Sphinx : How to Document A Python Project
Let’s look at how to create complete documentation for your entire Python project using Sphinx
Here is a guide to how to document a very small project, with 2 directories “src” and “docs” where all the source code is in “src” and the Sphinx specific files go inside “docs”.
You’ll be able to view each docstring, function, class and the source code from within this documentation as a web page, PDF or Latex file.
You’ll notice that this is the same format as used by https://pypi.org/ if you use the theme ‘sphinx_rtd_theme’.
Getting started
Shown below is the bare bones of my project, in the “src” directory along with an empty “docs” directory as the starting point.
~/Doc/python/Abacus
.
├── docs
└── src
├── get_mb.py
├── __init__.py
├── pr_hi.py
└── __pycache__
pip install Sphinx
pip install sphinx sphinx_rtd_theme
cd docs
sphinx-quickstart
vim conf.py
import os
import sys
sys.path.insert(0, os.path.abspath('..'))
project = 'ABACUS'
copyright = '2022, Mr Moo'
author = 'Mr Moo'
release = '0.0.1'
extensions = [
'sphinx.ext.autodoc',
'sphinx.ext.viewcode',
'sphinx.ext.napoleon'
]
templates_path = ['_templates']
exclude_patterns = ['_build', 'Thumbs.db', '.DS_Store']
html_theme = 'sphinx_rtd_theme'
html_static_path = ['_static']
cd ..
From project’s top directory:
sphinx-apidoc -o docs src/
├── docs
│ ├── _build
│ ├── conf.py
│ ├── index.rst
│ ├── make.bat
│ ├── Makefile
│ ├── modules.rst
│ ├── src.rst
│ ├── _static
│ └── _templates
└── src
├── get_mb.py
├── __init__.py
├── pr_hi.py
└── __pycache__
Add ‘modules’ to index.rst
...
.. toctree::
:maxdepth: 2
:caption: Contents:
modules
...
Inside modules.rst add the names of your python files, but without the “.py”
.. toctree::
:maxdepth: 4
get_mb
pr_hi
from inside docs directory :make html
to redo:
go to docs:
make clean html
make html
cd to Abacus/docs/_build/html and open “index.html” in a browser, or “firefox index.html” from cli.
View the output (html)
~/Documents/python/Abacus/docs/_build/html
.
├── genindex.html
├── index.html <------------------------------- This one
├── _modules
│ ├── index.html
│ └── src
├── modules.html
├── objects.inv
├── py-modindex.html
├── search.html
├── searchindex.js
├── _sources
│ ├── index.rst.txt
│ ├── modules.rst.txt
│ └── src.rst.txt
├── src.html
└── _static
├── basic.css
├── css
├── doctools.js
├── documentation_options.js
├── file.png
├── js
├── language_data.js
├── minus.png
├── plus.png
├── pygments.css
├── searchtools.js
└── sphinx_highlight.js
Successful Documentation
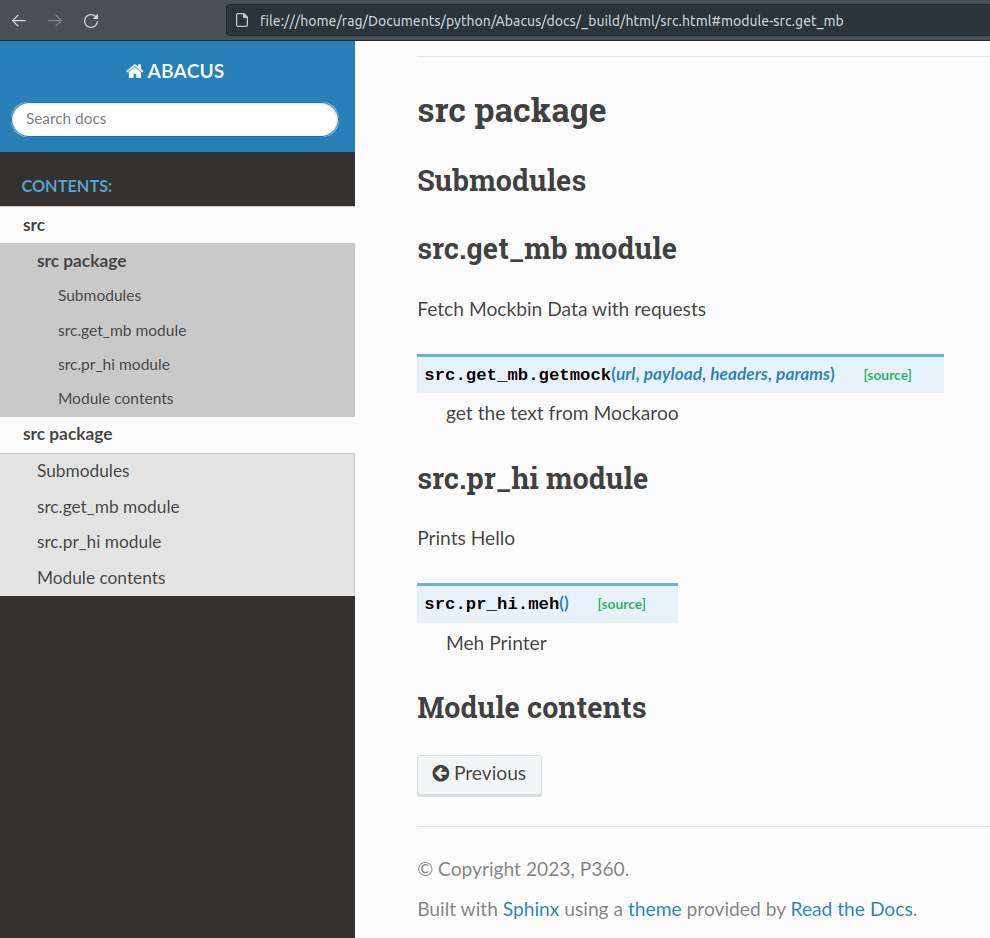
If you have any issues check that you have files in the correct directories, and that you have edited “conf.py” and “index.rst” correctly.
If you would like to copy an example project to see the process clone : https://github.com/Raxy45/sphinx_basics and you can build example documentation from the repo provided.
Note: The more descriptive docstrings and comments you use your code, the better your documentation will be.
As always don’t add unnecessary or obvious comments.
It may only benefit if a comment tells you where it’s stored or something you won’t already know.
Credit : https://towardsdatascience.com/documenting-python-code-with-sphinx-554e1d6c4f6d
Check out my other article on how to automate documentation to GitHub pages
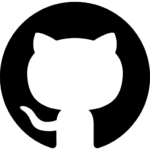