The Strategy Pattern in Rust
With the Strategy Pattern, you can:
- Define a family of algorithms.
- Encapsulate algorithms, making them easily interchangeable.
- Enhance code modularity, improving adaptability to evolving requirements.
- Write clean, efficient, and scalable Rust applications.
- Simplify complex decision-making processes in your projects.
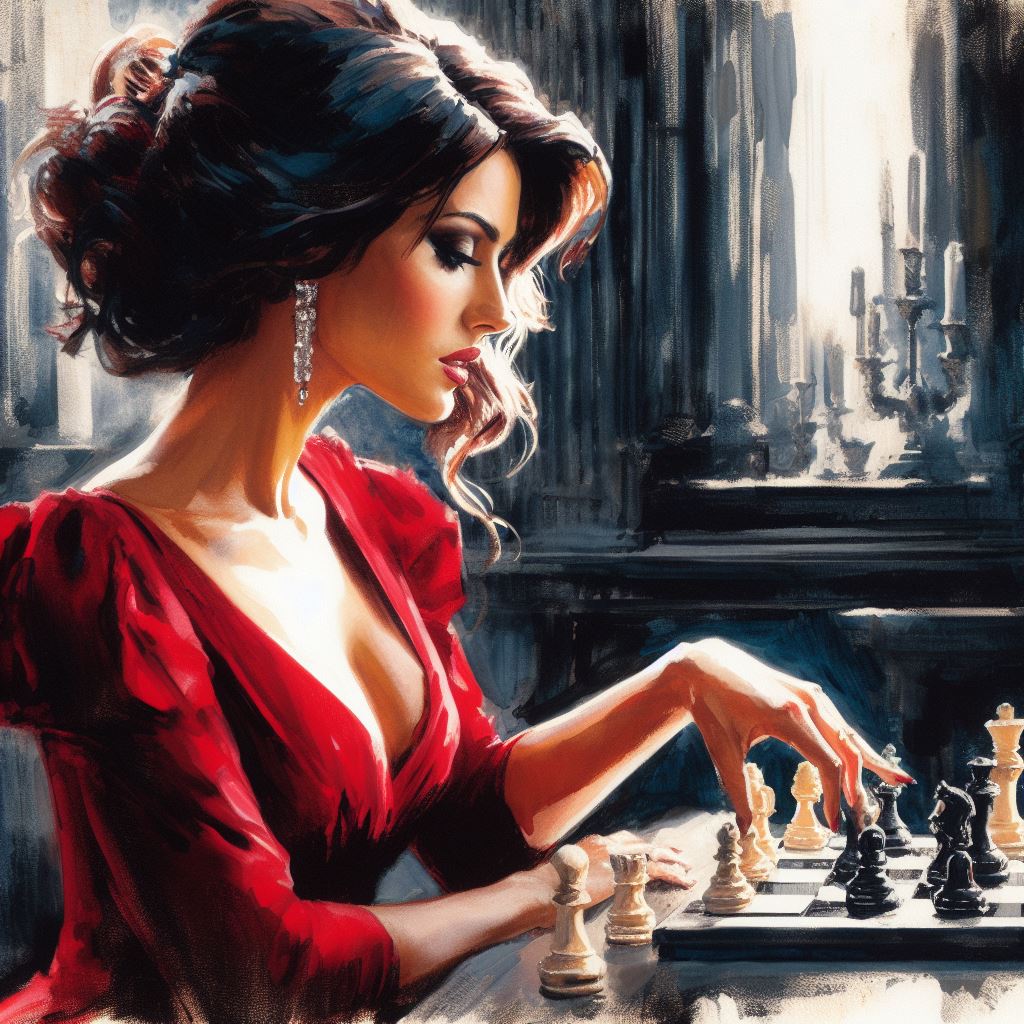
The Strategy Pattern is useful in various scenarios where you need to select and apply different algorithms or behaviors dynamically at runtime.
Webdock – Fast Cloud VPS Linux Hosting
Here are some situations in which you might need to use the Strategy Pattern:
- Algorithm Selection: When you have multiple algorithms that can be used to solve a problem, and you want to choose one of them based on specific conditions or user preferences. For example, sorting algorithms, encryption methods, or search strategies.
- Customizable Behavior: When you want to allow users or clients of your code to customize or extend its behavior without modifying the core codebase. The Strategy Pattern allows for the addition of new strategies without altering existing code.
- Testing and Mocking: In unit testing, you can use the Strategy Pattern to replace a real algorithm or service with a mock or fake implementation for testing purposes. This makes it easier to isolate and test different components of your code.
- Configuration and Tuning: When your application can have different configurations or settings that affect its behavior, and you want to switch between these configurations without changing the application’s code.
- Variations of an Interface: If you have a common interface but multiple implementations, and you want to use the appropriate implementation based on the context. This is common in libraries and frameworks where you provide a base interface but allow users to provide their own implementations.
- Plug-ins and Extensions: When you’re developing an extensible system or framework where third-party developers can create plug-ins or extensions. The Strategy Pattern allows these developers to provide custom functionality without modifying the core system.
- User Interface Design: In graphical user interface (GUI) development, you can use the Strategy Pattern to handle different input or user interaction methods, such as mouse or keyboard events.
- Optimization: In performance-critical applications, you might need to switch between different algorithms or data structures to optimize performance based on changing conditions.
In all these cases, the Strategy Pattern promotes flexibility, maintainability, and separation of concerns in your code by decoupling the context (the part of your code that uses the strategy) from the specific implementation of the strategy.
It allows for easy changes, extensions, and testing, making your code more robust and adaptable to evolving requirements.
Example code – Strategy Pattern
Consider a shipping company that needs to calculate shipping costs based on different shipping methods.
In this example, the ShippingCalculator
allows the shipping company to switch between different shipping methods (strategies) without changing the core logic.
The calculate_shipping_cost
method calculates the cost based on the currently set strategy (Standard or Express). This approach allows for flexibility and easy maintenance when dealing with various shipping strategies
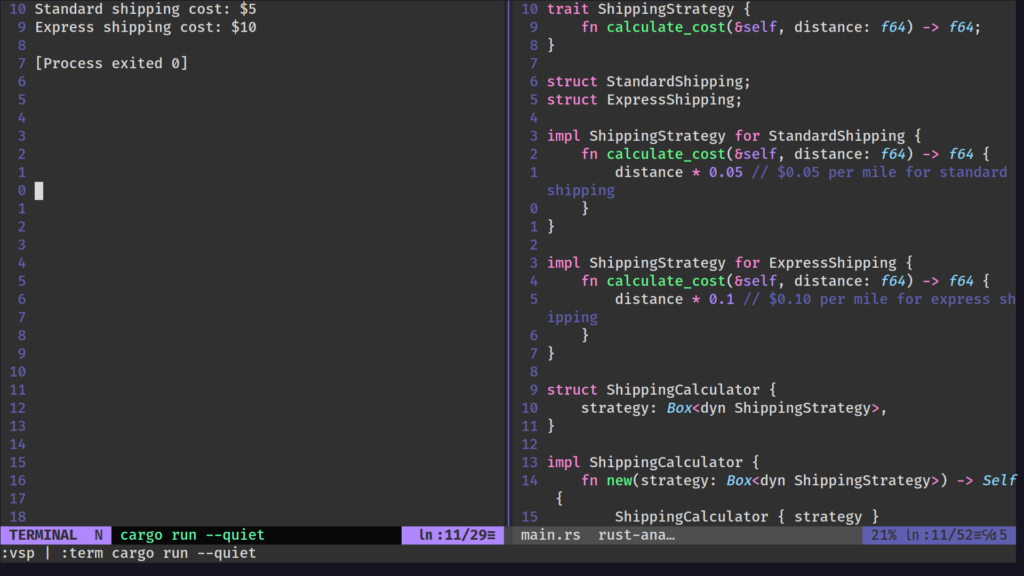
// Step 1: Define a trait for the shipping strategy
trait ShippingStrategy {
fn calculate_cost(&self, distance: f64) -> f64;
}
// Step 2: Implement concrete shipping strategies
struct StandardShipping;
struct ExpressShipping;
impl ShippingStrategy for StandardShipping {
fn calculate_cost(&self, distance: f64) -> f64 {
distance * 0.05 // $0.05 per mile for standard shipping
}
}
impl ShippingStrategy for ExpressShipping {
fn calculate_cost(&self, distance: f64) -> f64 {
distance * 0.1 // $0.10 per mile for express shipping
}
}
// Step 3: Create a shipping calculator context
struct ShippingCalculator {
strategy: Box<dyn ShippingStrategy>,
}
impl ShippingCalculator {
fn new(strategy: Box<dyn ShippingStrategy>) -> Self {
ShippingCalculator { strategy }
}
fn set_strategy(&mut self, strategy: Box<dyn ShippingStrategy>) {
self.strategy = strategy;
}
fn calculate_shipping_cost(&self, distance: f64) -> f64 {
self.strategy.calculate_cost(distance)
}
}
// Step 4: Main function to showcase the Strategy Pattern
fn main() {
let standard_shipping = Box::new(StandardShipping);
let express_shipping = Box::new(ExpressShipping);
let mut calculator = ShippingCalculator::new(standard_shipping);
let distance = 100.0; // 100 miles
let standard_cost = calculator.calculate_shipping_cost(distance);
println!("Standard shipping cost: ${}", standard_cost);
calculator.set_strategy(express_shipping);
let express_cost = calculator.calculate_shipping_cost(distance);
println!("Express shipping cost: ${}", express_cost);
}
The Rust code showcases the Strategy Pattern for calculating shipping costs. It defines standard and express shipping strategies, allowing dynamic selection. The code demonstrates how the pattern enables cost calculation flexibility by switching strategies while maintaining code simplicity.