How to implement a method for an enum in Rust
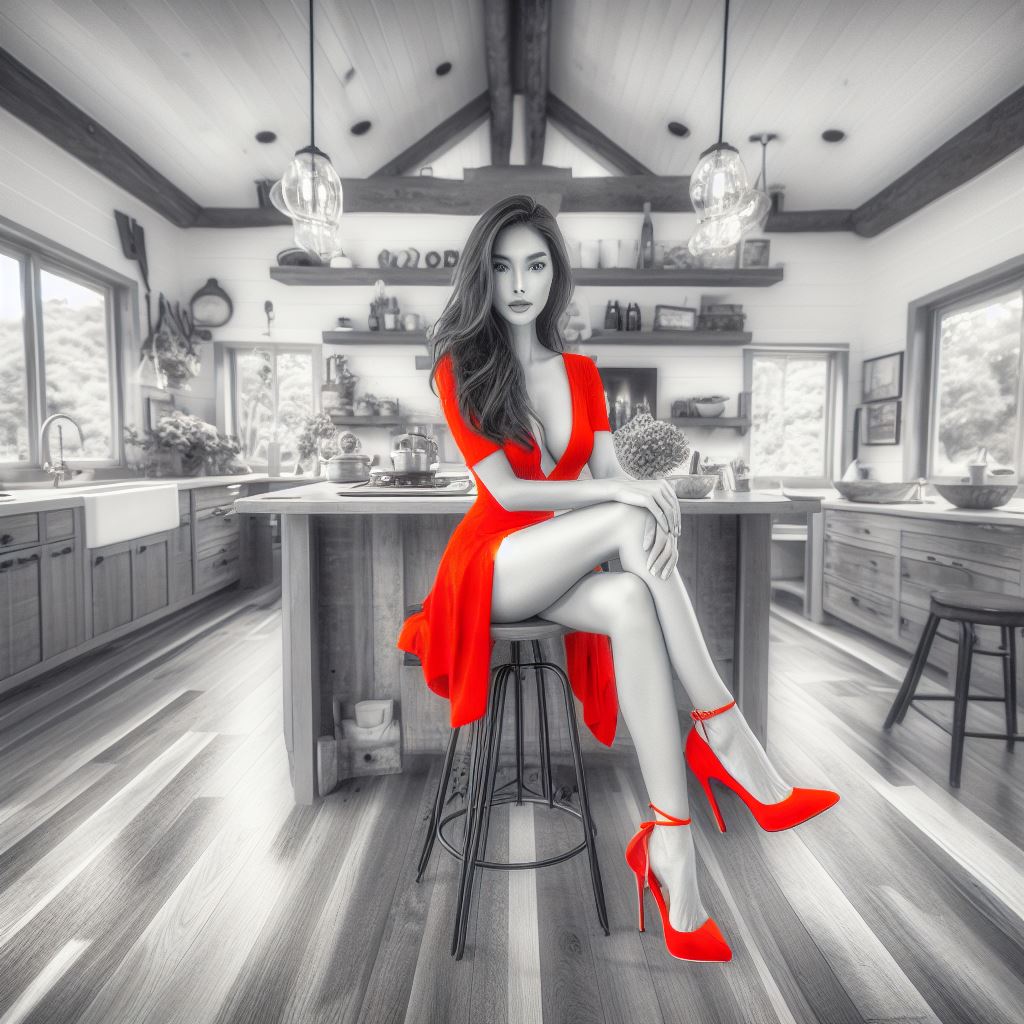
In Rust, an enum
can contain variants that allow different types or associated data with each variant. You can define functions that operate on these associated data by implementing methods for the enum. Note the enum + impl rater than the common struct + impl
Webdock
Fast Cloud VPS Linux Hosting
Example code:
In this example, the Shape
enum represents three different geometric shapes: Square
, Circle
, and Triangle
. The area()
method implemented for the Shape
enum calculates the area for each respective shape.
pub enum Shape {
Square(f64),
Circle(f64),
Triangle { base: f64, height: f64 },
}
When area()
is called on a Shape
instance, it pattern-matches the enum variant and calculates the area based on the associated data of each variant. The main function demonstrates using this method to calculate the areas of a square, circle, and triangle.
This example shows how you can implement a method within an enum to perform different operations based on the variant and its associated data. The match
statement within the method enables different behaviour for each variant of the enum.