Understanding Soroban Smart Contract Naming Conventions
When working with Soroban smart contracts, naming conventions are not just conventions—they’re built into the system, enforced by the Soroban SDK. This article explains how contract struct names and their functions translate into the client types and methods, ensuring seamless development. Blah blah blah.
What does that mean? I’ll show you, it means you get a free “builder” when you come to write a test…..
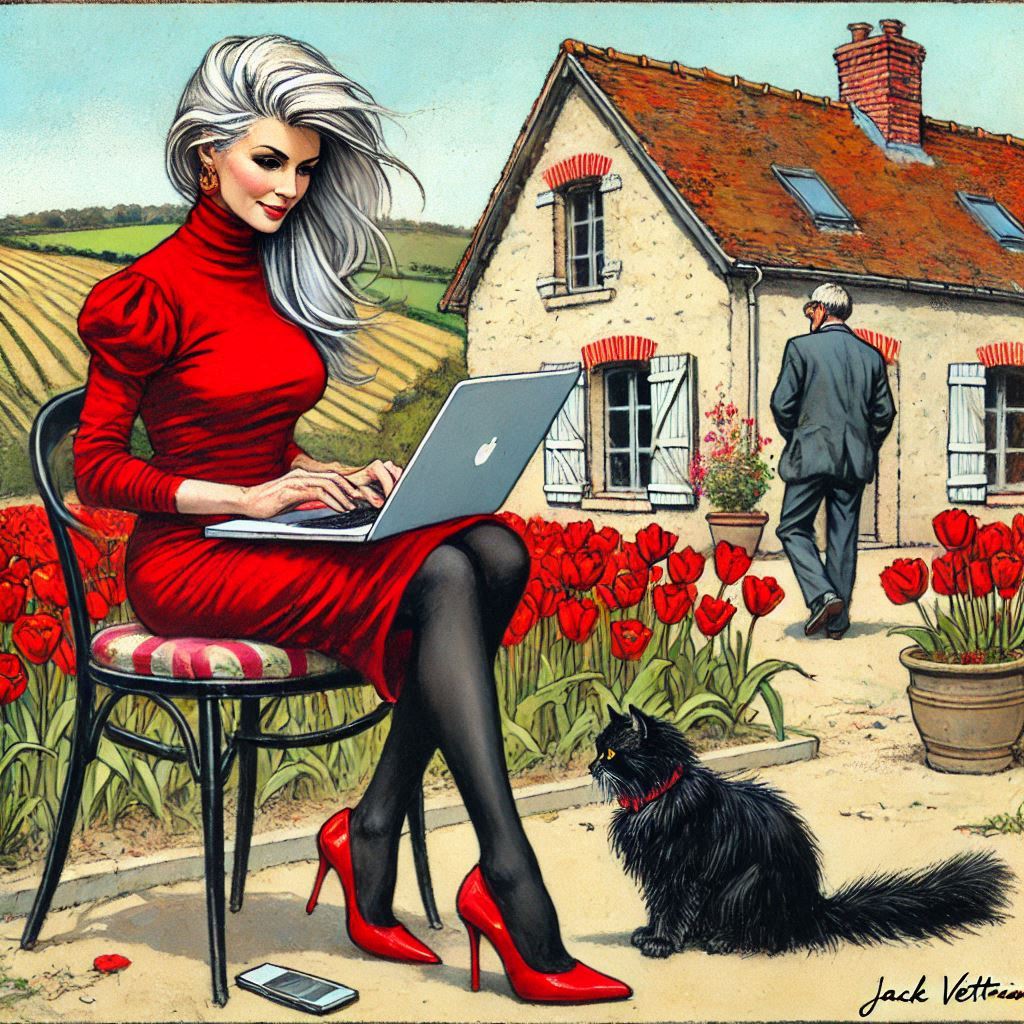
Note : The contract ID is represented as a hash derived from specific inputs. This ensures that it is unique and immutable, making it a reliable reference point for identifying a deployed smart contract.
Struct and Implementation Basics
A Soroban smart contract typically starts with a struct that represents the contract. For example:
pub struct Mofo;
The behavior of the contract is defined in an impl
block annotated with #[contractimpl]
.
#[contractimpl]
impl Mofo {
pub fn do_something(&self) {
// Logic here
}
}
How Client Types Are Generated
The #[contractimpl]
macro automatically generates a client type for the contract, appending Client
to the struct name. In this example, the client type will be:
MofoClient
All public functions in the #[contractimpl]
block are exposed as methods on the generated client. For the do_something
function, you can call it like this:
let client = MofoClient::new(&env, &contract_id);
client.do_something();
Key Rules to Remember
- Client Type Name: The client is named by appending
Client
to your contract struct.Mofo
→MofoClient
- Function Names: Public functions in the
#[contractimpl]
block are directly mirrored as methods in the client.do_something
→client.do_something()
Customizing Names
You cannot directly rename the generated client type or its functions, as these are determined by the macro. However, the struct name indirectly controls the client name.
For example:
- Struct:
MyCoolContract
→ Client:MyCoolContractClient
The client in the context of Soroban smart contracts refers to a generated helper type that provides an interface for interacting with the contract from external code, such as a test or production environment. It acts as a wrapper around the contract, enabling communication with it on the blockchain.
What Does the Client Do?
- Interacts with the Contract: The client allows you to call contract methods (such as
increment
ordo_something
) after the contract has been deployed to the blockchain. It acts as the bridge to send requests to the contract and interact with its functions. - Convenience: Instead of directly manipulating the contract’s raw data or writing out complicated blockchain interaction code, the client simplifies invoking the contract’s functions. It abstracts the complexity involved in interacting with the blockchain.
- Registers the Contract: The client helps in registering the contract with the environment (
Env
) and ensures that the contract’s functions are accessible via easy-to-use methods. For instance, it might look something like this in code:let client = MofoClient::new(&env, &contract_id); client.do_something();
Is the Client Necessary?
- In Tests: Yes, the client is often necessary in a test environment. It allows you to interact with your contract during testing by sending requests to the contract and verifying its behavior in a controlled, simulated environment.
- In Production: While the client is essential for tests or simulating blockchain interactions, in a production environment, the client itself may not be required in the same way. Instead, external systems (like your frontend or API) can directly interact with the blockchain through client libraries or APIs like the Soroban API, which communicates with deployed contracts.
Is It Just for This Example?
No, the client is a core part of the Soroban SDK’s design for interacting with smart contracts. It’s not just used for this specific example; it’s used in any scenario where you need to interact with a deployed contract—whether that’s for testing, calling functions from another program, or integrating with a larger application.
In short, the client is an essential tool for interacting with Soroban smart contracts, providing an easy-to-use API for invoking contract methods, but its necessity may vary depending on whether you are in a test, production, or interaction scenario.
Conclusion
Understanding these conventions ensures your Soroban development is intuitive and consistent. The automatic generation of client types and methods makes testing and interacting with contracts a streamlined experience.
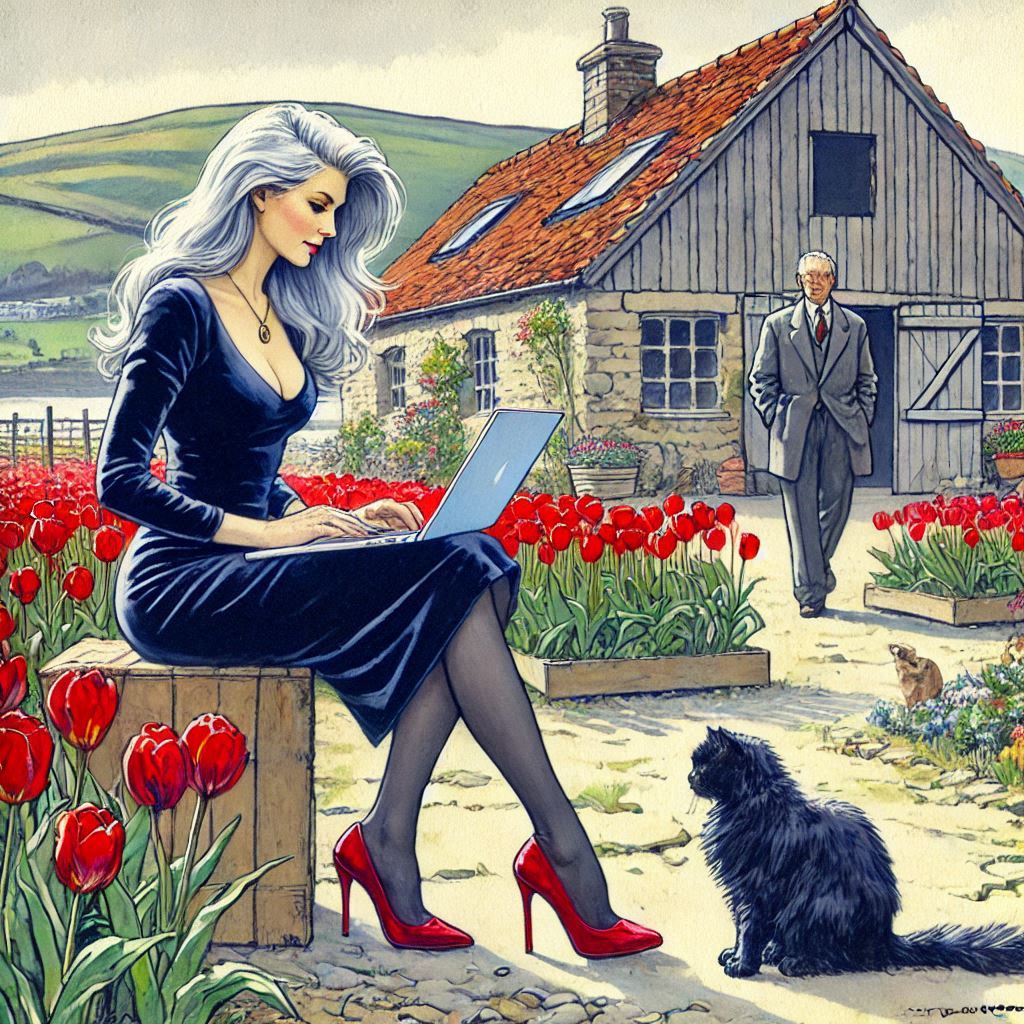