Displaying Secret Data in Binary Format (Rust Code)
In this article, we will explore how to format and display byte data in Rust. Specifically, we’ll focus on a simple program that formats a secret (a Vec<u8>
, or vector of bytes) as a binary string.
Understanding the Code
Here is the Rust code that we will break down:
use std::fmt;
struct DisplaySecret {
secret: Vec<u8>,
}
impl fmt::Display for DisplaySecret {
fn fmt(&self, f: &mut fmt::Formatter<'_>) -> fmt::Result {
for byte in &self.secret {
write!(f, "{:08b}", byte)?; // Format each byte as binary
}
Ok(())
}
}
fn main() {
let secret = DisplaySecret {
secret: vec![255, 34, 100, 9], // Example byte data
};
println!("{}", secret); // Prints: 11111111100000100011001000001001
}
1. Defining the Struct
struct DisplaySecret {
secret: Vec<u8>,
}
In this program, we define a structure DisplaySecret
with a single field secret
which is a Vec<u8>
. This field will hold the secret data we want to display. The Vec<u8>
is simply a dynamic array of bytes, and it’s a common way to represent binary data in Rust.
2. Implementing fmt::Display
for Custom Output
impl fmt::Display for DisplaySecret {
fn fmt(&self, f: &mut fmt::Formatter<'_>) -> fmt::Result {
for byte in &self.secret {
write!(f, "{:08b}", byte)?; // Format each byte as binary
}
Ok(())
}
}
In Rust, you can implement the fmt::Display
trait to control how your custom types are displayed when using println!
or format!
. Here, we implement this trait for DisplaySecret
.
- The
fmt::Display
trait allows us to define how to format the structure as a string. - The
write!(f, "{:08b}", byte)
statement inside the loop formats each byte in thesecret
vector as an 8-bit binary string ({:08b}
specifies binary format with zero-padding to ensure each byte is represented as exactly 8 bits). - The result is that each byte is printed as a string of 0s and 1s.
3. The main
Function
fn main() {
let secret = DisplaySecret {
secret: vec![255, 34, 100, 9], // Example byte data
};
println!("{}", secret); // Prints: 11111111100000100011001000001001
}
In the main
function:
- We create an instance of
DisplaySecret
, initializing it with some byte data (vec![255, 34, 100, 9]
). println!("{}", secret)
is used to display thesecret
. Since we’ve implemented thefmt::Display
trait forDisplaySecret
, this triggers thefmt
function we defined earlier.
When we run the program, the bytes are printed in their binary representation as follows:
11111111100000100011001000001001
This output corresponds to the binary representation of the bytes 255
, 34
, 100
, and 9
in sequence.
4. Conclusion
In this article, we’ve learned how to format and display byte data as binary strings in Rust by implementing the fmt::Display
trait. This allows for more readable and customized output, making it easier to understand and work with raw byte data in your programs.
Key Takeaways:
fmt::Display
is the trait we use to control how types are displayed in Rust.- We can format byte data using binary representations with
{}
and the:08b
format specifier. - Using custom formatting like this is particularly useful when working with low-level data, such as cryptographic secrets or network data.
This simple example demonstrates how flexible Rust’s formatting tools can be for presenting data in various ways.
Bonus
This shows the hex version…
{:02x}
specifies that the byte should be displayed in hexadecimal (x
), and padded with zeros if necessary to ensure two characters (02
).
use std::fmt;
struct DisplaySecret {
secret: Vec<u8>,
}
impl fmt::Display for DisplaySecret {
fn fmt(&self, f: &mut fmt::Formatter<'_>) -> fmt::Result {
for byte in &self.secret {
write!(f, "{:02x}", byte)?; // Format each byte as hexadecimal
}
Ok(())
}
}
fn main() {
let secret = DisplaySecret {
secret: vec![255, 34, 100, 9], // Example byte data
};
println!("{}", secret); // Prints: ff226409
}
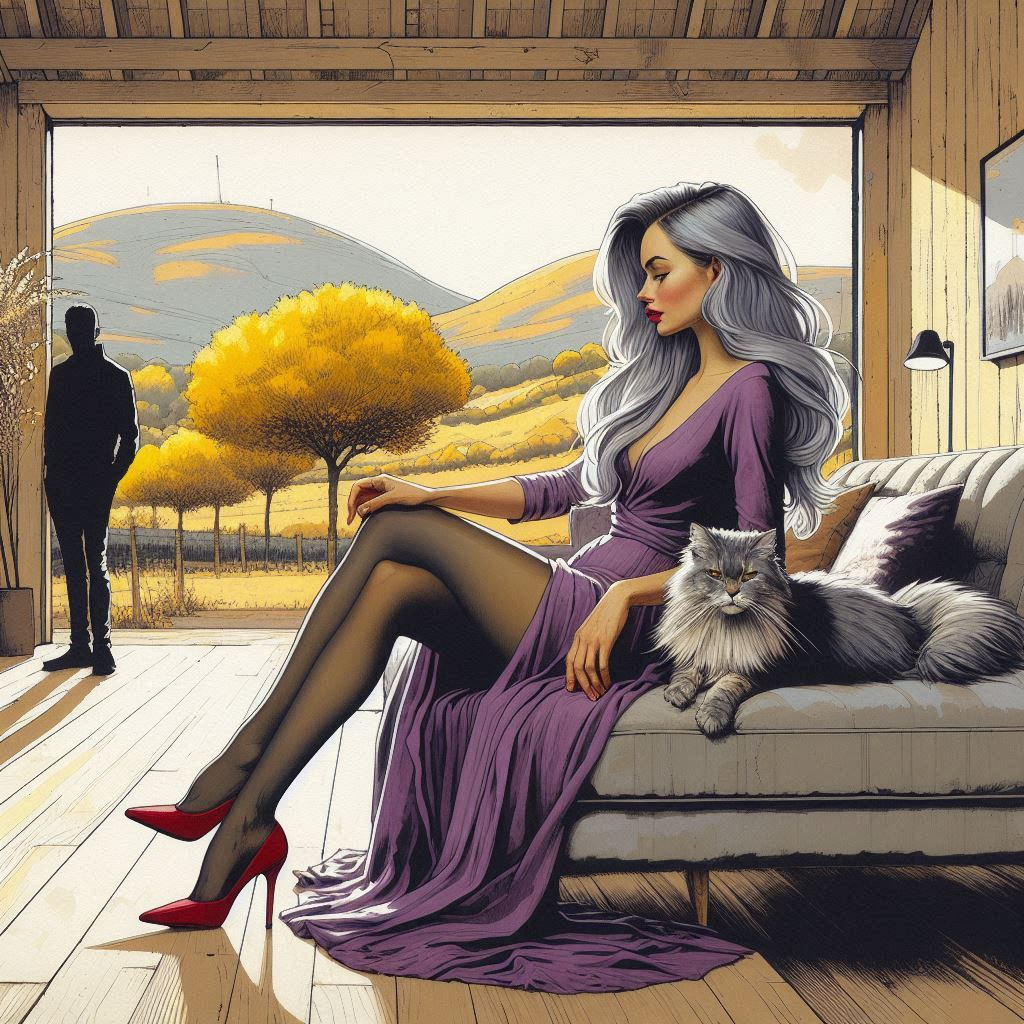
Display Data in Binary Format