What have I learned so far? Learning Rust in 2023
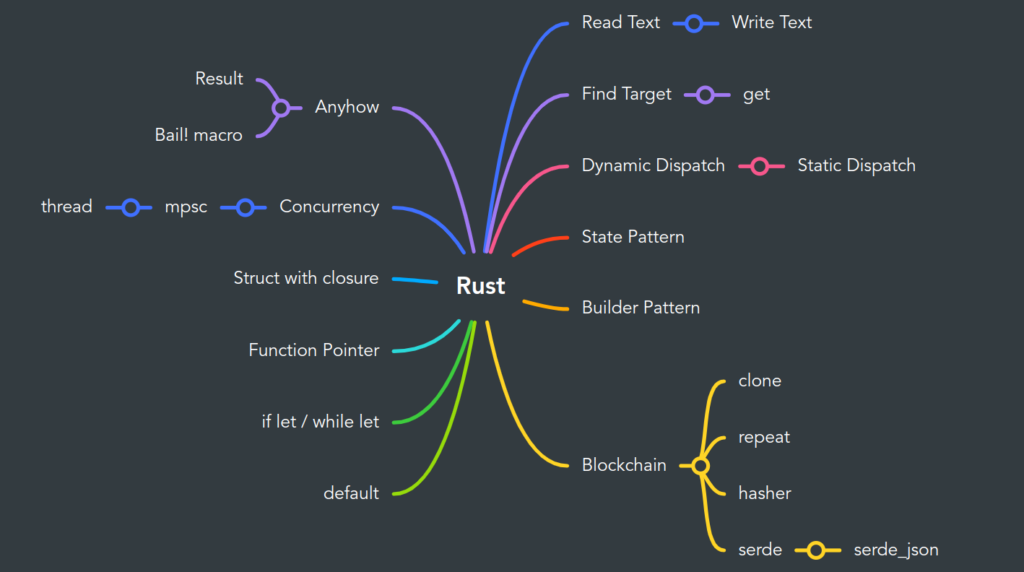
I have been learning rust in 2023 and have started to document the topics as I learn them. This also helps me us “spaced repetition” to make sure I revisit the topics as I go.
The diagram above is an aide memoire which I will update as I go along.
Webdock – Fast Cloud VPS Linux Hosting
What is spaced repetition?
Spaced repetition is a highly effective learning technique that optimizes memory retention and long-term learning. It is based on the principle that information is better remembered when it is reviewed at increasing intervals over time.
Instead of cramming all the code at once, spaced repetition involves revisiting and reviewing code at strategically spaced intervals. Initially, material is studied frequently, and as the learner demonstrates greater mastery, the intervals between reviews become longer.
This method leverages the psychological spacing effect, which indicates that our brains are more likely to remember information when it is encountered at intervals, reinforcing memory and preventing forgetting.
This technique has proven effective in improving learning outcomes and is widely used in language learning, medical education, and various other fields.
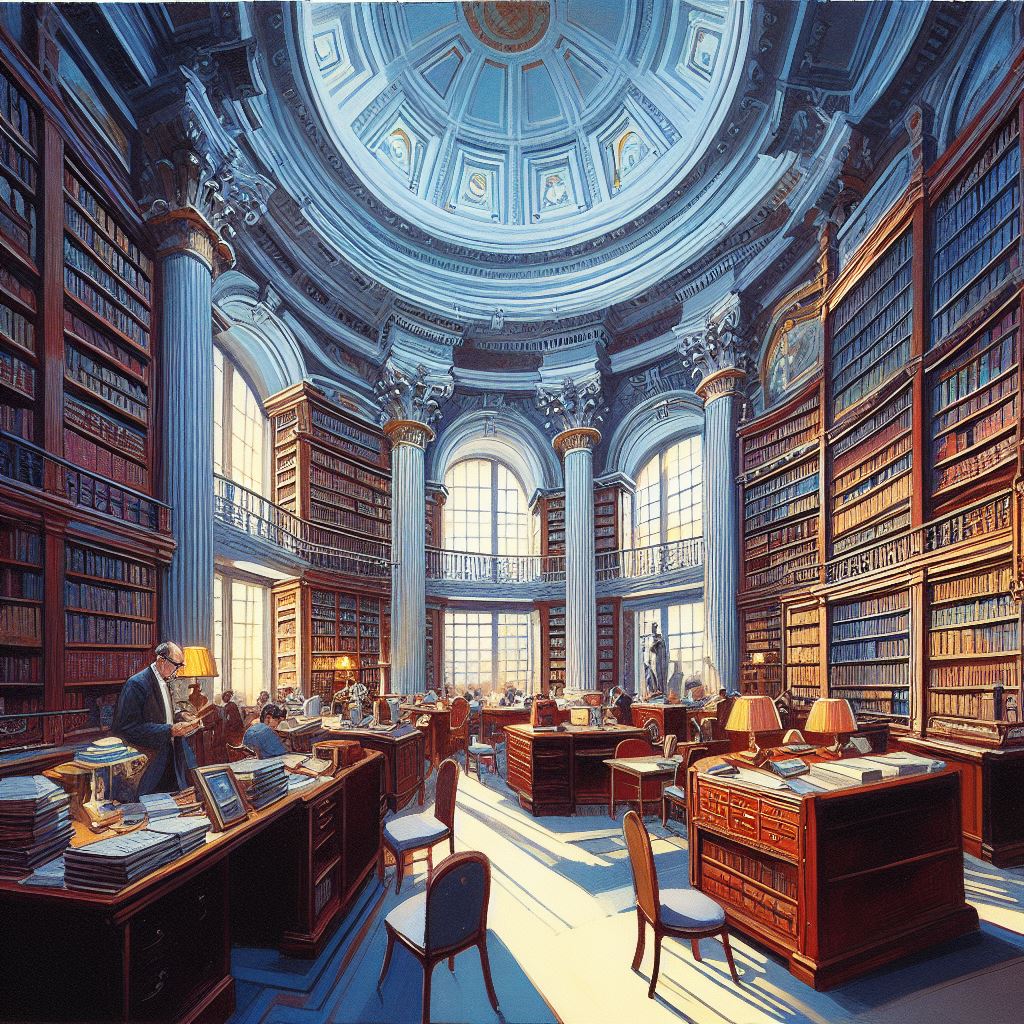
An “aide-memoire,” often spelled as “aidememoire” or “aidé-mémoire,” is a term that originates from the French language and translates to “memory aid” or “reminder.” It refers to a concise and easily accessible document, note, or mnemonic device that helps individuals recall important information, facts, or instructions.
What’s next?
I am revisiting how to create a Blockchain in Rust. This is purely for understanding as “there is no second best” and Bitcoin has been around for 14 years now and anything else is not truly decentralized without a CEO!
The example I am learning from is https://dev.to/ecj222/how-to-build-a-blockchain-from-scratch-in-rust-46
I started learning rust in 2023 and this was one of the examples that I bookmarked to come back to once I’d grasped many more of the fundamentals.
I have restructured the code slightly and am now typing in line by line and researching any parts that I don’t understand. The use of clone and mut do need plenty of thought.
Code for a Blockchain is a good refresher on using serde and serde_json, timestamp, and I’ve also come across “repeat” in the tutorial I am learning from.
fn main() {
let original_string = "Hello, ";
let repeated_string = original_string.repeat(3); // Repeat "Hello, " three times
println!("{}", repeated_string);
}
Standard Error
Compiling playground v0.0.1 (/playground)
Finished dev [unoptimized + debuginfo] target(s) in 0.40s
Running `target/debug/playground`
Standard Output
Hello, Hello, Hello,
Pick out the good bits
I like to pick out the good bits and dissect the code and make sure I know what every single nut and bolt is doing..
For example:
let mut hasher = Sha256::new();
hasher.update(serialized_block_data);
let result = hasher.finalize();
The code snippet above demonstrates how to use the SHA-256 cryptographic hash function in Rust, typically for hashing some data, like a block of data in a blockchain context. Let me break it down step by step:
let mut hasher = Sha256::new();
: This line creates a mutable variable namedhasher
and initializes it as a new SHA-256 hasher. In Rust, cryptographic hashers are part of thedigest
crate, andSha256
is a specific implementation of the SHA-256 hash function. This sets up the hasher to compute a SHA-256 hash.hasher.update(serialized_block_data);
: Here, theupdate
method of thehasher
is called with theserialized_block_data
as an argument. This method updates the hash computation with the provided data. In the context of a blockchain,serialized_block_data
likely represents the serialized content of a block, which needs to be hashed. Theupdate
method allows you to incrementally hash data, making it memory-efficient for large inputs.let result = hasher.finalize();
: Finally, thefinalize
method is called on thehasher
to compute the actual hash value. This method returns the result of the SHA-256 hashing operation and stores it in the variableresult
. Theresult
will be a fixed-size array of bytes that represents the SHA-256 hash of the serialized block data.
After these steps, result
will contain the SHA-256 hash of the serialized_block_data
, and you can use this hash for various purposes, such as verifying the integrity and uniqueness of the data in a blockchain.
Let’s see that as some standalone code:
cargo add sha2
use sha2::{Sha256, Digest};
fn main(){
// Calculate and return SHA-256 hash value.
let mut hasher = Sha256::new();
hasher.update("meh");
let result = hasher.finalize();
println!("{:?}", &result);
println!("\n{:x}", &result);
}
[246, 82, 85, 9, 77, 119, 115, 237, 141, 21
2, 23, 186, 220, 159, 192, 69, 193, 248, 15
, 220, 91, 45, 37, 23, 43, 3, 28, 230, 147,
62, 3, 154]
f65255094d7773ed8dd417badc9fc045c1f80fdc5b2
d25172b031ce6933e039a
[Process exited 0]
Described via a Metaphor
Imagine you’re making a cake. You start by getting a cake tin, which is like creating a hasher
for the SHA-256 hash. Then, you mix your cake batter, which is equivalent to the serialized_block_data
.
After that, you pour the cake batter into the cake tin, representing the use of the update
method. This action doesn’t immediately give you a cake but prepares it for baking.
Finally, you put the cake tin into the oven and bake it. This step corresponds to calling the finalize
method on the cake tin (or hasher
).
When the baking is done, you take out a fully baked cake, symbolizing the SHA-256 hash, which is a unique and fixed representation of your original mixture (or serialized_block_data
).
Just as a cake has a distinct taste and appearance after baking, the SHA-256 hash provides a unique and consistent representation of your data. eg. data verification in a blockchain.
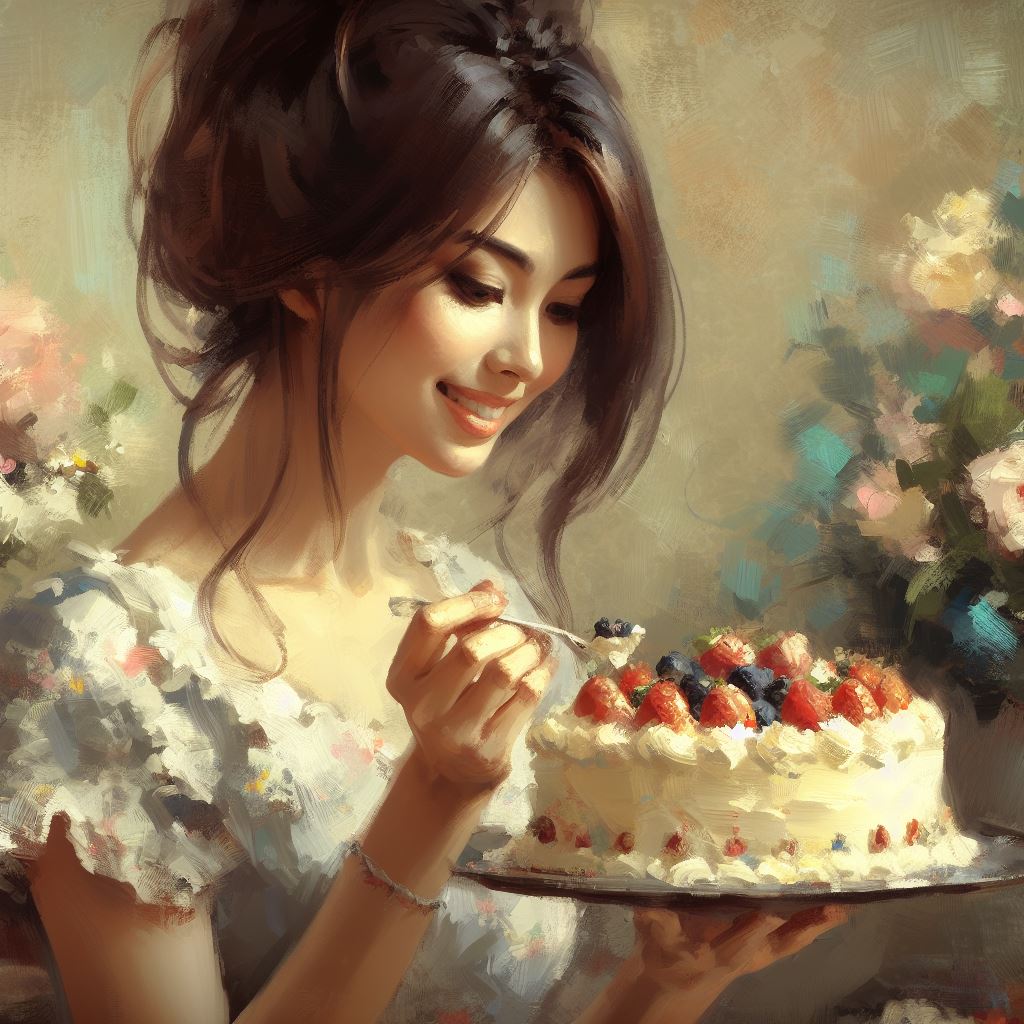
Timestamp
The timestamp is an essential part of a blockchain so well worth getting used to the various options.
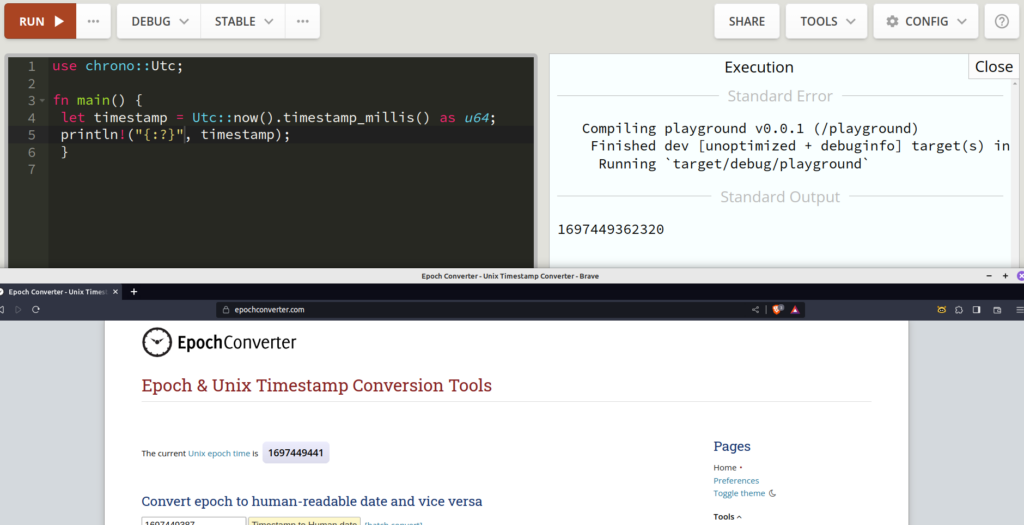
Dependencies
Knowing which dependencies to use is an additional skill, as with “KISS” principle I’ve stuck to learning from examples with fewer dependencies so far. These are the ones for the blockchain example, as see in the Cargo.toml file.
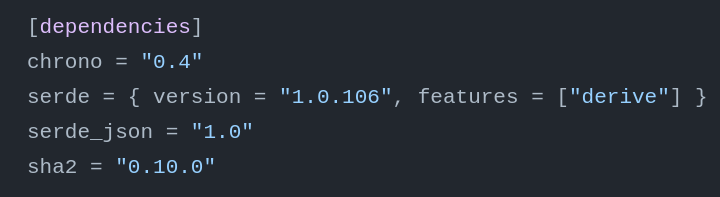
I hope you’ve found this record of my experience learning rust in 2023 useful, I’ll be adding to it so come back soon!