Rust Code Example: Serialize Data and Compute SHA-256 Hash
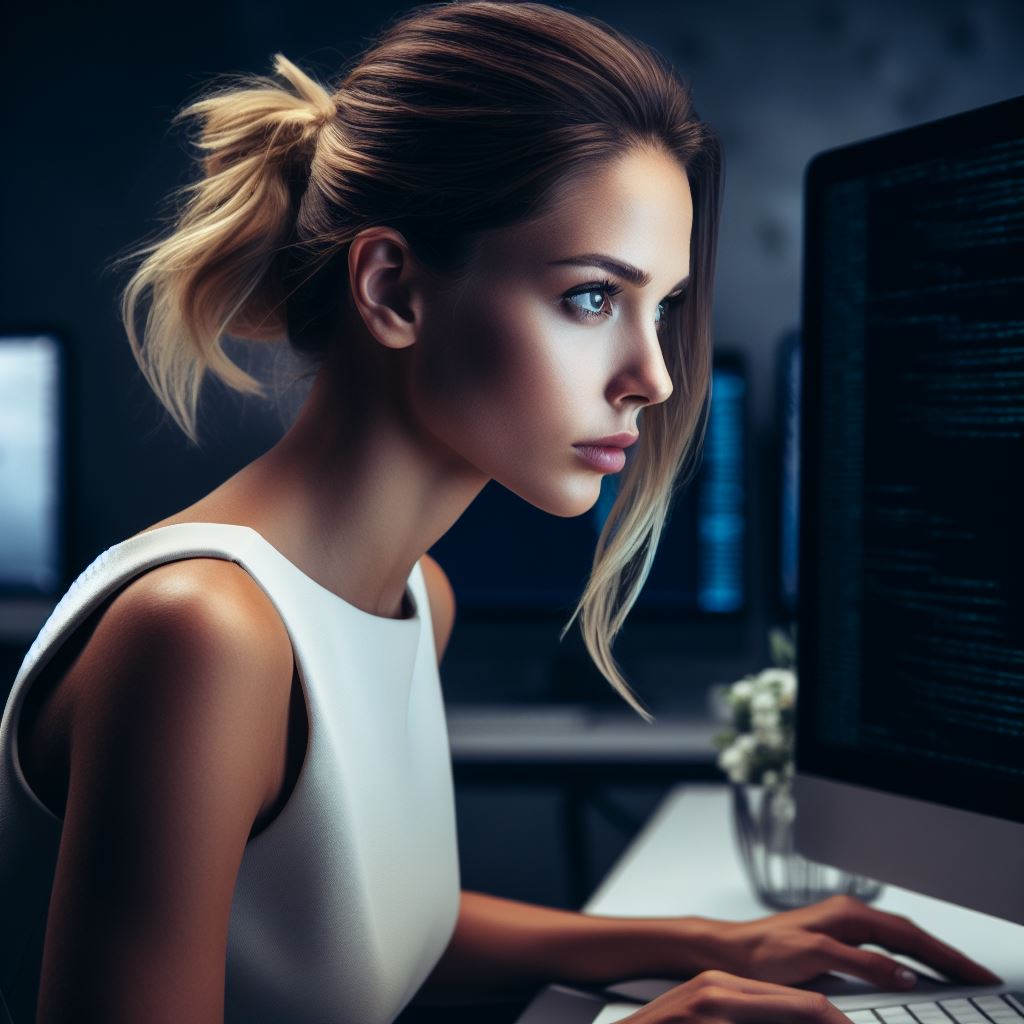
Introduction:
In this Rust code example, we’ll showcase the process of serializing data and computing a SHA-256 hash. This is a common operation when working with data in Rust, and it’s essential for tasks like data storage, security, and more. We’ll use the serde_json crate to serialize a data structure and the sha2 crate to compute the SHA-256 hash.
Webdock – Fast Cloud VPS Linux Hosting
Data Serialization:
We begin by defining a simple data structure called Bank
, which includes fields for an ID and a name. We’ll use the serde crate to serialize an instance of this structure into a JSON string. This is a fundamental operation for converting structured data into a format suitable for storage, transport, or communication.
Hashing:
We use the sha2 crate to create a Sha256 hasher, update it with the input “Meh,” and then finalize the hashing process. It’s important to note that we correctly capture the hash result in the result
variable and print it in hexadecimal format.
[dependencies]
crypto = "0.5.1"
serde = { version = "1.0.189", features = ["derive"] }
serde_json = "1.0.107"
sha2 = "0.10.8"
use serde::Serialize;
use serde_json;
use sha2::{Digest, Sha256};
#[derive(Serialize)]
struct Bank {
id: u32,
name: String,
}
fn main() {
let acc1 = Bank {
id: 111,
name: "Bob".to_string(),
};
let j = serde_json::to_string(&acc1);
println!("{:?}", j);
//hash
let mut hasher = Sha256::new();
hasher.update("Meh");
let meh = hasher.finalize();
println!("\nhash {:x}", meh);
}
Output
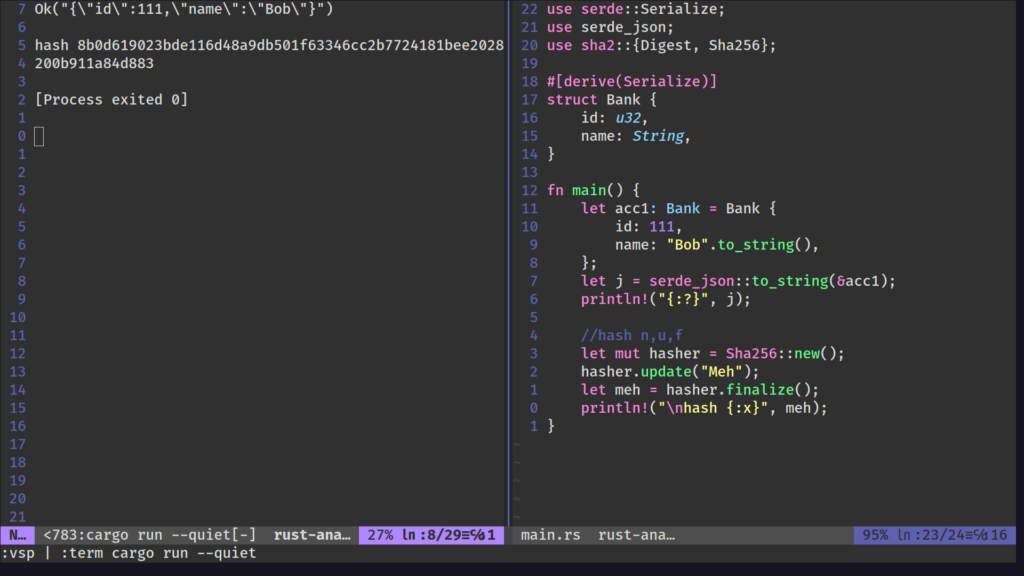
Conclusion:
This Rust code example demonstrates the essential operations of data serialization and SHA-256 hashing, which are often used in various applications, including data storage, security, and cryptography. By following this example, you can build more complex applications that involve data manipulation and cryptographic processes with Rust.