The Iterator Trait
when implementing the Iterator
trait in Rust, your struct usually needs some form of state to keep track of the iteration’s progress. Often, this is achieved by including a counter or index field in your struct.
Also, check out :
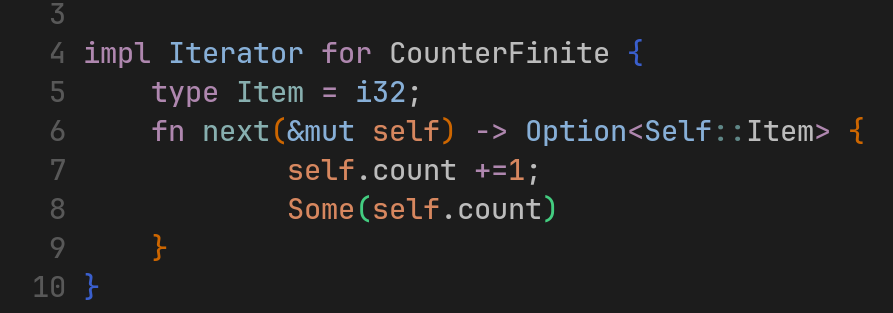
impl Iterator for
Here’s a basic example of how to implement an iterator in Rust with a counter:
struct Counter {
current: u32,
max: u32,
}
impl Counter {
fn new(max: u32) -> Self {
Counter { current: 0, max }
}
}
impl Iterator for Counter {
type Item = u32;
fn next(&mut self) -> Option<Self::Item> {
if self.current < self.max {
let result = self.current;
self.current += 1;
Some(result)
} else {
None
}
}
}
fn main() {
let mut counter = Counter::new(5);
while let Some(value) = counter.next() {
println!("{}", value);
}
}
Yes, when implementing the Iterator
trait in Rust, your struct usually needs some form of state to keep track of the iteration’s progress. Often, this is achieved by including a counter or index field in your struct.
Here’s a basic example of how to implement an iterator in Rust with a counter:
struct Counter {
current: u32,
max: u32,
}
impl Counter {
fn new(max: u32) -> Self {
Counter { current: 0, max }
}
}
impl Iterator for Counter {
type Item = u32;
fn next(&mut self) -> Option<Self::Item> {
if self.current < self.max {
let result = self.current;
self.current += 1;
Some(result)
} else {
None
}
}
}
fn main() {
let mut counter = Counter::new(5);
while let Some(value) = counter.next() {
println!("{}", value);
}
}
Explanation
- Struct with a Counter:
- The
Counter
struct has acurrent
field that keeps track of the current iteration index and amax
field that defines when the iteration should stop.
- Implementing
Iterator
:
- The
next
method is where the logic for producing the next item resides. Ifcurrent
is less thanmax
, it returns the current value and then increments the counter. Ifcurrent
is equal to or greater thanmax
, it returnsNone
, signaling the end of the iteration.
- Using the Iterator:
- The
main
function demonstrates how to use theCounter
iterator, printing values from0
to4
.
In this example, the current
field acts as the counter that determines where the iterator is in its sequence.
Note the use of mut in the next function? It's required because the function modified the state of the iterator.
The next function will always return Option<Self::Item> as well
Challenge!
Implement an iterator that generates only even numbers up to a specified maximum
Here’s a cut cat. If you want the answer scroll past it!
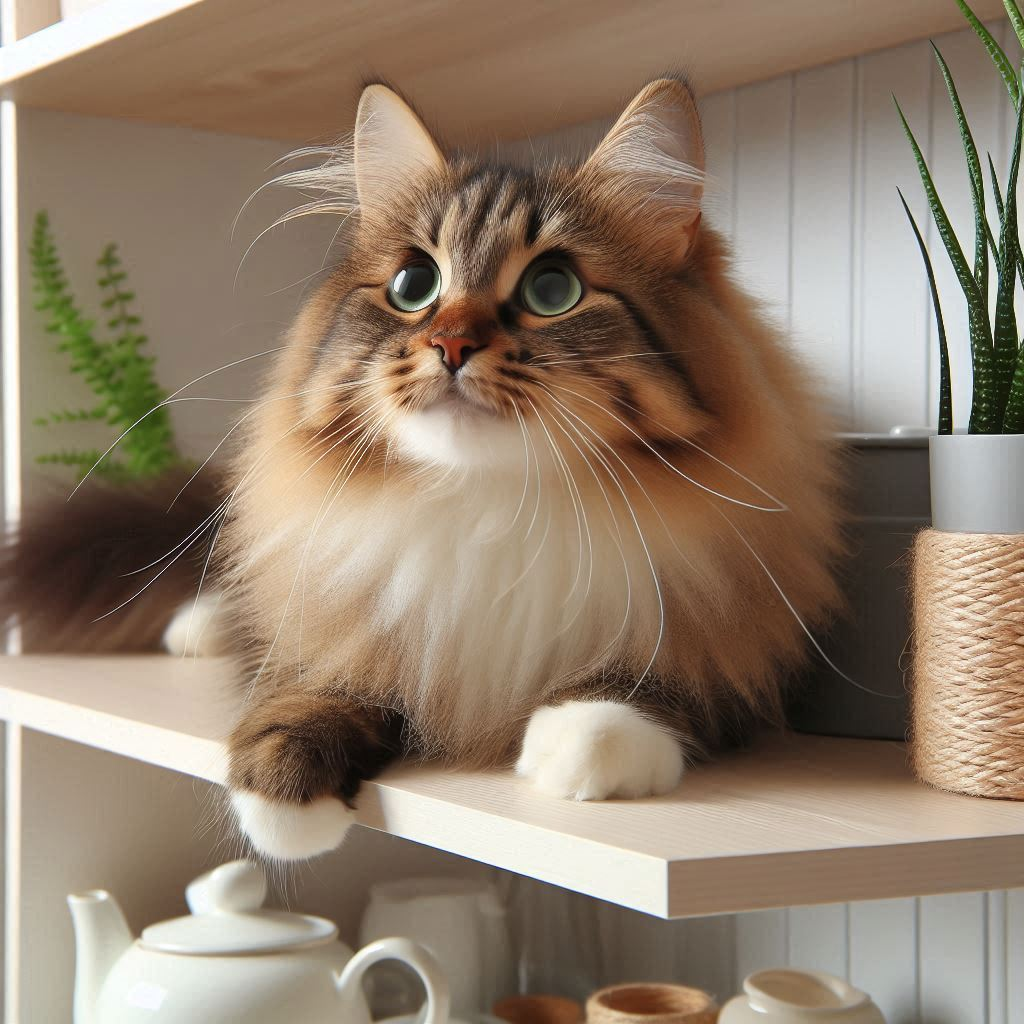
Solution
struct EvenCounter {
current: u32,
max: u32,
}
impl EvenCounter {
fn new(max: u32) -> Self {
EvenCounter { current: 0, max }
}
}
impl Iterator for EvenCounter {
type Item = u32;
fn next(&mut self) -> Option<Self::Item> {
if self.current <= self.max {
let result = self.current;
self.current += 2; // Move to the next even number
Some(result)
} else {
None
}
}
}
fn main() {
let mut even_counter = EvenCounter::new(10);
while let Some(value) = even_counter.next() {
println!("{}", value);
}
}