Working with dictionaries in Python
In this article we will look at OrderedDict and Counter
Use an OrderedDict to sort an existing dictionary such as the one below:
dict = {'bob': 340, 'jim': 939,
'sid': 152, 'baz': 27, 'foo': 32}
How to sort a Python dictionary
Enclose the dictionary with OrderedDict(sorted(dict.items())
Note: you can also add in “reverse” if you want the opposite sort order:
dict1 = OrderedDict(sorted(dict.items(), reverse=True))
print(dict1)
How to remove a key:value pair from a Python Dictionary
To remove a key value pair use pop – this will work even if the key value pair you pass to pop is absent:
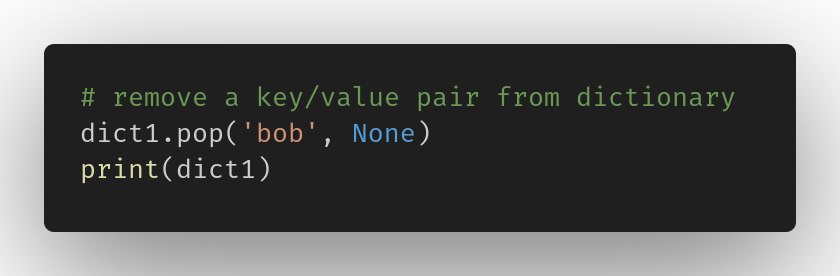
How to add a new key:value pair
Add a new key value pair in a Python dictionary:
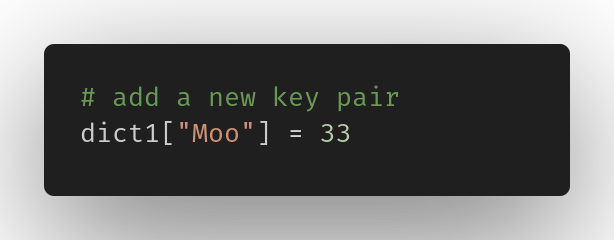
How to rename a key
Rename a key in a Python dictionary:
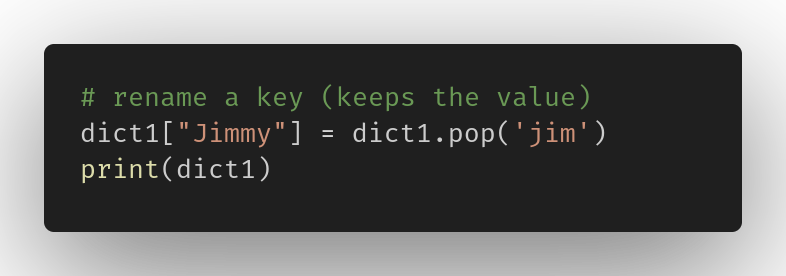
Capitalise each key in a dictionary:
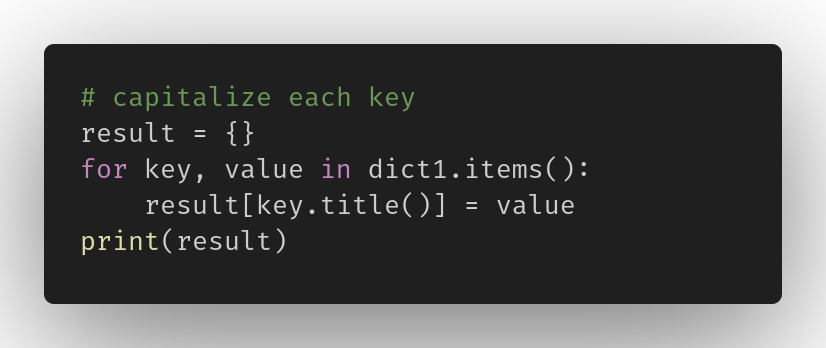
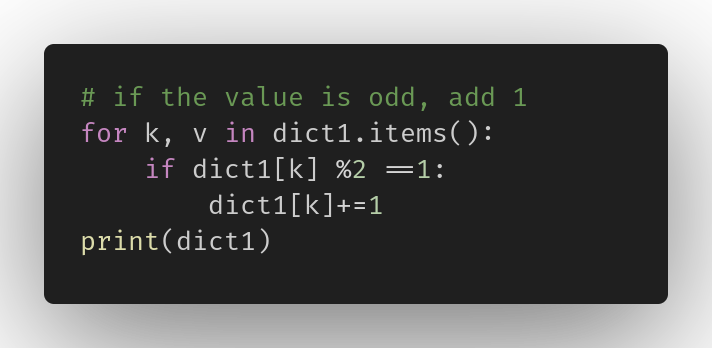
Buy me a coffee?
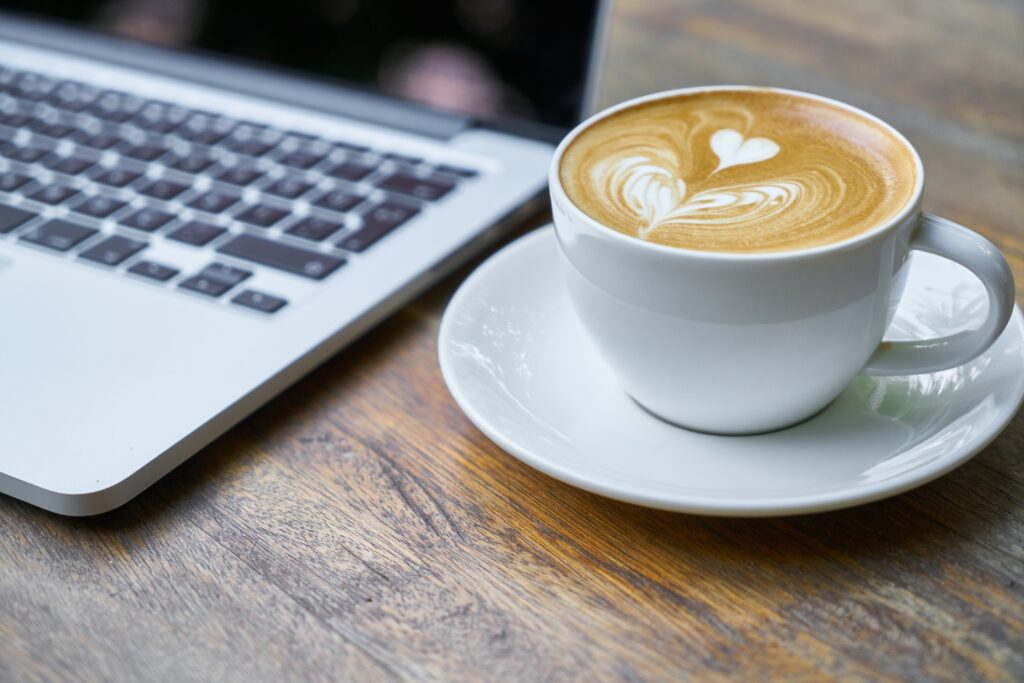
This site is hosted with https://webdock.io/en Fast Cloud VPS Hosting Flat fee all-inclusive VPS with a Free Control Panel if you are looking for fast reliable hosting then check them out!
OrderedDict
# Create sorted dictionary (sorted by key)
dict = {'bob': 340, 'jim': 939,
'sid': 152, 'baz': 27, 'foo': 32}
dict1 = OrderedDict(sorted(dict.items(), reverse=True))
print(dict1)
# remove a key/value pair from dictionary
dict1.pop('bob', None)
print(dict1)
# add a new key pair
dict1["Moo"] = 33
# rename a key (keeps the value)
dict1["Jimmy"] = dict1.pop('jim')
print(dict1)
# capitalize each key
result = {}
for key, value in dict1.items():
result[key.title()] = value
print(result)
# if the value is odd, add 1
for k, v in dict1.items():
if dict1[k] %2 ==1:
dict1[k]+=1
print(dict1)
Counter
from collections import Counter
ls = ['a','b','b','c','c','c']
from collections import Counter
Counter(ls)
Counter({'a': 1, 'b': 2, 'c': 3})
Working with dictionaries : Video
Check out the code to extract “.co.uk” email address values from JSON and test the functions using pytest and the fixtures decorator.