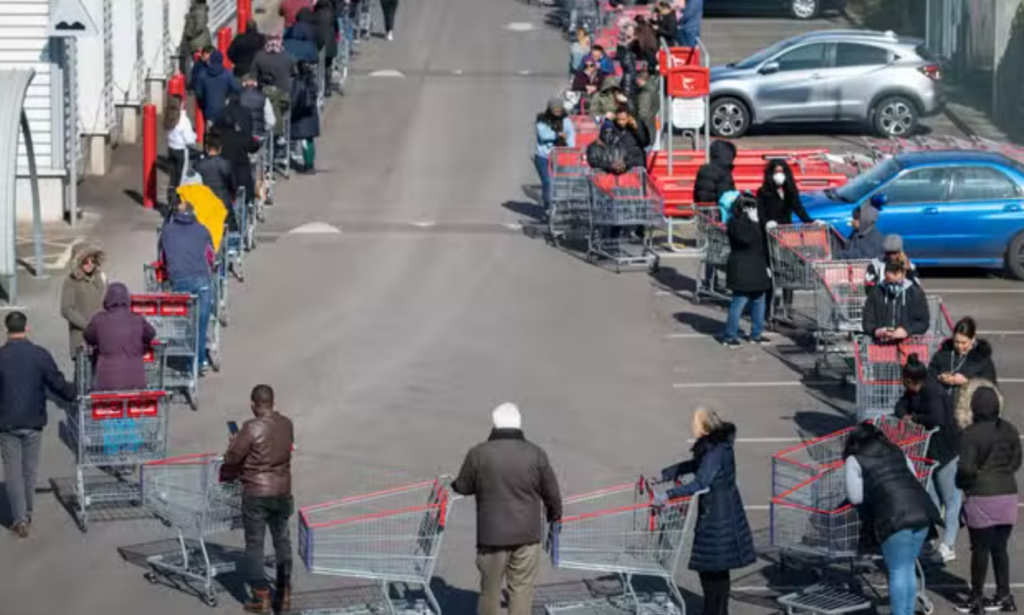
The is is the link to the “shopping queue” problem. I’ll update this post in due course, as I want to solve it before checking the solution(s).
My initial idea is to identify the 3 main scenarios, and use this code for starters :
Firstly, Scenario 1 is where there is 1 queue
Secondly, Scenario 2 is where the number of tills is the same or greater than the number of customers
Finally, Scenario 3 is where there are more customers than tills
1 and 2 are “Easy” to solve.
3 is not so straightforward and this is where the double ended queue “Deque” will help, with the use of “pop_front”
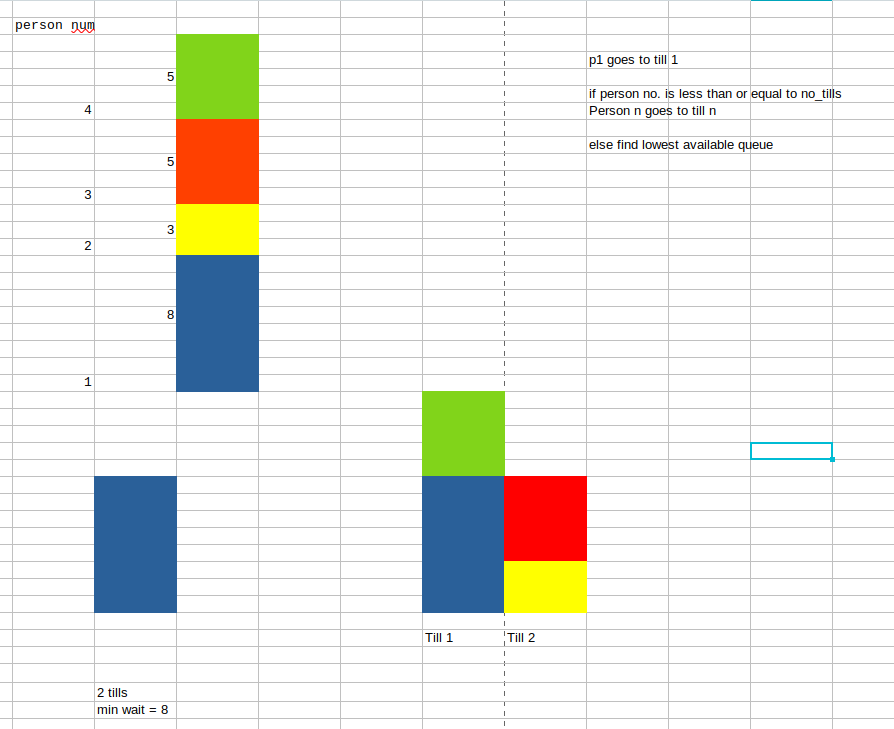
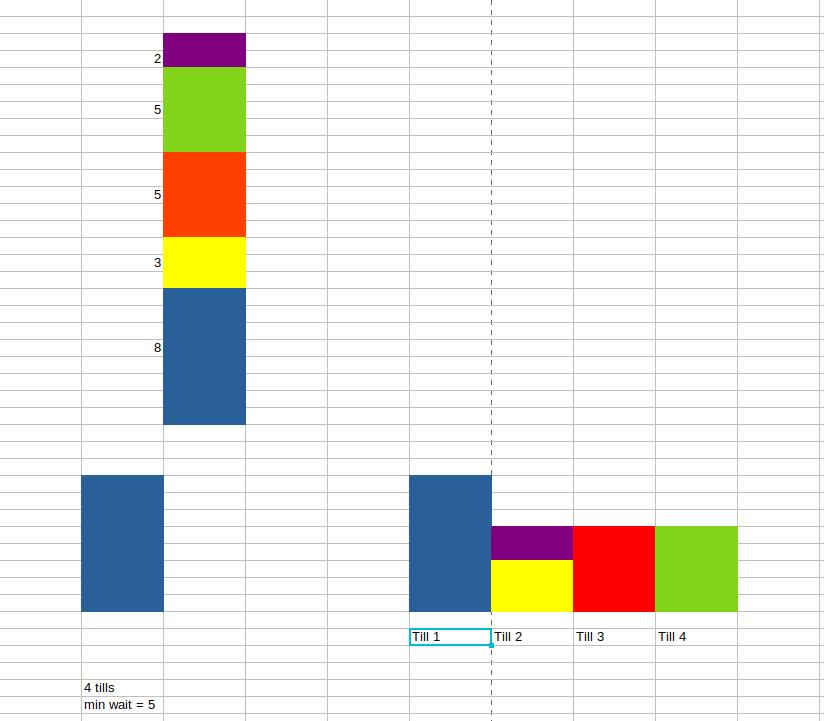
Check back soon for a working solution and a comparison against the “best” solution.
In the meantime, here’s my experimental code gist: https://gist.github.com/6d49285fa7b079ad16ea79f7305a44e5
Rust Solution = (Work-in-progress)
Python Working Solution:
VecDeque
If you want to know more about double ended queues and circular buffers, check the Rust Docs and this example:
https://doc.rust-lang.org/std/collections/struct.VecDeque.html#examples-14
Collections in Rust
If you want to know which type of collection would be suitable have a look at the Rust comparison here: