Code from code: Automating Bitcoin Price Fetching with OpenAI and Python
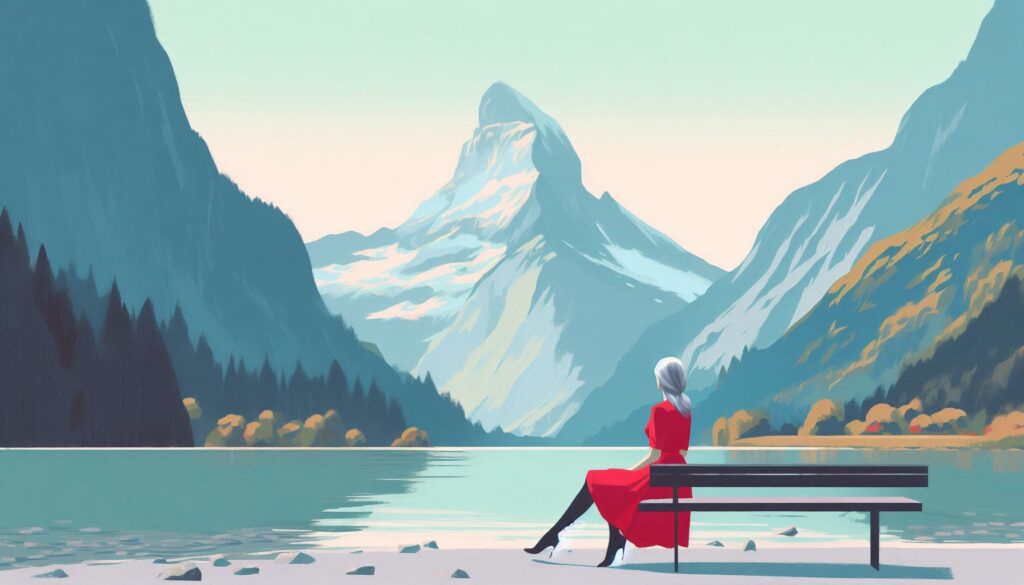
In this tutorial, we’ll demonstrate how to generate and execute a Python script dynamically using OpenAI’s API. The script will fetch the latest Bitcoin price from the Gemini API and display the result. This approach leverages AI to automate script creation, reducing manual effort and enhancing efficiency.
Prerequisites
To follow along, ensure you have:
- Python installed on your system
- An OpenAI API key (set as an environment variable:
OPENAI_API_KEY
) - Internet connectivity
Step 1: Setting Up the Environment
The script fetches an OpenAI-generated Python script dynamically. First, we obtain the OpenAI API key from the environment:
import os
import requests
import subprocess
import shutil
from pathlib import Path
api_key = os.getenv("OPENAI_API_KEY")
if not api_key:
raise ValueError("OPENAI_API_KEY not set in environment")
Step 2: Requesting a Script from OpenAI
We send a request to OpenAI’s API, instructing it to generate a script that retrieves Bitcoin prices from Gemini’s API.
url = "https://api.openai.com/v1/chat/completions"
prompt = ("Generate a Python script that fetches the bitcoin price from Gemini API - just give the code, so it can be run, "
"no smalltalk! - no backticks either ok, just code")
headers = {
"Authorization": f"Bearer {api_key}",
"Content-Type": "application/json"
}
data = {
"model": "gpt-4",
"messages": [{"role": "user", "content": prompt}],
"max_tokens": 100
}
response = requests.post(url, headers=headers, json=data)
response_json = response.json()
script = response_json.get("choices", [{}])[0].get("message", {}).get("content", "print('No response from AI')")
Step 3: Saving and Executing the Script
Once the script is generated, we save it as a Python file and execute it:
script_path = Path("script.py").resolve()
with open(script_path, "w") as file:
file.write(script)
print("Python script generated:", script_path)
python_command = shutil.which("python") or shutil.which("python3")
if not python_command:
raise RuntimeError("Python interpreter not found.")
process = subprocess.run([python_command, str(script_path)], capture_output=True, text=True)
if process.returncode != 0:
print("Error running Python script:", process.stderr)
else:
print("Python script output:", process.stdout)
Conclusion
This showcases how AI can automate coding tasks. By integrating OpenAI’s API, you can dynamically generate, modify, and execute scripts for various tasks, such as fetching real-time cryptocurrency prices.