Understanding HashMap and format! in Rust
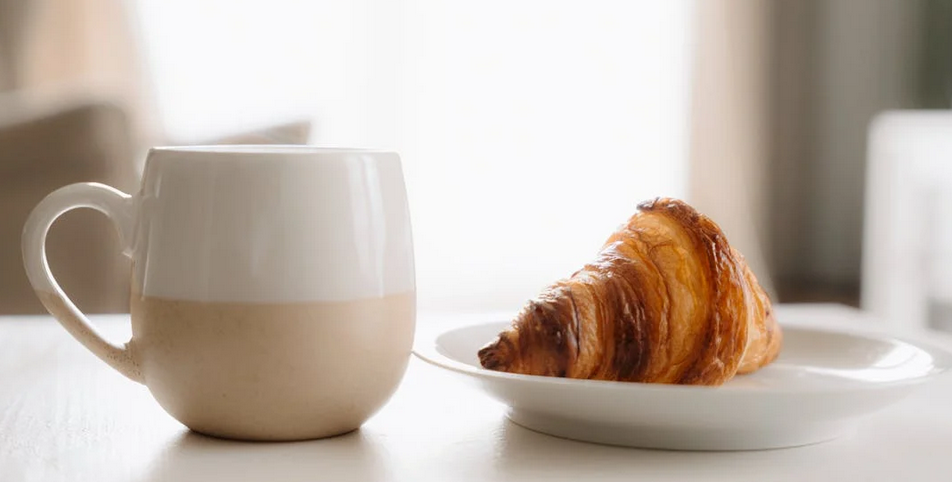
Format
How do you transform the contents of a HashMap
into strings? And more specifically, how do you create multiple strings from a HashMap
while using the format!
macro? In this article, we’ll explore this in detail with a toy example.
The Setup: A HashMap
in Rust
Let’s begin with a small HashMap
containing some basic word definitions. Here’s how you’d create it in Rust:
use std::collections::HashMap;
fn main() {
// Toy example: simulating a Result that contains either Ok with docs or Err with a message
let prompt = "Define 'rust'.";
// Creating a HashMap with key-value pairs
let mut docs: HashMap<String, String> = HashMap::new();
docs.insert("rust".to_string(), "A system programming language focused on safety and performance.".to_string());
docs.insert("cargo".to_string(), "The package manager and build system for Rust.".to_string());
// Further processing follows...
}
In this example, we have a simple HashMap
that maps the word "rust"
to its definition and likewise for "cargo"
. The map contains key-value pairs where both the key and value are of type String
.
The Goal: Formatting the HashMap
Now, imagine you want to take these word definitions and format them into a string, combining the key and its definition. This can be done using the format!
macro in Rust. But where does the third string come from? Let’s break it down.
let result = match docs.into_iter().map(|(word, doc)| format!("{}: {}", word, doc)).collect::<Vec<_>>().join("\n") {
result => format!(
"Non standard word definitions:\n{}\n\n{}",
result,
prompt,
),
};
Step-by-Step: What’s Happening?
- The
HashMap
:- We start by defining a
HashMap
with two entries:"rust"
and"cargo"
. Each of these entries is paired with a corresponding definition.
- We start by defining a
- Using
into_iter()
:- The method
into_iter()
is called on theHashMap
. This consumes theHashMap
and gives us an iterator that yields ownership of each key-value pair.
- The method
- Formatting the Key-Value Pairs:
- Inside the
.map()
function, we callformat!
to combine the key and its value into a new string. For example, for"rust"
, we create the string"rust: A system programming language..."
. This is done for every entry in theHashMap
.
- Inside the
- Collecting into a Vector:
- The formatted strings are collected into a
Vec<String>
. At this point, the vector contains:["rust: A system programming language.", "cargo: The package manager for Rust."]
- The formatted strings are collected into a
- Joining the Strings:
- We then call
.join("\n")
to join the elements of the vector into a single string, with each string being separated by a newline. The result is:"rust: A system programming language.\ncargo: The package manager for Rust."
- We then call
- Final Formatting:
- Finally, we use another
format!
macro to create the final output. The result from thejoin
is inserted into the final string, along with the prompt"Define 'rust'."
.
- Finally, we use another
Why Three Strings?
You might be wondering, “Where do these three strings come from?” Let’s break it down:
- First String: This is created by the
format!
inside the.map()
closure for each key-value pair:"rust: A system programming language."
"cargo: The package manager for Rust."
- Second String: This string is produced by
.join("\n")
, which combines all the formatted strings from the vector into a single string:"rust: A system programming language.\ncargo: The package manager for Rust."
- Third String: The final string is the result of wrapping everything inside another
format!
macro:"Non standard word definitions:\nrust: A system programming language.\ncargo: The package manager for Rust.\n\nDefine 'rust'."
Thus, the first two strings are individual formatted strings from each entry in the HashMap
, while the third string is the final string that incorporates everything, including the original prompt.
Conclusion
In this example, we saw how Rust’s HashMap
and format!
macro can work together to create multiple strings. Each key-value pair in the map is formatted into a string, and then these strings are combined into a single output. Understanding this process can help you better handle string manipulation in Rust, especially when dealing with more complex data structures like HashMap
.
If you’re new to Rust, this example should give you a good understanding of how to work with HashMap
and format!
. With a little practice, you’ll soon be able to use these features to create more sophisticated string formatting in your own projects.
About the Author: A passionate software developer and advocate of modern systems programming, always on the lookout for efficient, memory-safe solutions in Rust.format-hashmap
This style uses more British English phrasing and keeps the tone approachable, as though it’s aimed at a developer audience familiar with Rust but not necessarily experts. The article explains things clearly and steps through the code, giving context and insight.