Rust for Python with Maturin
Integrating Rust code as a Python module allows you to leverage the performance benefits of Rust while still benefiting from Python’s versatility and ease of use. This approach is particularly useful when you need to optimize performance-critical parts of your Python application.
Let’s not duplicate the code, you can find it here:
https://www.maturin.rs/tutorial
pip install maturin
This article is just a summary of how it can be used.
So, here are the five steps for using compiled Rust code as a Python module
Write Rust Code:
- Create the performance-critical functionality in Rust using Cargo.
Expose Rust Functions to Python:
- Use the
pyo3
crate to define Rust functions callable from Python.
Compile Rust Code:
- Compile the Rust code into a shared library (
.so
) . - Use : “maturin develop” from command line
Create a Python Module:
- Write a Python module that imports the compiled Rust library and provides a Pythonic interface.
Usage:
- Import your Python module and call Rust functions like regular Python functions to leverage Rust’s performance within your Python application.
Using a module in Python that was written in Rust
Here’s the Python code being used,
The “guessing_game” module is written in Rust.
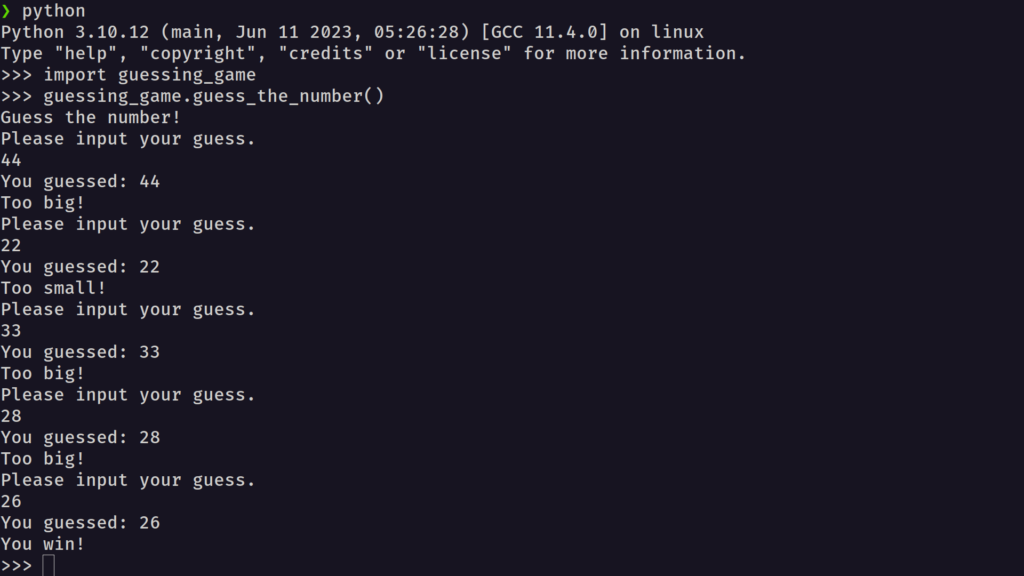
Here is the structure of the code used to build the module, the code for the actual function is added into src/lib.rs
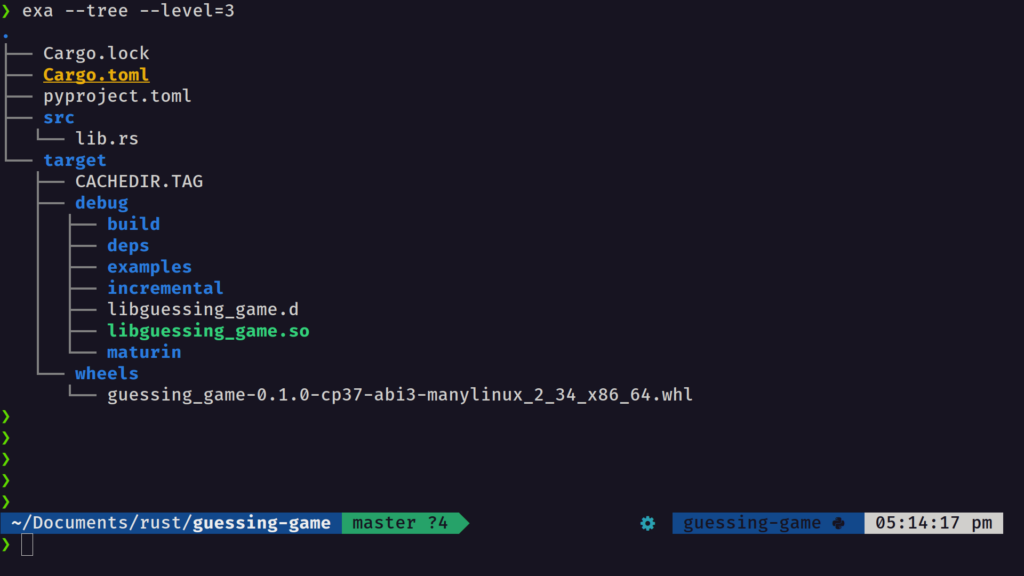
use pyo3::prelude::*;
use rand::Rng;
use std::cmp::Ordering;
use std::io;
#[pyfunction]
fn guess_the_number() {
println!("Guess the number!");
let secret_number = rand::thread_rng().gen_range(1..101);
loop {
println!("Please input your guess.");
let mut guess = String::new();
io::stdin()
.read_line(&mut guess)
.expect("Failed to read line");
let guess: u32 = match guess.trim().parse() {
Ok(num) => num,
Err(_) => continue,
};
println!("You guessed: {}", guess);
match guess.cmp(&secret_number) {
Ordering::Less => println!("Too small!"),
Ordering::Greater => println!("Too big!"),
Ordering::Equal => {
println!("You win!");
break;
}
}
}
}
/// A Python module implemented in Rust. The name of this function must match
/// the `lib.name` setting in the `Cargo.toml`, else Python will not be able to
/// import the module.
#[pymodule]
fn guessing_game(_py: Python, m: &PyModule) -> PyResult<()> {
m.add_function(wrap_pyfunction!(guess_the_number, m)?)?;
Ok(())
}
Cargo.toml
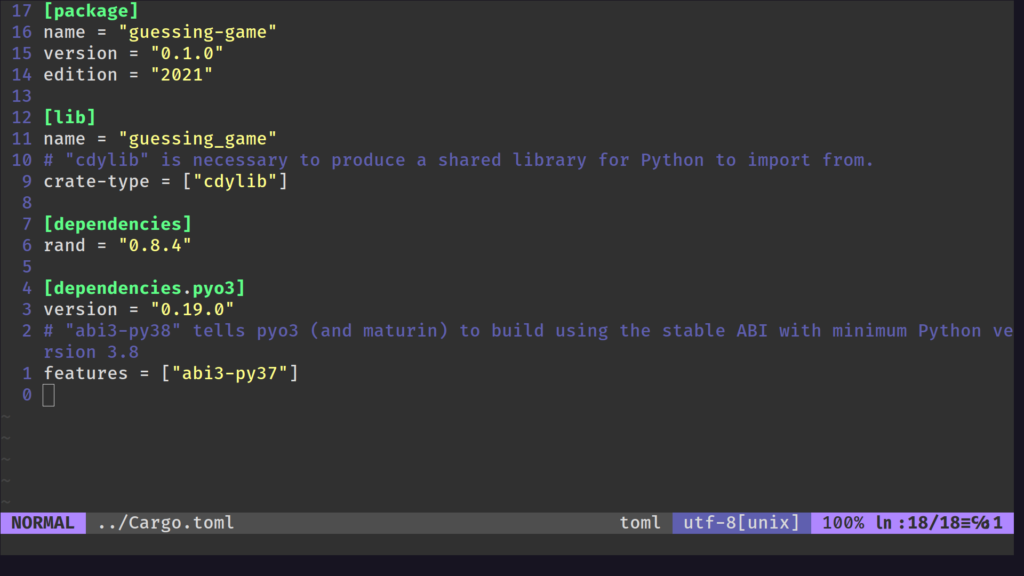
pyproject.toml
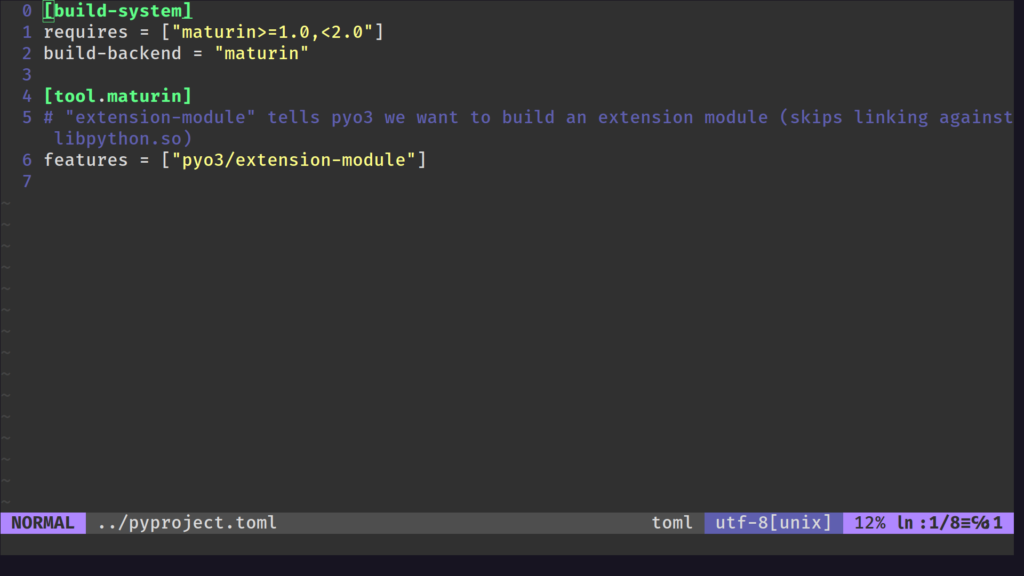
Thanks for reading
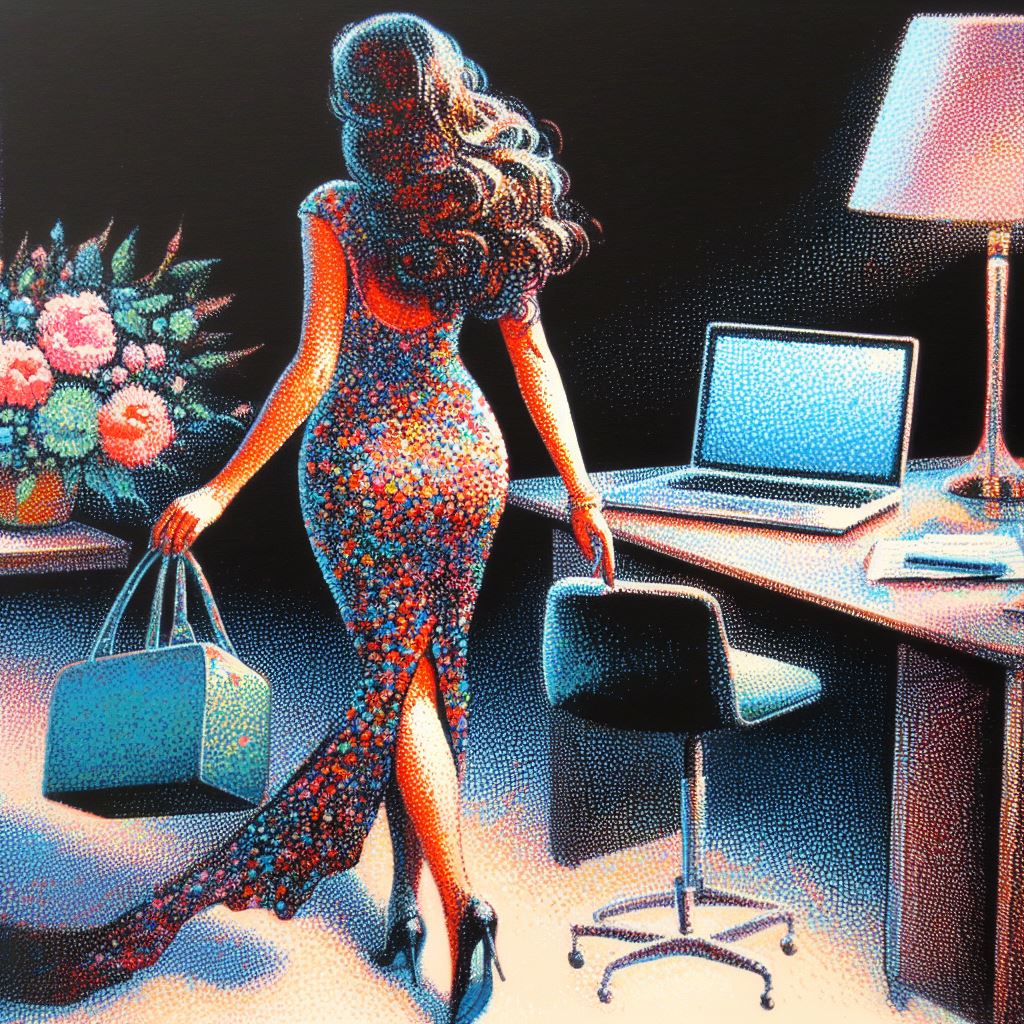