If let some match…
In Rust, you can use the if let
expression to conditionally match and destructure an enum variant or a pattern and execute code based on whether the match is successful or not.
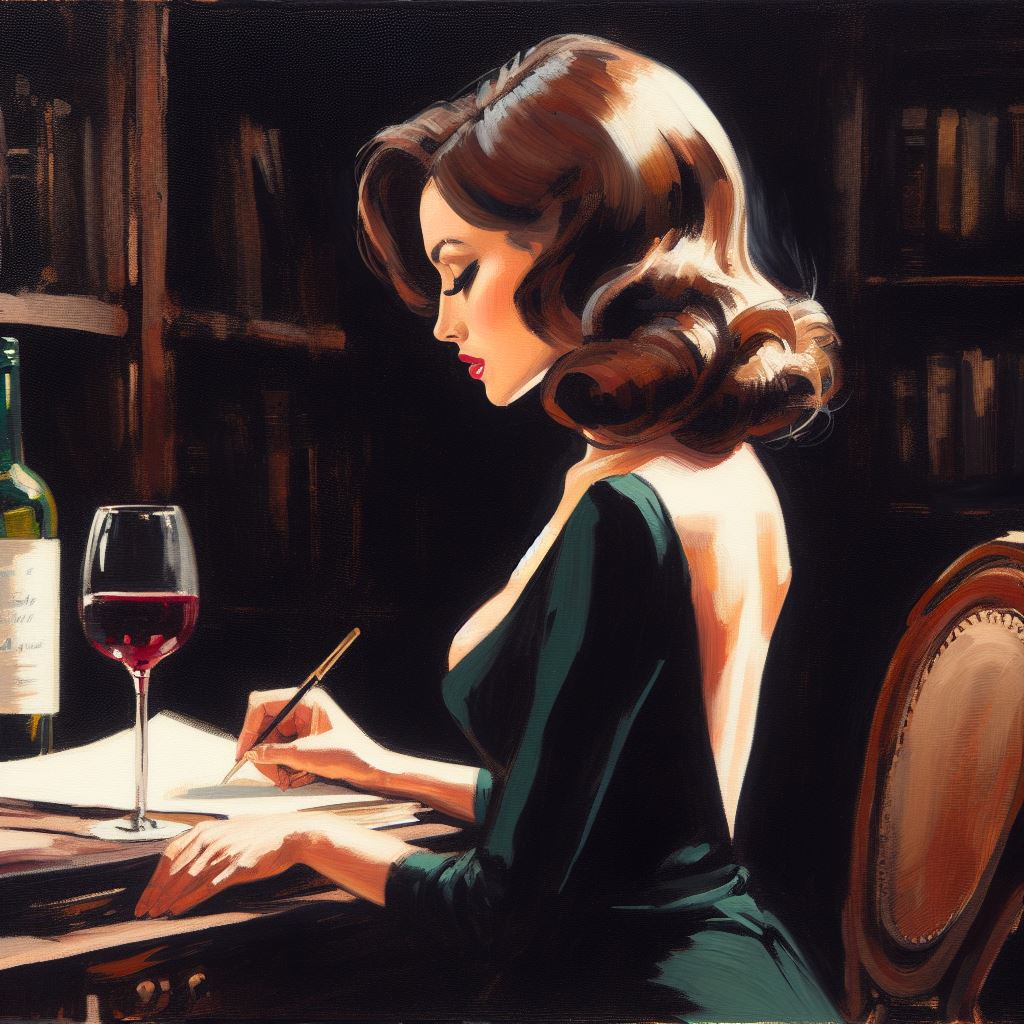
Example 1
enum SomeEnum {
SomeName(String),
NoneValue,
}
fn main() {
// Create a vector of 'SomeEnum' instances with names
let names = vec![
SomeEnum::SomeName("Alice".to_string()),
SomeEnum::NoneValue,
SomeEnum::SomeName("Bob".to_string()),
SomeEnum::SomeName("Moo".to_string()),
SomeEnum::NoneValue,
SomeEnum::NoneValue,
];
for name in names {
// Use 'if let' to check if 'name' is a 'SomeName' variant and extract the name
if let SomeEnum::SomeName(inner_name) = name {
// Use 'match' to further process the extracted name
match inner_name.as_str() {
"Alice" => println!("Found a SomeName variant with name Alice"),
"Bob" => println!("Found a SomeName variant with name Bob"),
_ => println!("Found a SomeName variant with an unknown name"),
}
} else {
println!("Did not find a SomeName variant.");
}
}
}
Example 2
enum ProductStatus {
InStock,
OutOfStock,
}
struct Product {
name: String,
price: f64,
status: ProductStatus,
}
fn main() {
// Create a list of products
let products = vec![
Product {
name: "Smartphone".to_string(),
price: 599.99,
status: ProductStatus::InStock,
},
Product {
name: "Laptop".to_string(),
price: 999.99,
status: ProductStatus::InStock,
},
Product {
name: "Tablet".to_string(),
price: 299.99,
status: ProductStatus::OutOfStock,
},
];
// Display the products and their availability
println!("Product Inventory:");
for product in &products {
if let ProductStatus::InStock = product.status {
match product.name.as_str() {
"Smartphone" => {
println!("Special Offer: {} - Price: ${:.2} - In Stock", product.name, product.price);
},
_ => {
println!("Product: {} - Price: ${:.2} - In Stock", product.name, product.price);
}
}
} else {
println!("Product: {} - Status: Out of Stock", product.name);
}
}
}