Building a Merkle Tree in Rust: Step-by-Step Guide with Code
Merkle trees are a fundamental component in many blockchain and cryptographic systems. They allow for efficient and secure verification of large datasets. In this article, we’ll walk through how to build a Merkle tree in Rust, step-by-step, and provide the complete code at the end.
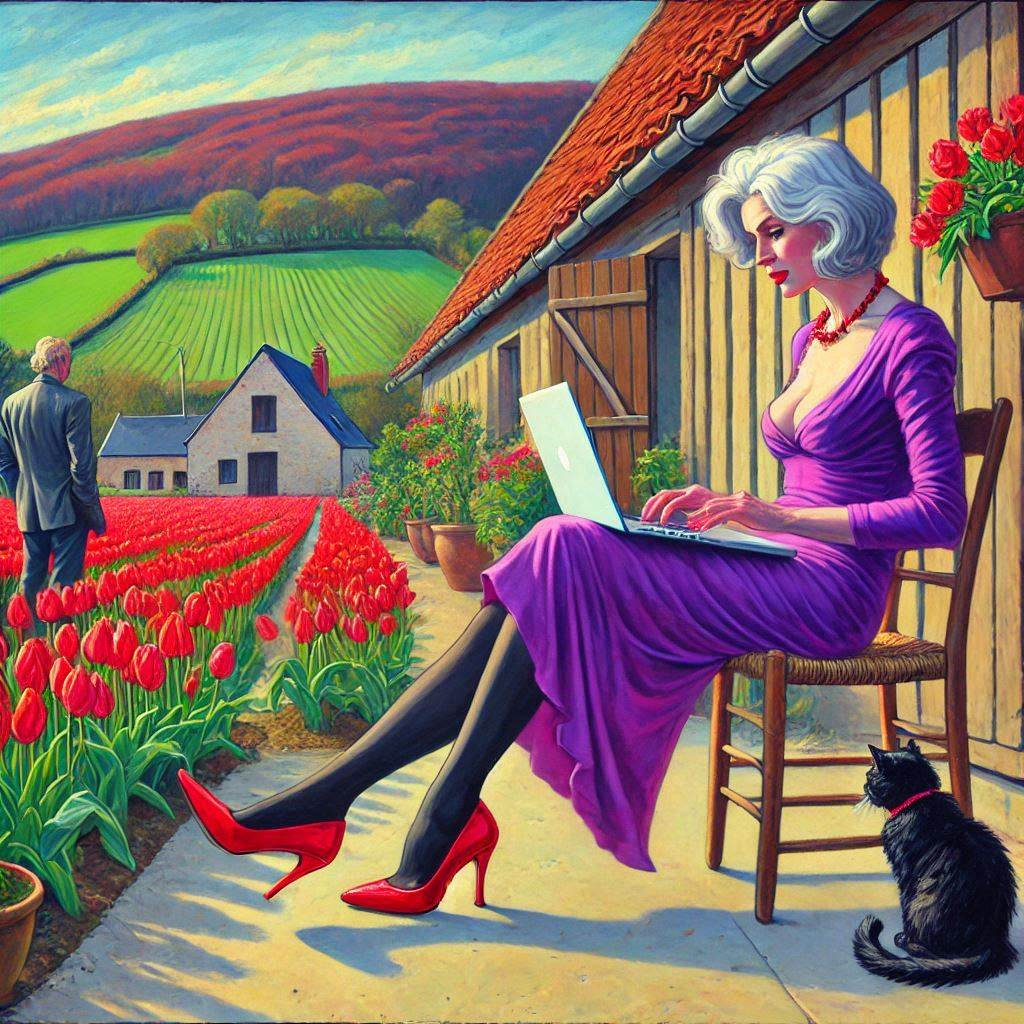
Building a Merkle Tree in Rust
Understanding the Process
Here’s how the construction of a Merkle tree works:
- Hash Each Leaf:
- Start by hashing each piece of data (leaf node) to create the first layer of the tree.
- Handle Odd Nodes:
- If the number of nodes in a layer is odd, duplicate the last node to ensure every node has a pair.
- Combine and Hash Pairs:
- Concatenate each pair of nodes in the current layer, hash the result, and create a new layer.
- Repeat Until a Single Node Remains:
- Continue combining and hashing pairs of nodes layer by layer until there’s only one node left. This node becomes the root of the Merkle tree.
- Output the Root Hash:
- The final remaining hash is the Merkle tree root, representing the entire dataset.
Complete Code Example
Here’s the Rust implementation of a Merkle tree:
use sha2::{Sha256, Digest};
/// Hashes a piece of data using SHA-256
fn hash_data(data: &str) -> String {
let mut hasher = Sha256::new();
hasher.update(data.as_bytes());
let result = hasher.finalize();
format!("{:x}", result) // Convert the hash to a hexadecimal string
}
/// Constructs a Merkle tree and returns the root hash
fn merkle_tree(leaves: Vec<&str>) -> String {
// Step 1: Hash all of the leaves to create the first layer of the tree
let mut layer: Vec<String> = leaves.iter().map(|&leaf| hash_data(leaf)).collect();
// Step 2–4: Build the tree upwards until a single node remains
while layer.len() > 1 {
// Step 2: If there's an odd number of nodes, duplicate the last one
if layer.len() % 2 != 0 {
layer.push(layer.last().unwrap().clone());
}
// Step 3: Combine and hash pairs of nodes
let mut new_layer: Vec<String> = Vec::new();
for i in (0..layer.len()).step_by(2) {
let combined = format!("{}{}", layer[i], layer[i + 1]);
new_layer.push(hash_data(&combined));
}
// Move to the new layer
layer = new_layer;
}
// Step 5: The remaining hash is the root of the Merkle tree
layer[0].clone()
}
/// Entry point: Demonstrates Merkle tree construction
fn main() {
let leaves = vec!["data1", "data2", "data3", "data4", "data5", "data6", "data7", "data8"];
let root_hash = merkle_tree(leaves);
println!("Merkle tree root hash: {}", root_hash);
}
How the Code Works
- Hashing Function:
- The
hash_data
function takes a string, hashes it using the SHA-256 algorithm, and returns the hexadecimal representation of the hash.
- The
- Merkle Tree Function:
- The
merkle_tree
function constructs the Merkle tree by repeatedly hashing pairs of nodes until a single root hash remains.
- The
- Main Function:
- The
main
function provides an example dataset (data1
todata8
), constructs the Merkle tree, and prints the resulting root hash.
- The
Try It Out
Paste this code into your Rust project to see it in action. You can modify the dataset or use it as part of a larger cryptographic or blockchain application. Understanding Merkle trees will give you a solid foundation for secure data verification.