I asked ChatGPT for Rust code – does it work?
Did the code from ChatGPT compile straight away? No. Keep reading to see what I had to fix…
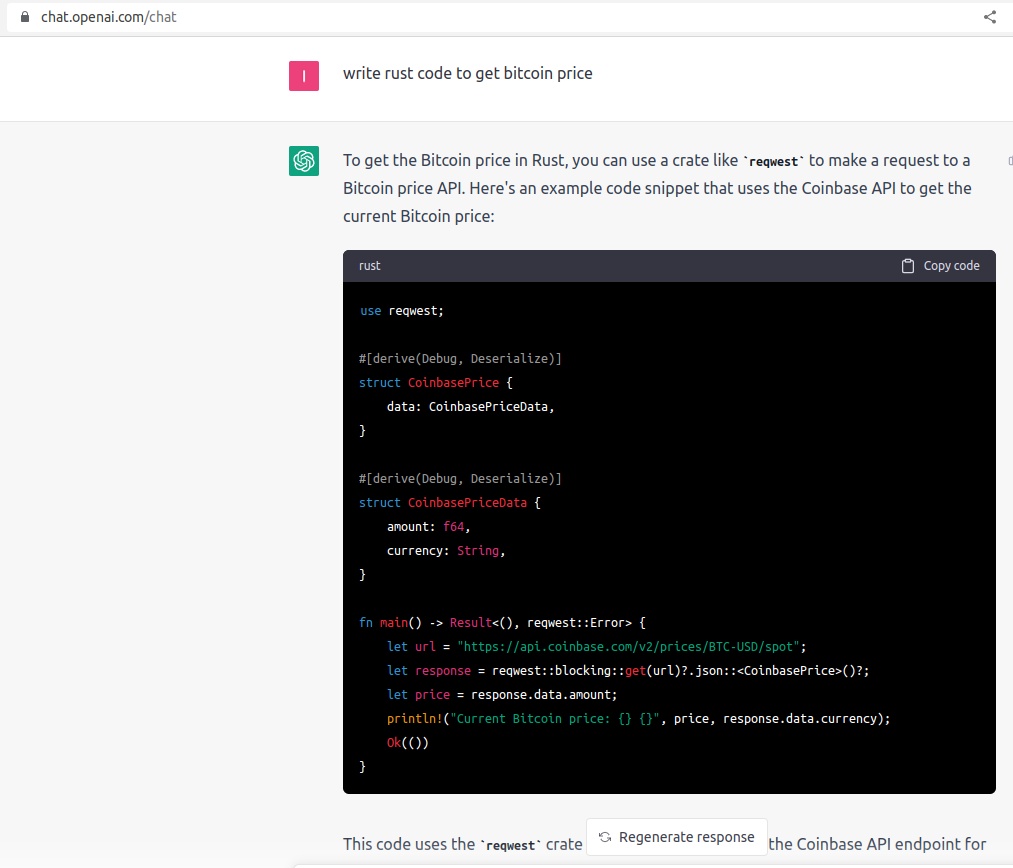
The Cargo file is the main reason why it didn’t compile, and interestingly the screenshot here uses the Coinbase API, but an earlier question to ChatGPT replied with code using CoinGecko.
Each time the issues were with the serde and reqwest syntax required for the Cargo.toml file.
Working code (Does compile)
use reqwest;
use serde::Deserialize;
#[derive(Debug, Deserialize)]
struct CoinbasePrice {
data: CoinbasePriceData,
}
#[derive(Debug, Deserialize)]
struct CoinbasePriceData {
amount: String,
currency: String,
}
fn main() -> Result<(), reqwest::Error> {
let url = "https://api.coinbase.com/v2/prices/BTC-GBP/spot";
let response = reqwest::blocking::get(url)?.json::<CoinbasePrice>()?;
let price = response.data.amount;
println!("Current Bitcoin price: {} {:?}", price, response.data.currency);
Ok(())
}
The Cargo file shown below allowed a successful compilation
[package]
name = "gptbtc2"
version = "0.1.0"
edition = "2021"
# See more keys and their definitions at https://doc.rust-lang.org/cargo/reference/manifest.html
[dependencies]
serde_json = "1.0.94"
serde = { version = "1.0.154", features = ["derive"] }
tokio = { version = "1.26.0", features = ["full"] }
reqwest = { version = "0.11.14", features = ["blocking", "json"] }
Curiously this second attempt didn’t use “Tokio” with “Async” and “Await”. The first time I asked ChatGPT for the code it gave me this:
The other version that I got from ChatGPT (uses Tokio)
use reqwest;
use serde::Deserialize;
#[derive(Debug, Deserialize)]
struct CoinGeckoPrice {
bitcoin: PriceInfo,
}
#[derive(Debug, Deserialize)]
struct PriceInfo {
usd: f64,
}
#[tokio::main]
async fn main() -> Result<(), reqwest::Error> {
let url = "https://api.coingecko.com/api/v3/simple/price?ids=bitcoin&vs_currencies=usd";
let response = reqwest::get(url).await?.json::<CoinGeckoPrice>().await?;
println!("Bitcoin price: ${}", response.bitcoin.usd);
Ok(())
}
Conclusion
This is possibly easier than searching through StackOverflow provided you can correct the Cargo file. You can also paste in the compilation errors as well which may or may not lead you to the correct solution. In general the code is good, but it won’t compile unless you know what you’re doing with the Cargo.toml file.
Would I use this for assistance in future? Yes. Especially as it can give different results to what you might find elsewhere on the internet and it saves scrolling through StackOverflow checking to see if the highest rated result is more recent than 2011!
That is one of my biggest annoyances with finding code snippets, they can be very old, which for “C” might not be a problem but for Python or Rust it is a problem. Thanks for reading, I’ll be back soon with some more, original content. No adverts, no pop ups, no BS!
If you have a business enquiry please feel free to contact me via ‘contact’ at the top of this page.