iced.rs – example snippets (version 0.13)
Code plus screenshots – how to manipulate the layout in Rust + iced.rs
Credit : https://jl710.github.io/iced-guide/index.html
© Héctor Ramón (hecrj) for the iced logo.
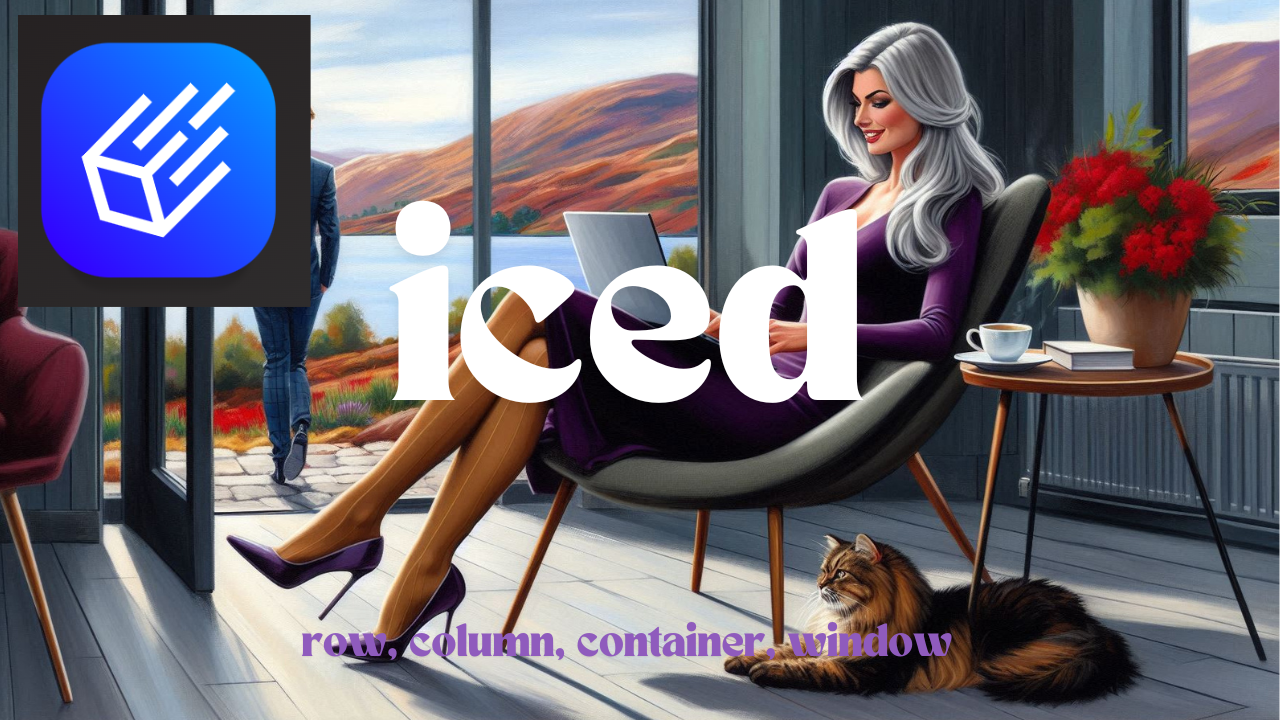
use iced::widget;
struct Counter {
// This will be our state of the counter app
// a.k.a the current count value
count: i32,
}
#[derive(Debug, Clone, Copy)]
enum Message {
// Emitted when the increment ("+") button is pressed
IncrementCount,
// Emitted when decrement ("-") button is pressed
DecrementCount,
}
// Implement our Counter
impl Counter {
fn new() -> Self {
// initialize the counter struct
// with count value as 0.
Self { count: 0 }
}
fn update(&mut self, message: Message) -> iced::Task<Message> {
// handle emitted messages
match message {
Message::IncrementCount => self.count += 1,
Message::DecrementCount => self.count -= 1,
}
iced::Task::none()
}
fn view(&self) -> iced::Element<'_, Message> {
let row = widget::row![
widget::button("-").on_press(Message::DecrementCount),
widget::text(self.count.to_string()),
widget::button("+").on_press(Message::IncrementCount)
];
row.into()
}
}
fn main() -> Result<(), iced::Error> {
// run the app from main function
iced::application("Counter Example", Counter::update, Counter::view)
.run_with(|| (Counter::new(), iced::Task::none()))
}
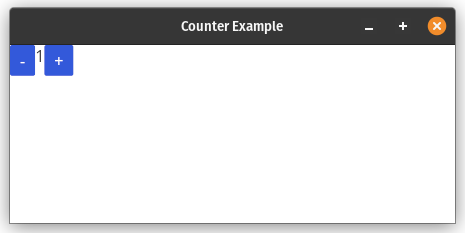
just using “row”
fn view(&self) -> iced::Element<'_, Message> {
let column = widget::column![
widget::button("-").on_press(Message::DecrementCount),
widget::text(self.count.to_string()),
widget::button("+").on_press(Message::IncrementCount)
].align_x(iced::Alignment::Center);
column.into()
}
Change “row” to “column”
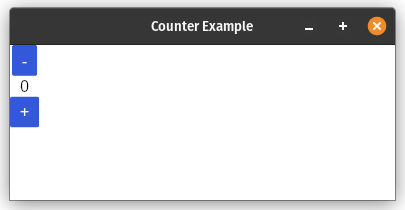
just using “column”
fn view(&self) -> iced::Element<'_, Message> {
let column = widget::column![
widget::button("-").on_press(Message::DecrementCount),
widget::text(self.count.to_string()),
widget::button("+").on_press(Message::IncrementCount)
];
let stuff_centered: Container<'_, Message, Theme, Renderer> =
widget::Container::new(column)
.align_x(Horizontal::Center)
.align_y(Vertical::Center)
.width(Length::Fill)
.height(Length::Fill);
stuff_centered.into()
}
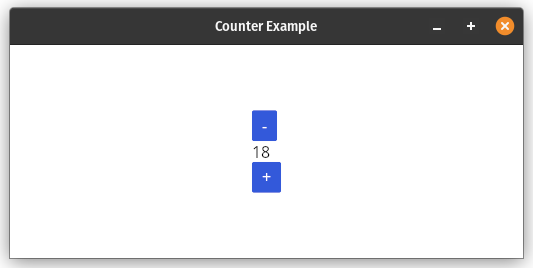
Column is centered…but not the text! 🤔
fn view(&self) -> iced::Element<'_, Message> {
let column = widget::column![
widget::button("-").on_press(Message::DecrementCount),
widget::text(self.count.to_string()),
widget::button("+").on_press(Message::IncrementCount)
].align_x(Horizontal::Center);
let stuff_centered: Container<'_, Message, Theme, Renderer> =
widget::Container::new(column)
.align_x(Horizontal::Center)
.align_y(Vertical::Center)
.width(Length::Fill)
.height(Length::Fill);
stuff_centered.into()
}
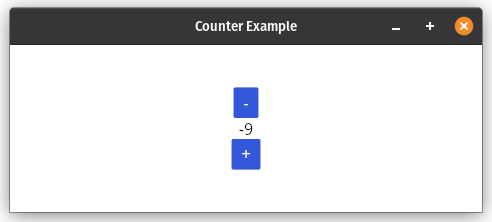
Column is centered and the text! 😀
Centre the window – iced.rs – 0.13
fn main() -> Result<(), iced::Error> {
// run the app from main function
iced::application("Counter Example", Counter::update, Counter::view)
.window(window::Settings{position:Position::Centered,..Default::default()})
.run_with(|| (Counter::new(), iced::Task::none()))
}
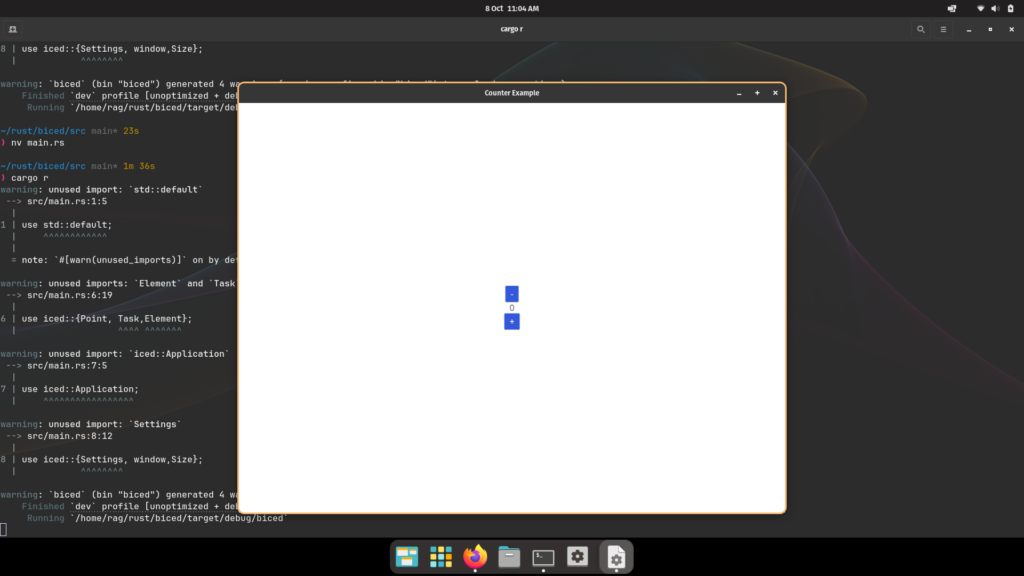
Resize the window – iced.rs – 0.13
Size is a struct that you must to import and instantiate to be able to use here…
use iced::{window, Settings, Size};
https://docs.rs/iced/latest/iced/struct.Size.html
fn main() -> Result<(), iced::Error> {
// run the app from main function
iced::application("Counter Example", Counter::update, Counter::view)
.window(window::Settings {
position: Position::Centered,
resizable: false,
size: Size::new(200.0, 400.0),
..Default::default()
})
.run_with(|| (Counter::new(), iced::Task::none()))
}
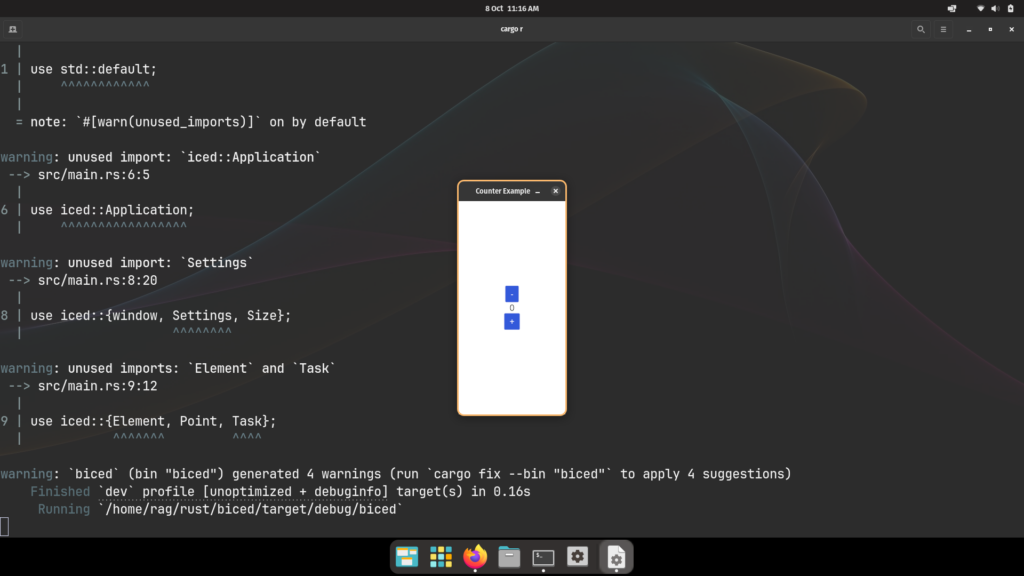
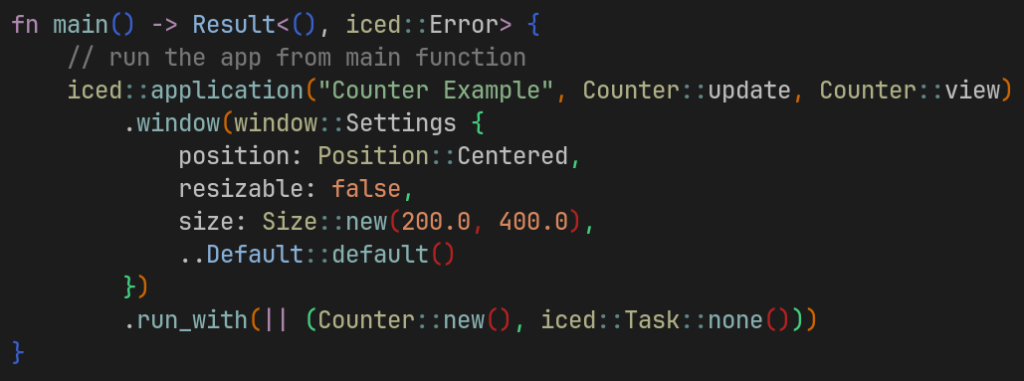
iced rows, columns, containers, windows