Arc::Clone
Part of the YouTube series I made on concurrency in Rust
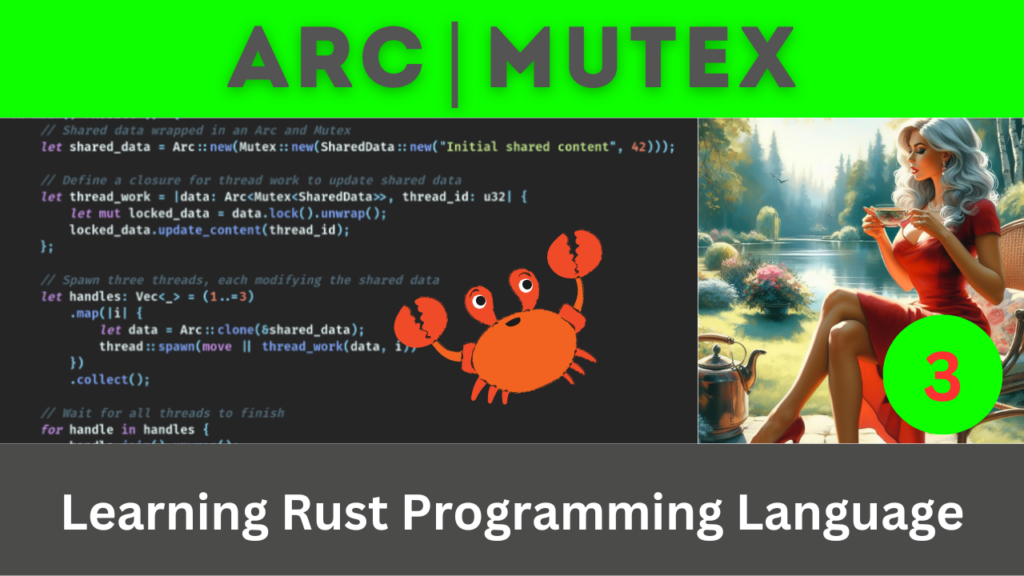
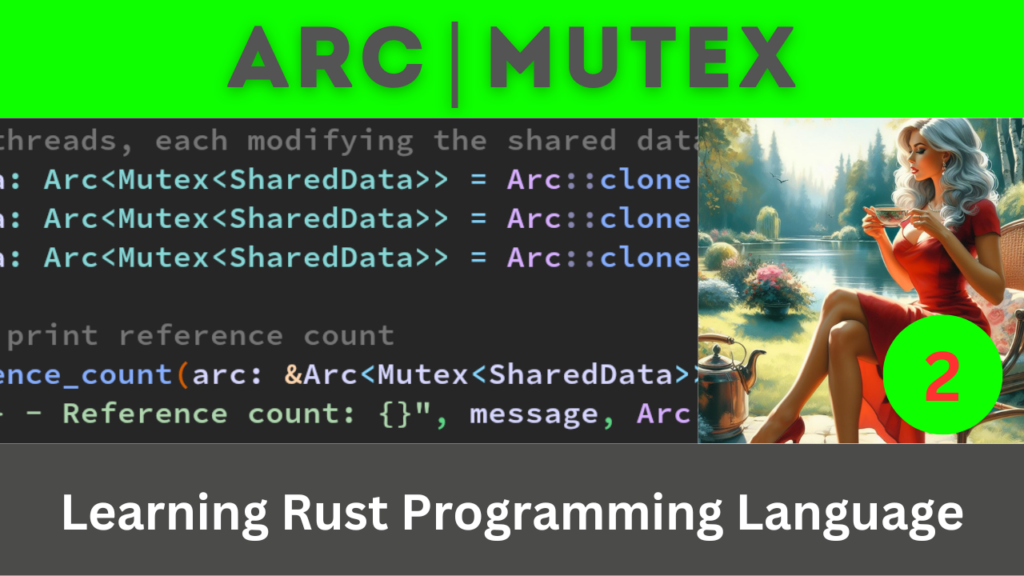
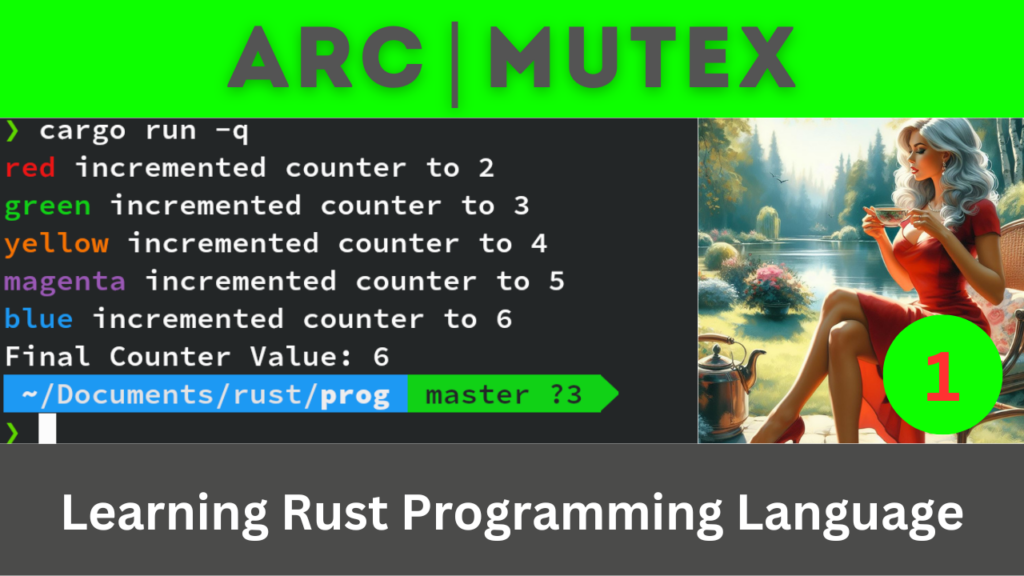
https://youtu.be/w6Incy9VBuE?si=kUP1Q1Lq69SB159A
So, why can’t the Arc::clone happen inside the thread?
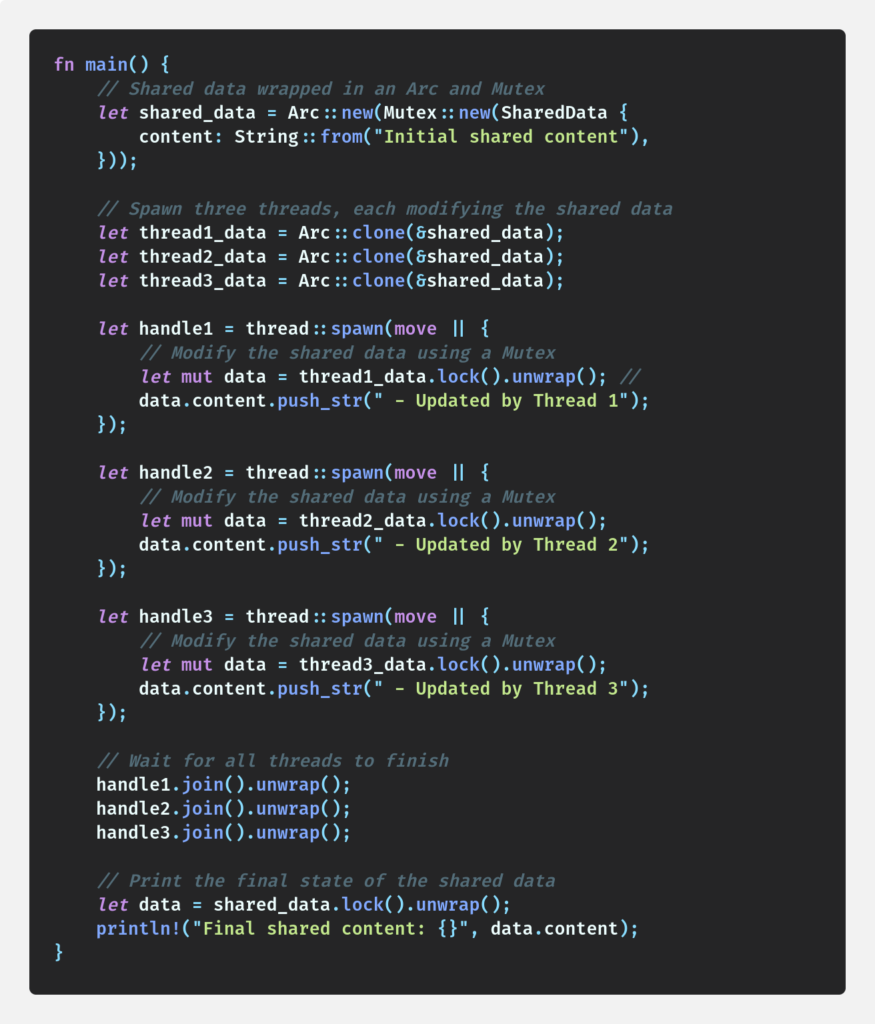
The reason you can’t perform Arc::clone
inside the thread is because Arc::clone
increases the reference count of the Arc, and doing so inside the thread would involve modifying the reference count concurrently, which can lead to data races and undefined behavior.
The purpose of using Arc
(Atomic Reference Counting) is to safely share ownership of data among multiple threads. When you clone an Arc
, it increments the reference count atomically, ensuring that multiple threads can access the shared data without causing data races.
We correctly clone the Arc
before spawning the threads:
let thread1_data = Arc::clone(&shared_data);
let thread2_data = Arc::clone(&shared_data);
let thread3_data = Arc::clone(&shared_data);
This ensures that each thread has its own reference to the shared data, and the reference count is increased safely before the threads start executing. Inside each thread, you are working with the cloned Arc
, and there is no need to perform additional cloning inside the thread itself.
In summary, the Arc::clone
should happen outside the thread to ensure proper ownership and reference counting, and each thread should work with its own cloned Arc
to safely access and modify the shared data.
Full Code
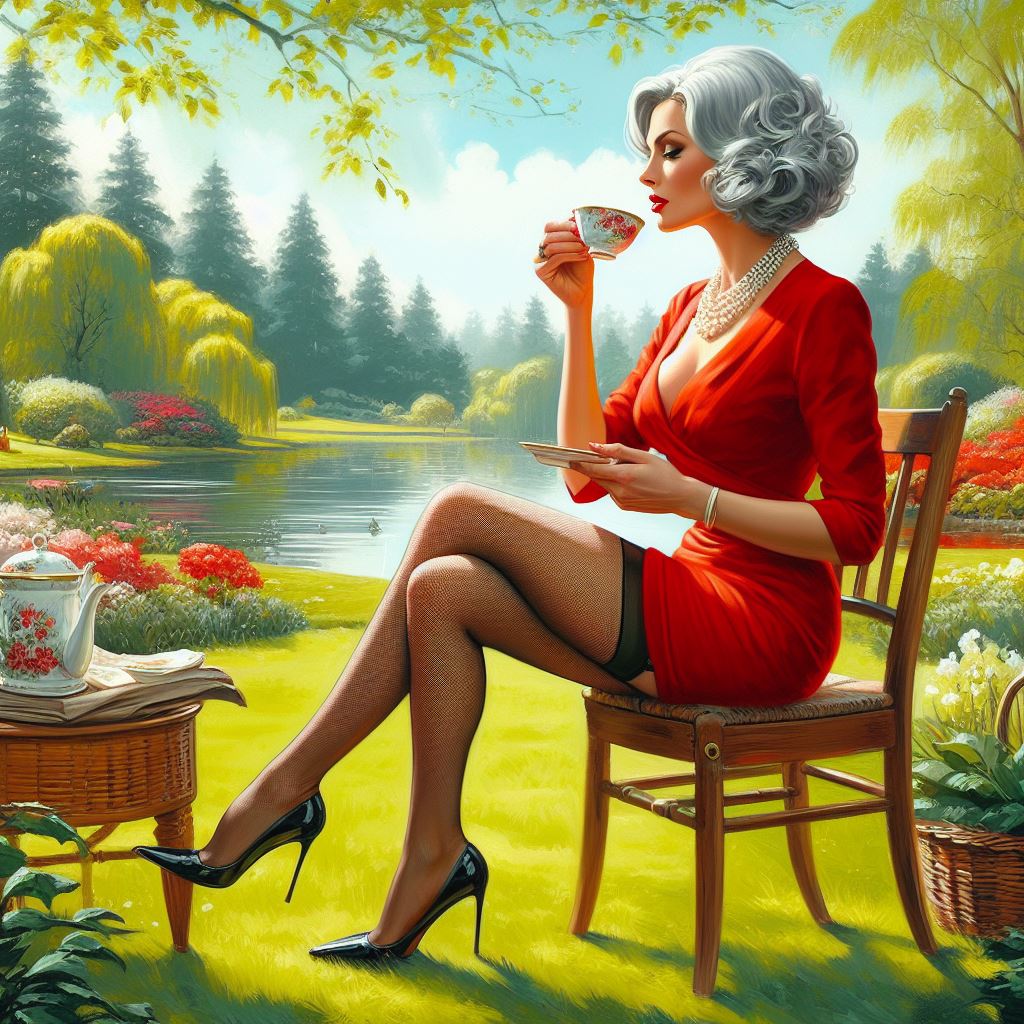
Also see the Rust docs :
https://doc.rust-lang.org/std/sync/struct.Arc.html#examples
https://doc.rust-lang.org/std/sync/struct.Arc.html#cloning-references