Byte Array and String Conversion in Rust
In Rust, working with byte arrays (Vec<u8>
) and strings (String
or &str
) is a common task, especially when dealing with raw data or low-level processing. Knowing how to convert between these types is very handy, particularly when you’re dealing with UTF-8 encoded strings yeah?
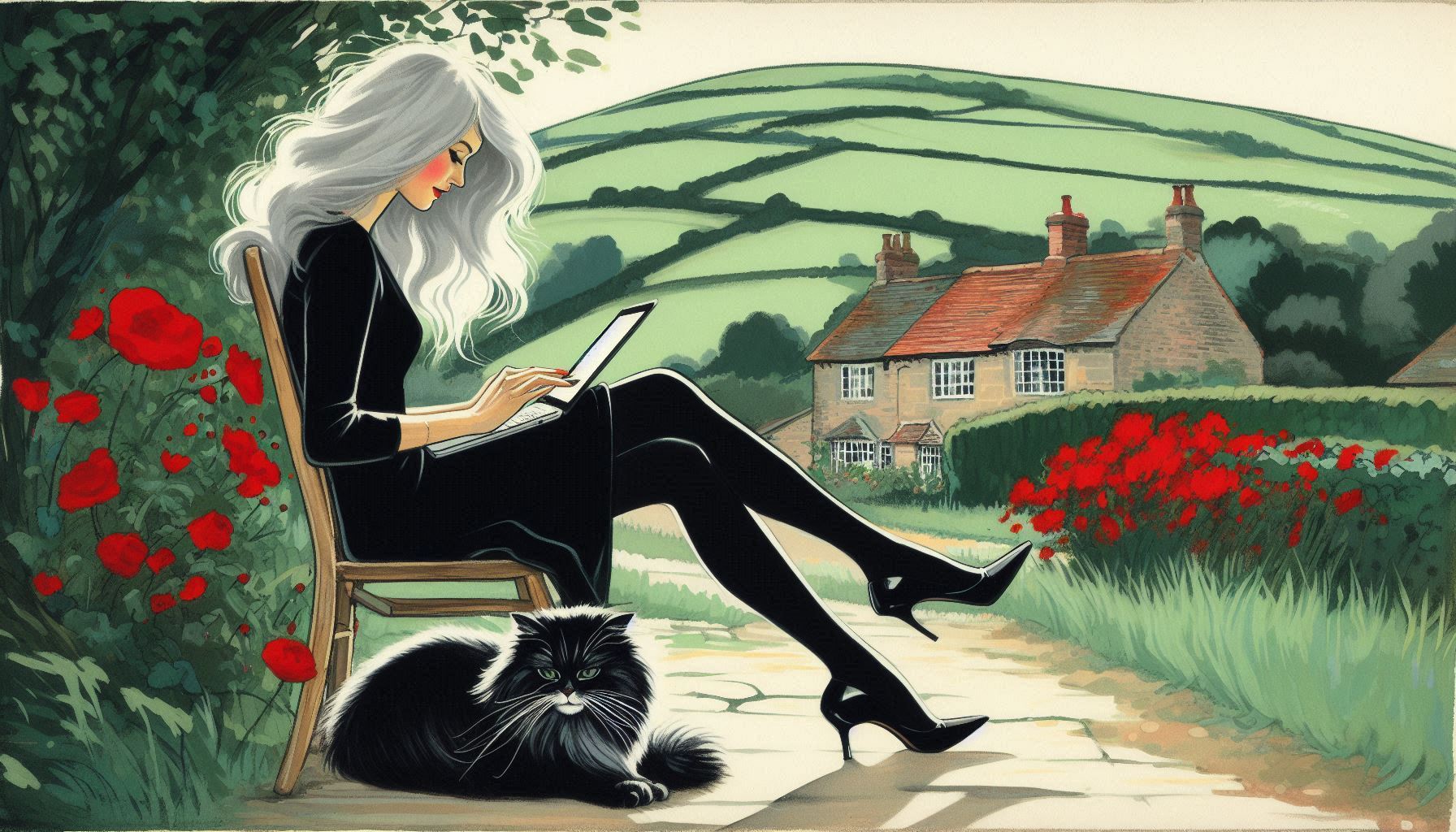
how to convert byte data
Example :
fn main() {
let bond_token_name: Vec<u8> = "BondToken".as_bytes().to_vec();
let bond_token_symbol: Vec<u8> = "BND".as_bytes().to_vec();
// Print the byte representation
println!("Token Name (bytes): {:?}", bond_token_name);
println!("Token Symbol (bytes): {:?}", bond_token_symbol);
// Clone the Vec<u8> to retain ownership for later conversion to &str
let name_str = String::from_utf8(bond_token_name.clone()).unwrap();
let symbol_str = String::from_utf8(bond_token_symbol.clone()).unwrap();
println!("Token Name (string): {}", name_str);
println!("Token Symbol (string): {}", symbol_str);
// Convert Vec<u8> back to &str (using from_utf8 for safe conversion)
let name_str_slice = std::str::from_utf8(&bond_token_name).unwrap();
let symbol_str_slice = std::str::from_utf8(&bond_token_symbol).unwrap();
println!("Token Name (str slice): {}", name_str_slice);
println!("Token Symbol (str slice): {}", symbol_str_slice);
}
Rust’s Vec<u8>
is a dynamic array that holds bytes. A string in Rust is a sequence of UTF-8 encoded bytes, and String
is the owned version of a string, while &str
is a reference to a string slice. The conversion between Vec<u8>
and String
is straightforward using the String::from_utf8
method, which attempts to convert the bytes into a valid UTF-8 string. If the byte sequence is not valid UTF-8, it will return an error, which can be handled with unwrap()
or proper error handling.
To convert from a Vec<u8>
to a String
, the String::from_utf8
method is typically used. This method consumes the Vec<u8>
and returns a Result<String, FromUtf8Error>
. When the bytes are valid UTF-8, it will return an Ok
with the converted String
; otherwise, it will return an error. For example:
let bytes: Vec<u8> = "Hello".as_bytes().to_vec();
let string = String::from_utf8(bytes).unwrap(); // Converts Vec<u8> to String
To convert from a Vec<u8>
to a &str
, the std::str::from_utf8
function is used. This function accepts a byte slice (&[u8]
) and returns a Result<&str, Utf8Error>
, which is a borrowed reference to the string. This method does not consume the original data and allows for more efficient memory usage:
let bytes: Vec<u8> = "Hello".as_bytes().to_vec();
let str_slice = std::str::from_utf8(&bytes).unwrap(); // Converts Vec<u8> to &str
In summary, converting between byte arrays and strings in Rust is critical for many applications, especially when handling raw data or interacting with external systems. By using String::from_utf8
and std::str::from_utf8
, you can seamlessly convert byte data into human-readable strings while ensuring UTF-8 compatibility.
https://gist.github.com/rust-play/1df71cd623c11650ff9564dcb389f4b2