Using “Upsert” with Qdrant Vector Database
Upsert additional points with the next available index – add more vectors without recreating the collections. You’ll need to specify the next index, so here’s how to do it…
Check Qdrant out here :
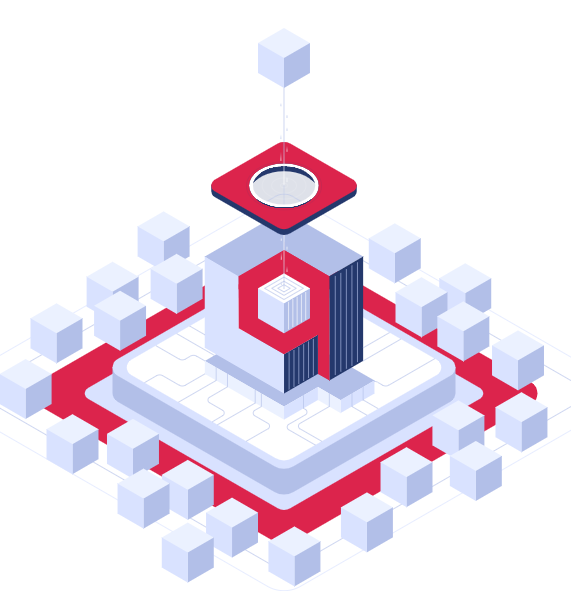
https://qdrant.tech/documentation/quick-start/
Do the imports and and create the “client”
Use client.get_collection() to retrieve the current status of the collection
This will give you the current total number of vectors. We’ll use that further on.
from qdrant_client.models import Distance, VectorParams
from qdrant_client import QdrantClient
# set up client
client = QdrantClient(host="localhost", port=6333)
coll_name="testx_collection"
collection_info = client.get_collection(collection_name=coll_name)
Get the current highest index
# get highest index
print(f"current vector count = ", collection_info.vectors_count)
try:
client.create_collection(
collection_name=coll_name,
vectors_config=VectorParams(size=100, distance=Distance.COSINE),
)
except:
pass
Upsert the additional points
# upsert additional points
import numpy as np
from qdrant_client.models import PointStruct
vectors = np.random.rand(100, 100)
client.upsert(
collection_name=coll_name,
points=[
PointStruct(
id=collection_info.vectors_count + idx,
vector=vector.tolist(),
payload={"color": "red", "rand_number": idx % 10}
)
for idx, vector in enumerate(vectors)
]
)
Check the number of vectors has increased
collection_info = client.get_collection(collection_name=coll_name)
print(f"new vector count = after upsert ", collection_info.vectors_count)