HTLC – Atomic Swaps
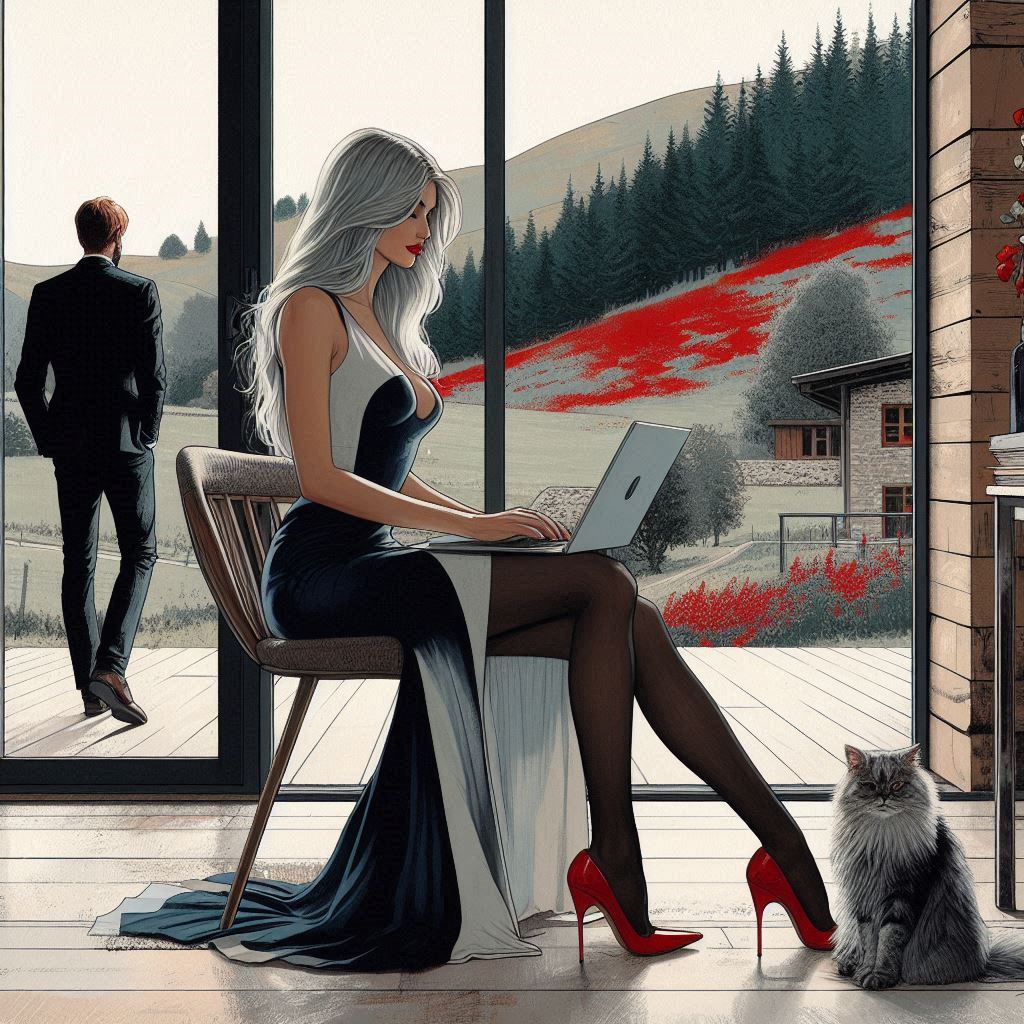
Hash Time-Locked Contracts (HTLCs) are used in atomic swaps, allowing parties to exchange assets across different blockchains without trusting each other directly.
Understanding HTLC Atomic Swaps with Bob and Alice
Hash Time-Locked Contracts (HTLCs) are smart contracts used in atomic swaps, enabling two parties—let’s call them Bob and Alice—to exchange assets across different blockchains without the need for trust. HTLCs leverage cryptographic hash functions and time locks, ensuring that both parties either complete the swap or get their funds back.
Scenario: Alice and Bob Atomic Swap
Objective: Alice wants to swap her cryptocurrency on Blockchain A with Bob’s cryptocurrency on Blockchain B.
Step-by-Step Process
- Agreement on Terms:
- Alice and Bob agree on the amount, exchange rate, hash function, and a time lock (deadline for the swap).
2. Alice Creates an HTLC on Blockchain A:
- Alice generates a random secret value
S
. - Alice computes the hash
H(S)
of this secret. - Alice creates an HTLC on Blockchain A, locking her funds with these conditions:
- Condition 1: Bob can claim the funds if he provides the secret
S
that hashes toH(S)
before the time lock expires. - Condition 2: If Bob fails to provide
S
within the time limit, Alice can reclaim her funds.
- Condition 1: Bob can claim the funds if he provides the secret
3. Bob Creates a Corresponding HTLC on Blockchain B:
- Bob, after seeing the hash
H(S)
, creates his own HTLC on Blockchain B and locks his funds with these conditions:- Condition 1: Alice can claim the funds if she provides the secret
S
that matchesH(S)
before the time lock expires. - Condition 2: If Alice fails to provide
S
within the time limit, Bob can reclaim his funds.
- Condition 1: Alice can claim the funds if she provides the secret
4. Alice Claims Bob’s Funds on Blockchain B:
- Alice, who knows
S
, claims Bob’s funds by providing the secretS
in a transaction on Blockchain B.
5. Bob Sees the Secret S
:
- Since transactions on blockchains are public, Bob sees Alice’s claim transaction on Blockchain B, revealing the secret
S
.
6. Bob Claims Alice’s Funds on Blockchain A:
- Bob uses the revealed secret
S
to claim Alice’s funds from the HTLC on Blockchain A.
How Bob Sees the Secret
- Bob doesn’t know the secret
S
initially; he only knows the hashH(S)
. - When Alice claims the funds from Bob’s HTLC, she must reveal the secret
S
in the transaction. - This transaction publicly exposes
S
on the blockchain, allowing Bob to see and use it to complete his side of the swap.
The Pseudo Code
# Step 1: Alice creates a secret and computes its hash
alice_secret = generate_random_secret() # Alice's random secret 'S'
hash_of_secret = hash(alice_secret) # H(S) - Hash of the secret
# Step 2: Alice creates an HTLC contract on Blockchain A
alice_htlc = create_htlc(
recipient = "Bob", # Bob can claim the funds
hashlock = hash_of_secret, # Condition 1: Must provide S matching H(S)
timelock = 48 hours, # Condition 2: Must claim within 48 hours
amount = 10 coins # Funds locked by Alice
)
# Step 3: Bob creates a corresponding HTLC contract on Blockchain B
bob_htlc = create_htlc(
recipient = "Alice", # Alice can claim the funds
hashlock = hash_of_secret, # Condition 1: Must provide S matching H(S)
timelock = 24 hours, # Condition 2: Must claim within 24 hours
amount = 5 coins # Funds locked by Bob
)
# Step 4: Alice claims Bob's funds by revealing the secret on Blockchain B
if alice_htlc.verify_and_claim(alice_secret):
print("Alice claims Bob's funds using the secret")
# Step 5: Bob sees the secret and claims Alice's funds on Blockchain A
if bob_htlc.verify_and_claim(alice_secret):
print("Bob claims Alice's funds using the revealed secret")
# Step 6: Fallback conditions (if either party fails to claim)
if alice_htlc.time_expires():
alice_htlc.refund() # Alice reclaims her funds on Blockchain A
if bob_htlc.time_expires():
bob_htlc.refund() # Bob reclaims his funds on Blockchain B
Summary
Hash Time-Locked Contracts
HTLC atomic swaps are secure because the secret S
must be revealed by one party to complete the trade, ensuring that the swap either happens fully or not at all. This system of cryptographic commitments and public exposure ensures a trustless and fair exchange between parties.
Blockchain Transparency: Blockchains are designed to be transparent and auditable, so all transaction details, including revealed secrets, are publicly visible. This is essential to allow anyone, including Bob, to verify that the correct conditions were met