Understanding Rust’s From Trait
In Rust, the From
trait plays a crucial role in allowing the conversion of one type into another. Let’s explore this concept through a straightforward example involving the conversion of lengths from feet to meters.
Struct Definitions
// Define a simple struct named `Feet` to represent a length in feet.
struct Feet {
value: f64,
}
// Define another struct named `Meters` to represent a length in meters.
struct Meters {
value: f64,
}
Here, we have two structs, Feet
and Meters
, each representing a length in a different unit.
From
Trait Implementation
// Implement the `From` trait to convert from `Feet` to `Meters`.
impl From<Feet> for Meters {
fn from(feet: Feet) -> Self {
// Conversion logic: 1 foot is approximately 0.3048 meters.
let meters_value = feet.value * 0.3048;
Meters { value: meters_value }
}
}
This code snippet shows how we implement the From
trait for the conversion from Feet
to Meters
. The conversion logic is applied in the implementation, making it clear and concise.
Main Function
fn main() {
// Create an instance of `Feet`.
let feet_length = Feet { value: 10.0 };
// Convert the length from `Feet` to `Meters` using the `Into` trait.
let meters_length: Meters = feet_length.into();
// Print the result.
println!("Length in meters: {:.2}", meters_length.value);
}
In the main
function, we showcase the practical usage of the conversion. We create an instance of Feet
, convert it to Meters
using the into()
method, and print the result.
This example serves to illustrate how the From
trait facilitates seamless conversions between different types in Rust. It is particularly useful when dealing with scenarios like unit conversions or working with different representations of data.
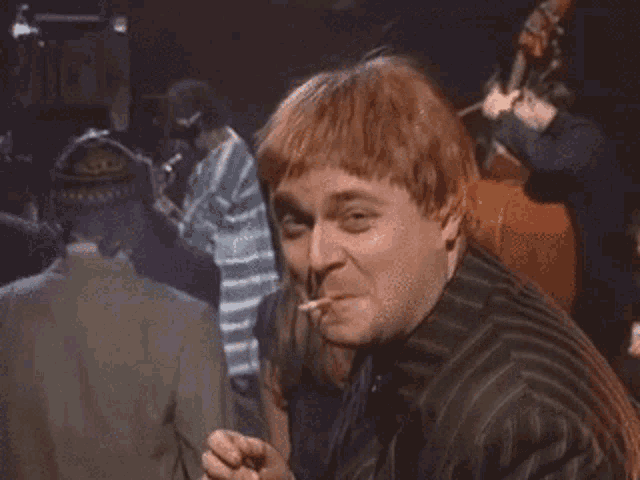
Rust – from trait – example code in Rust Playground
Output
Length in meters: 3.05
And finally, there is another way to achieve this using “into” – see the “Learning Rust” video below