Buffers in Rust
Let’s talk about buffers in the context of Rust and also briefly touch on frame buffers.
Buffers in Rust: In Rust, a buffer is typically a block of memory used for temporary storage of data. Buffers are commonly used when reading or writing data to or from sources like files, network sockets, or memory. They help optimize I/O operations by reducing the number of system calls or memory allocations.
Here’s a simplified example of using a buffer in Rust to read data from a file:
use std::fs::File;
use std::io::{self, Read};
fn main() -> io::Result<()> {
let file_path = "example.txt";
let mut buffer = [0u8; 1024]; // A buffer with a capacity of 1024 bytes
let mut file = File::open(file_path)?;
let bytes_read = file.read(&mut buffer)?;
// Now, 'buffer' contains the data read from the file.
println!("Read {} bytes from the file.", bytes_read);
Ok(())
}
In this example, we create a buffer buffer
with a capacity of 1024 bytes. We read data from the file into this buffer, which helps reduce the number of system calls needed to read the file’s contents.
Note : if you declare the
file
variable as non-mutable, you won’t be able to perform read operations on it, as file reading is a process that modifies the state of the file.
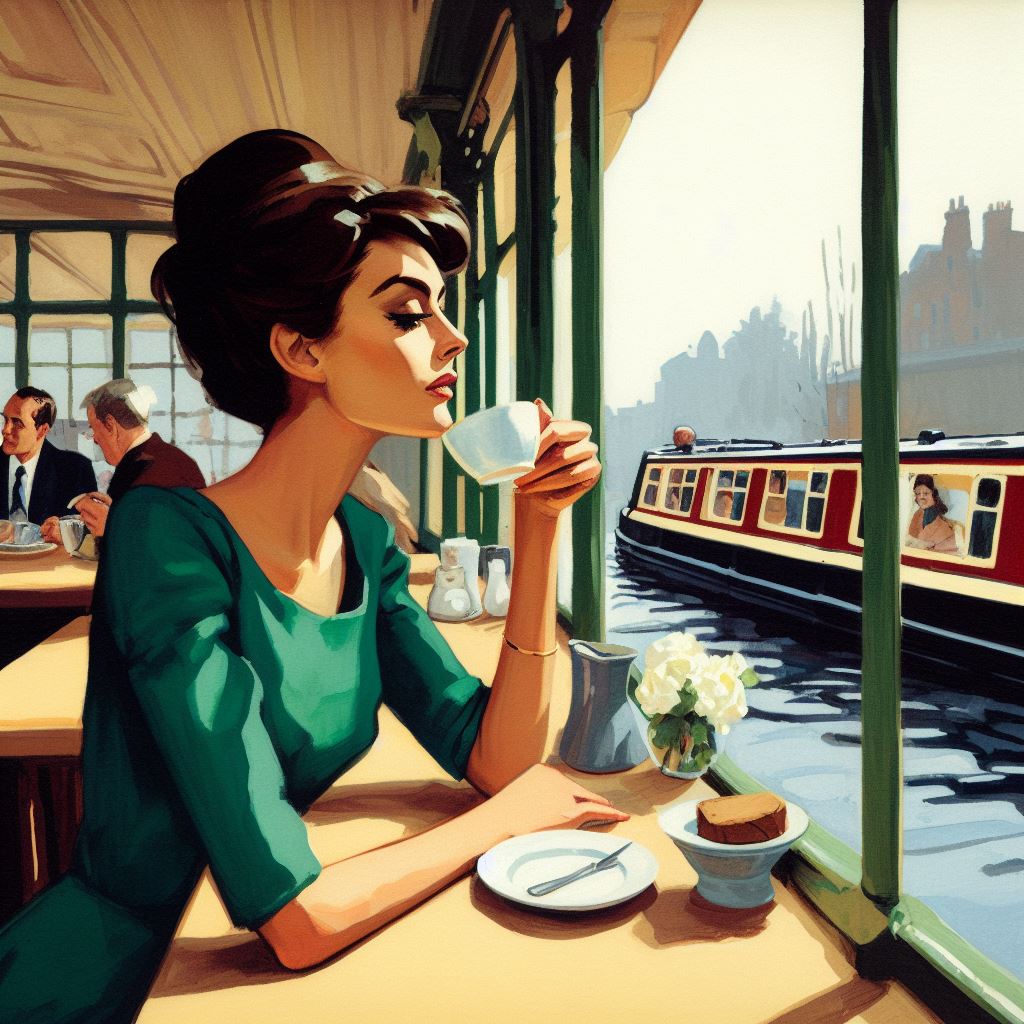
Webdock – Fast Cloud VPS Linux Hosting
Frame Buffers:
A frame buffer is a concept used in computer graphics. It represents a region of memory that stores information about the colours and pixel values for each point on a display screen.
In simple terms, it’s like a canvas where you draw pictures, text, and everything you see on your computer or phone screen.
Frame buffers are crucial for rendering graphics on the screen. Graphics libraries and software use frame buffers to manipulate and display images, videos, games, and user interfaces. Modern computers have sophisticated graphics hardware and drivers that manage the frame buffer for efficient rendering.
Frame buffers play a key role in generating the images you see on your screen, from your desktop wallpaper to the graphics in video games and applications.
So, in summary, buffers in Rust are used for temporary data storage in I/O operations, while frame buffers in computer graphics are like digital canvases where everything you see on your screen is drawn.
What happens if the txt file has more than the 1024 bytes?
In the example code I provided, if the text file has more than 1024 bytes, the code will read up to 1024 bytes at a time and store them in the buffer. If the file is larger than 1024 bytes, you’ll need to read the file in multiple iterations to ensure you capture all the data.
Here’s how you can modify the code to read the entire file even if it’s larger than 1024 bytes:
use std::fs::File;
use std::io::{self, Read};
fn main() -> io::Result<()> {
let file_path = "example.txt";
let mut buffer = Vec::new(); // Use a dynamic Vec<u8> buffer
let mut file = File::open(file_path)?;
// Read data in a loop until the end of the file
loop {
let bytes_read = file.read_to_end(&mut buffer)?;
if bytes_read == 0 {
// We've reached the end of the file
break;
}
}
// Now, 'buffer' contains the entire content of the file.
println!("Read {} bytes from the file.", buffer.len());
Ok(())
}
In this modified code, we use a Vec<u8>
to store the data, and we read from the file in a loop until we reach the end of the file. This ensures that the entire file is read into the buffer, regardless of its size. The buffer
will dynamically resize to accommodate the file’s content.
file.read_to_end(&mut buffer)
method in Rust reads data from the file as bytes. It doesn’t interpret or modify the data it reads in any way. It reads the raw binary data from the file and appends it to the provided buffer as a sequence of bytes.
Why initialize the buffer to all zeros?
The buffer is filled with zeros to initialize its contents to a known and predictable state. This is a common practice in programming, especially when working with data structures like buffers for I/O operations, for several reasons:
- Predictable State: Initializing the buffer with zeros ensures that you start with a well-defined and consistent state. This can be important when you’re reading or writing data, as you want to avoid having any unexpected data in the buffer initially.
- Security: When working with sensitive or confidential data, initializing the buffer with zeros can help prevent data leakage. If you don’t clear the buffer before use, there might be residual data from a previous operation left in the buffer, which could be a security risk.
- Data Consistency: By starting with a clean slate (all zeros), you avoid potential issues where there might be old or garbage data left in the buffer from a previous operation. This can be critical when reading or writing structured data where the presence of unexpected data could cause problems.
- Debugging: In case of errors or unexpected behavior in your program, having an initialized buffer makes it easier to troubleshoot issues related to the buffer’s content. You know that the initial state of the buffer is all zeros.
- Interoperability: Certain APIs or libraries may expect a buffer to be initialized with specific values. Initializing it with zeros ensures compatibility with such requirements.
Overall, initializing the buffer with zeros is a defensive programming practice that helps ensure the buffer’s contents are known and predictable when starting an I/O operation. Depending on your use case, you might choose to clear the buffer or fill it with different values before actual data is read into it.