Enum in a Class in Python
Enum, short for “enumerate” is a robust way for us to enforce a strict set of options in Python. For example a fixed choice of Aeroplane Manufacturers and each choice manufacturers matches a model name.
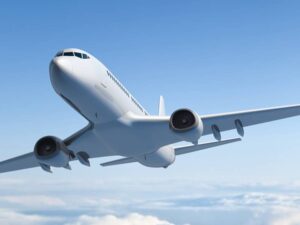
Let’s look at the example below and see we can do this can be done in practice.
Example Code
from enum import Enum
# enum
class Planes(Enum):
Airbus = "A380"
Boeing = "737"
Example = "373"
a = Planes.Airbus.value
print("Value = ",a)
Value = A380
Pass an enum value as a parameter to a class
# __str__
class AircraftModel:
def __init__(self, plane):
self.plane = plane
def __str__(self):
return self.plane
aircraftmodel = AircraftModel(Planes.Airbus.value)
print(f"Airbus Model = {aircraftmodel}")
Airbus Model = A380
Use a generator to access the values in a Class
The generator is a more memory efficient way of working with iterables. See how yield is used rather than “return”
# generator comprehension
def gencomp(Planes):
for i in Planes:
yield i
print("type = ",type(gencomp(Planes)))
""" lazy evaluation means that the object is evaluated
when it is needed, not when it is created """
print("Models in the Enum")
for i in gencomp(Planes):
print("\t",i.value)
Airbus Model = A380
type = <class 'generator'>
Models in the Enum
A380
737
373
Conclusion
Try the code shown here and learn how enums can be created and how to access the value and name from within.
The example of a generator is an efficient way to only evaluate the object when needed.
The full code :
from enum import Enum
# enum
class Planes(Enum):
Airbus = "A380"
Boeing = "737"
Example = "373"
a = Planes.Airbus.value
print("Value = ",a)
# __str__
class AircraftModel:
def __init__(self, plane):
self.plane = plane
def __str__(self):
return self.plane
aircraftmodel = AircraftModel(Planes.Airbus.value)
print(f"Airbus Model = {aircraftmodel}")
# generator comprehension
def gencomp(Planes):
for i in Planes:
yield i
print("type = ",type(gencomp(Planes)))
""" lazy evaluation means that the object is evaluated
when it is needed, not when it is created """
print("Models in the Enum")
for i in gencomp(Planes):
print("\t",i.value)
The python documentation also has some examples you can try out. If you’re trying enum for the first time remember to import with “from enum import Enum“.
Tip
Other languages such as Rust use enumerate frequently so it’s a good thing to understand. Remember that although the syntax is similar to that used with a Python dictionary you use “=” rather than “:” between the name and the value!