Musl – static linking
I have a chat with chatGPT about Musl, static linking, libc.so.6 and dereferencing smart pointers…here is the conversation!
sudo apt-get install musl-tools
is it possible to make a rust program that prints it’s main.rs and shows what variables live on the stack and which live on the heap?
In Rust, it’s not straightforward to inspect the stack and heap at runtime due to its emphasis on memory safety and ownership. Rust’s ownership system ensures memory safety at compile-time, and it avoids exposing low-level details about memory management during runtime.
However, you can use certain tools and techniques to get insights into memory usage:
- Debugging Macros: Rust has built-in debugging macros like
dbg!
that can help you print the values of variables. While these won’t give you detailed information about the stack and heap, they can be useful for inspecting variables during execution.rustCopy codefn main() { let x = 42; dbg!(x); }
- Profiling Tools: Tools like
perf
orgdb
(Gnu Debugger) can provide information about program execution, including memory usage. However, these tools are not Rust-specific and may not give you a Rust-centric view of your program. - Heap Profiling: You can use external tools like
heaptrack
orvalgrind
to profile heap usage. These tools are not Rust-specific either but can provide insights into memory allocation and deallocation. - Memory Profiling with
valgrind
andmassif
: You can usevalgrind
with themassif
tool to profile memory usage. This may not give you Rust-specific details, but it can help you understand how your program utilizes memory.bashCopy codevalgrind --tool=massif ./your_rust_program
- Rust Memory Profiling Tools: There are some Rust-specific tools like
cargo-flamegraph
andcargo-profiling
that you can use for profiling. These tools often focus on performance profiling rather than detailed memory analysis.
Keep in mind that while these tools can provide useful information, the design of Rust discourages direct manipulation of the stack and heap at runtime. Rust’s ownership system and borrow checker are designed to ensure memory safety and prevent common memory-related bugs, making it less common for developers to directly manage memory during program execution.
Valgrind is a tool for memory debugging, memory leak detection, and profiling, and its output typically includes information about memory errors and allocations.
Here’s an example of what Valgrind output might look like:
==1234== Memcheck, a memory error detector ==1234== Copyright (C) 2002-2017, and GNU GPL'd, by Julian Seward et al. ==1234== Using Valgrind-3.15.0 and LibVEX; rerun with -h for copyright info ==1234== Command: ./your_program ==1234== ==1234== Conditional jump or move depends on uninitialised value(s) ==1234== at 0x4E7AB80: strlen (vg_replace_strmem.c:454) ==1234== by 0x40068E: main (example.c:9) ==1234== ==1234== ==1234== HEAP SUMMARY: ==1234== in use at exit: 72 bytes in 1 blocks ==1234== total heap usage: 1 allocs, 0 frees, 72 bytes allocated ==1234== ==1234== LEAK SUMMARY: ==1234== definitely lost: 72 bytes in 1 blocks ==1234== indirectly lost: 0 bytes in 0 blocks ==1234== possibly lost: 0 bytes in 0 blocks ==1234== still reachable: 0 bytes in 0 blocks ==1234== suppressed: 0 bytes in 0 blocks ==1234== Rerun with --leak-check=full to see details of leaked memory ==1234== ==1234== For lists of detected and suppressed errors, rerun with: -s ==1234== ERROR SUMMARY: 1 errors from 1 contexts (suppressed: 0 from 0)
This output indicates a memory error related to using an uninitialized value and also provides information about heap memory usage and potential memory leaks.
โฏ valgrind –tool=massif ./mofo_crap ==32578== Massif, a heap profiler ==32578== Copyright (C) 2003-2017, and GNU GPL’d, by Nicholas Nethercote ==32578== Using Valgrind-3.18.1 and LibVEX; rerun with -h for copyright info ==32578== Command: ./mofo_crap ==32578== Rooms: 2, Age 2, Roof tiles, Parking true, Description None 100%|โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ| 100/100 [00:03<00:00, 31.98it/s]==32578==
The output is from Valgrind’s Massif tool, which is a heap profiler. The output doesn’t contain any obvious memory errors or leaks, but it provides information about heap memory usage over time.
The important part of the output is typically towards the end, where Massif summarizes the heap usage. Unfortunately, the output you’ve posted doesn’t include that summary. Usually, it shows information like total heap usage, peak heap usage, and the number of allocations.
If you can provide the summary part of the Massif output, I might be able to help you interpret it. Look for lines that start with “heap summary” or something similar. It will give you an idea of how much memory your program is using and where the memory is allocated. Additionally, if there are any memory leaks, Massif will typically report that as well.
This is my source code, does that help??
#![allow(unused)]
use kdam::tqdm;
#[derive(Debug)]
struct House {
rooms: u8,
age: u16,
roof: String,
parking: bool,
description: Option<String>,
}
impl House {
fn new() -> Self {
House {
rooms: 2,
age: 2,
roof: "tiles".to_string(),
parking: true,
description: None,
}
}
}
impl Into<String> for House {
fn into(self) -> String {
format!(
"Rooms: {}, Age {}, Roof {}, Parking {}, Description {:?}",
self.rooms, self.age, self.roof, self.parking, self.description
)
}
}
fn main() {
let my_house = House::new();
let my_house_string: String = my_house.into();
println!("{}", my_house_string);
for _ in tqdm!(0..100) {
std::thread::sleep(std::time::Duration::from_secs_f32(0.03));
}
}
The source code you provided seems to be a Rust program that defines a House
struct and then uses it to create an instance of a house. It also implements the Into<String>
trait for the House
struct to convert it into a string representation. Finally, it prints the string representation and runs a loop using the tqdm!
macro, which is often used for creating progress bars.
The code itself doesn’t seem to have any obvious memory issues, and Valgrind’s Massif output doesn’t show any memory errors or leaks. The Massif tool primarily focuses on heap memory, and your program appears to be quite straightforward in terms of memory management.
If you have specific questions or concerns related to memory usage or if there’s a particular aspect you would like me to focus on, please let me know. Otherwise, based on the provided information, your Rust program seems well-structured and memory-safe.
You
Give me code to add, so that valgrind sees some heap related info
ChatGPT
To see meaningful heap-related information in Valgrind’s Massif output, you need to dynamically allocate memory using functions like Box::new
or Vec::new
. Here’s a modified version of your code that includes dynamic memory allocation:
#![allow(unused)]
use kdam::tqdm;
#[derive(Debug)]
struct House {
rooms: u8,
age: u16,
roof: String,
parking: bool,
description: Option<String>,
}
impl House {
fn new() -> Self {
House {
rooms: 2,
age: 2,
roof: "tiles".to_string(),
parking: true,
description: None,
}
}
fn with_description(description: &str) -> Self {
House {
rooms: 2,
age: 2,
roof: "tiles".to_string(),
parking: true,
description: Some(description.to_string()),
}
}
}
impl Into<String> for House {
fn into(self) -> String {
format!(
"Rooms: {}, Age {}, Roof {}, Parking {}, Description {:?}",
self.rooms, self.age, self.roof, self.parking, self.description
)
}
}
fn main() {
let my_house = Box::new(House::new()); // Dynamic allocation
let my_house_with_desc = Box::new(House::with_description("A cozy home")); // Dynamic allocation
let my_house_string: String = (*my_house).into();
let my_house_with_desc_string: String = (*my_house_with_desc).into();
println!("{}", my_house_string);
println!("{}", my_house_with_desc_string);
for _ in tqdm!(0..100) {
std::thread::sleep(std::time::Duration::from_secs_f32(0.03));
}
}
In this modified version, I’ve added dynamic allocation using Box::new
for two instances of House
. This should result in heap allocations that Valgrind’s Massif tool can track. When you run this code with Valgrind, you should see heap-related information in the Massif output.
You
explain this : why is there a “*” before my_house ?
ChatGPT
The *
symbol before my_house
is the dereference operator in Rust. It is used to obtain the value pointed to by a reference or a box. In this case, my_house
is a Box
, which is a smart pointer that owns a heap-allocated value.
When you allocate memory on the heap using Box::new
, it returns a box (a pointer) to the allocated value. To access the actual value, you need to dereference the box. The *
operator is used for this purpose.
In the line let my_house_string: String = (*my_house).into();
, (*my_house)
dereferences the Box<House>
to obtain the actual House
value, and then the into
method is called on that value to convert it into a String
.
Here’s a breakdown of what’s happening in that line:
*my_house
: Dereferences theBox<House>
to get theHouse
value..into()
: Converts theHouse
value into aString
using theInto<String>
trait.
Without the *
operator, you would be trying to call the into
method directly on the Box
, which wouldn’t work because Box
doesn’t implement Into<String>
. You need to dereference the Box
to access the value it points to.
You
tell me about “libc.so.6″ChatGPT
ChatGPT
libc.so.6
is a dynamic link library (shared object) on Linux systems that provides a standard C library. The name libc.so.6
follows the naming convention for shared libraries on Unix-like systems. The “libc” part stands for “C library,” and the “so” stands for “shared object,” indicating that it is a dynamically linked library.
Here’s a breakdown of the name:
- libc: C Library
- .so: Shared Object (Dynamic Library)
- .6: Version number (the actual version number may vary)
The libc.so.6
library is a crucial component of a Linux system, providing a set of standard functions that are fundamental for the execution of C programs. These functions include basic input/output operations, memory allocation and deallocation, string manipulation, mathematical functions, and various other utilities.
The version number (6
in libc.so.6
) is specific to the implementation and version of the GNU C Library (glibc) on the system. Different versions of glibc may introduce new features, improvements, or bug fixes.
When a program is compiled on a Linux system, it is linked to libc.so.6
by default. During program execution, the dynamic linker/loader (ld.so
or ld-linux.so
) is responsible for loading the necessary shared libraries, including libc.so.6
, into the process’s address space.
If you encounter issues related to libc.so.6
during program execution or linking, it often involves library compatibility or missing dependencies. Ensuring that the required version of the C library is available on the system is crucial for the proper functioning of C programs on Linux.
You
does rust compiler include it in compiled binary?ChatGPT
ChatGPT
No, the Rust compiler (rustc
) does not include libc.so.6
in the compiled binary. Rust programs are compiled to machine code and, by default, dynamically link to the system’s C library (libc
). The C library provides the standard C functions and system calls that are used by Rust programs for various operations.
During the execution of a Rust program, the dynamic linker/loader on the target system is responsible for locating and loading the necessary shared libraries, including libc.so.6
, into the process’s address space.
If you inspect a compiled Rust binary, you won’t find a copy of libc.so.6
bundled with it. Rust binaries depend on the presence of the C library on the target system. When you distribute a Rust program, you typically assume that the target system has the required version of libc.so.6
or an equivalent C library.
If you want to create a standalone, statically linked binary that includes the necessary libraries, you can use the musl
target with the Rust compiler. The musl
target allows you to statically link the C library into your Rust binary. However, this approach increases the size of the binary and may have licensing considerations.
You
tell me more about muslChatGPT
ChatGPT
Musl is a lightweight, fast, and standard-conformant C library implementation designed for static linking. It is an alternative to the more common GNU C Library (glibc) that is often used in many Linux distributions. Musl is known for its focus on simplicity, efficiency, and small footprint.
Here are some key points about Musl:
- Static Linking: Musl is particularly well-suited for static linking, where the C library is directly included in the executable, resulting in standalone and portable binaries. This can be beneficial for creating small, self-contained executables that do not rely on external shared libraries.
- Size and Efficiency: Musl is designed to be lightweight, and the binaries produced with Musl tend to be smaller compared to those linked with glibc. This is advantageous for resource-constrained environments and scenarios where minimizing the binary size is crucial.
- Standard Conformance: Musl aims to adhere strictly to standards such as ISO C and POSIX. Its design philosophy is to provide a clean and minimal implementation of these standards while avoiding unnecessary extensions.
- Compatibility: Musl is compatible with a wide range of applications and libraries. It is intended to be a drop-in replacement for glibc in many cases, making it possible to use Musl without significant code changes.
- License: Musl is released under the MIT license, which is a permissive open-source license. This makes Musl suitable for use in various projects, including both open-source and proprietary software.
- Use with Rust: Rust, being a systems programming language, can be configured to target the Musl C library when compiling for specific platforms. This allows Rust developers to create statically linked executables with reduced dependencies.
To use Musl with Rust, you need to set the target architecture to one that supports Musl. For example, the x86_64-unknown-linux-musl
target is commonly used for 64-bit Linux systems with Musl.
cargo build --target=x86_64-unknown-linux-musl
Keep in mind that while Musl is a powerful and lightweight choice for certain use cases, the selection of C library depends on your specific requirements and the target environment of your applications.
You
Give me an idea of the binary size with and without musl being used
ChatGPT
The binary size can vary significantly based on factors such as the complexity of your code, the dependencies you use, and the optimizations applied during compilation. Additionally, the use of Musl or glibc, static or dynamic linking, and other compilation options can also impact the size of the resulting binary.
To provide a rough idea, here’s a basic comparison using a simple “Hello, World!” program in Rust. The examples assume static linking with Musl and dynamic linking with glibc.
- Hello World with Musl (Static Linking):
# Build with Musl
cargo build --release --target=x86_64-unknown-linux-musl
# Check the size of the resulting binary
ls -lh target/x86_64-unknown-linux-musl/release/your_program
- Hello World with glibc (Dynamic Linking):
# Build with glibc
cargo build --release
# Check the size of the resulting binary
ls -lh target/release/your_program
The binary size comparison will depend on various factors, but generally, you may observe that the binary produced with Musl is smaller due to static linking. Keep in mind that the actual impact on binary size can vary based on your application’s complexity and dependencies.
Additionally, if you want to further reduce the binary size, you can explore techniques like optimizing for size during compilation (-C opt-level=s
), stripping debug information (-C strip=symbols
), or manually excluding unnecessary features and dependencies.
Remember that while minimizing binary size is often desirable, the choice between static and dynamic linking, as well as the selection of the C library, should be based on your specific use case and deployment requirements.
You
this gives 100’s of errors, how can I check my linux pc? cargo build –release –target=x86_64-unknown-linux-muslChatGPT
ChatGPT
Building a Rust project with the x86_64-unknown-linux-musl
target requires the appropriate target toolchain to be installed. Additionally, building with Musl may require you to install the Musl development libraries on your system.
Here are steps to check and set up your Linux system for building with the x86_64-unknown-linux-musl
target:
- Install the Musl Development Libraries: Musl development libraries need to be installed on your system. The exact package name might vary depending on your Linux distribution.
- On Debian/Ubuntu-based systems:bashCopy code
sudo apt-get install musl-tools
- On Fedora:bashCopy code
sudo dnf install musl
- On Alpine Linux:bashCopy code
apk add musl-dev
- On Debian/Ubuntu-based systems:bashCopy code
- Install the
x86_64-unknown-linux-musl
Target: Ensure that the target is available for your Rust toolchain. You can userustup
to install it.bashCopy coderustup target add x86_64-unknown-linux-musl
- Build the Project: Once you have the Musl development libraries and the target installed, you can build your Rust project with the Musl
cargo build --release --target=x86_64-unknown-linux-musl
If you encounter errors during the build, carefully read the error messages. Common issues might include missing dependencies or incompatible code. Ensure that your project’s dependencies are compatible with Musl and don’t rely on features specific to glibc.Additionally, some crates may require adjustments in theCargo.toml
file to specify that the Musl target should be used. For example:tomlCopy code[target.x86_64-unknown-linux-musl.dependencies] # Specify Musl-compatible dependencies here
- Check the Binary Size: After a successful build, you can check the size of the resulting binary.bashCopy code
ls -lh target/x86_64-unknown-linux-musl/release/your_program
This will show you the size of the binary.
You
and will this musl version now run without the client needing libc.so6 ?ChatGPT
ChatGPT
Yes, if you successfully build your Rust program with the x86_64-unknown-linux-musl
target and static linking against Musl, the resulting binary should be more self-contained and not require the client to have libc.so.6
available on their system.
Musl is designed for static linking, and when you use it as the target, the resulting binary includes the Musl library and is less dependent on external shared libraries like libc.so.6
. This can be beneficial when you want to distribute a binary that works across different Linux distributions without worrying about specific versions of the C library.
Keep in mind that, while Musl provides great portability, there might still be dependencies in your Rust project that rely on system-specific features. You need to ensure that all dependencies are compatible with the Musl target.
You can test the portability of your Musl-linked binary by running it on a clean Linux system or in a minimal Docker container. If it runs successfully without requiring additional libraries, then it’s a good indication that the binary is self-contained.
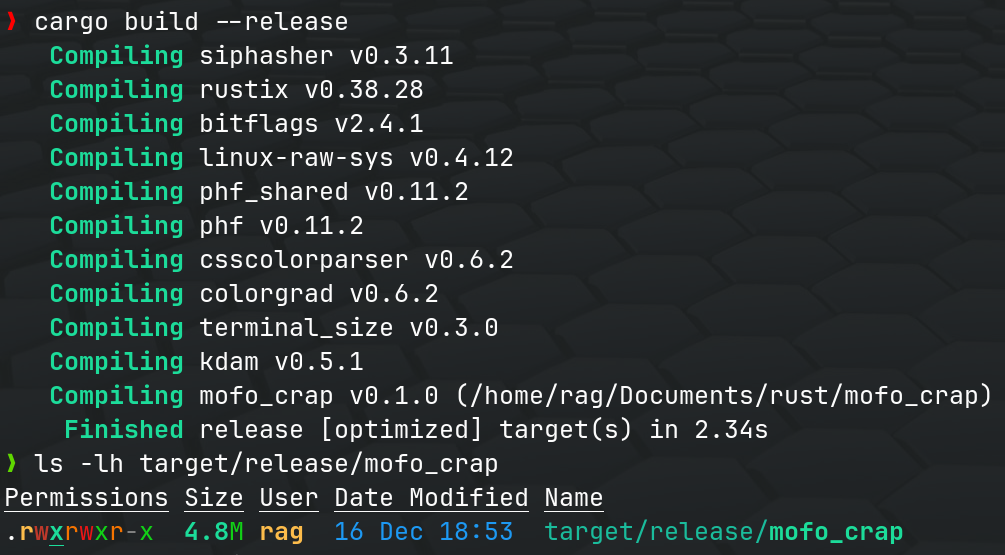
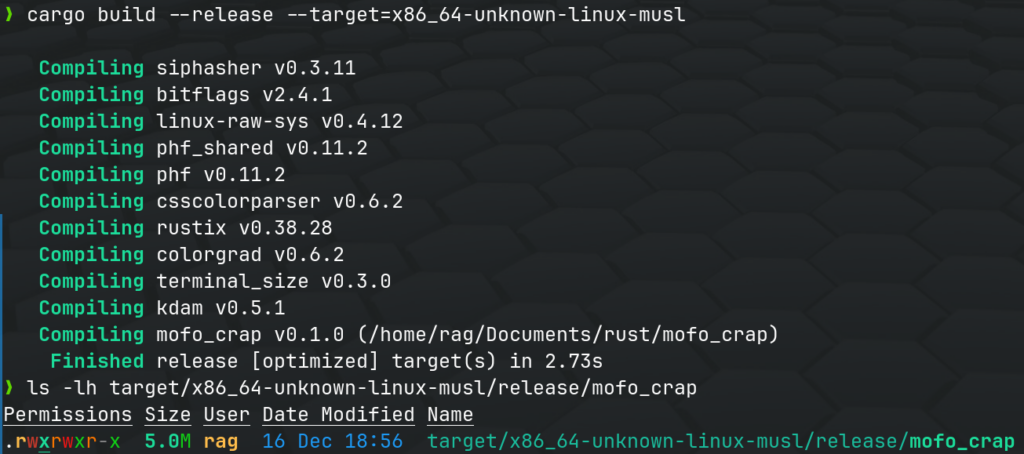

Is libc.so.6 the only file that musl includes?
No, when you statically link a Rust program with Musl, the resulting binary includes more than just libc.so.6
. Musl is a C library implementation, and when statically linked, it becomes part of the executable. Additionally, Musl provides its own set of headers and other components that are necessary for the program to function correctly.
Here’s a general overview of what happens when you statically link with Musl:
- Musl C Library:
Musl provides its own implementation of the C library, which includes standard C functions and system calls. This library is statically linked into the executable, so the resulting binary doesn’t depend on an externallibc.so.6
file. - Static Linking:
Statically linking with Musl means that the Rust compiler includes Musl’s functionality directly in the executable. This results in a larger binary size because it contains everything needed for the program to run, including the C library. - Headers and Components:
In addition to the C library itself, Musl includes headers and other components necessary for compilation and linking. These components help define the interface between your Rust code and the underlying operating system.
When you distribute a Rust binary built with Musl, the binary is expected to be more self-contained and less dependent on external shared libraries compared to a binary linked with glibc dynamically. This can improve portability across different Linux distributions. However, keep in mind that Musl and glibc are different C library implementations, and there may be subtle differences between them, so testing on the target platform is essential.