Ruff – Python Linter written in Rust
To use Ruff we need to consider the configuration for pyproject.toml
with a focus on how to set it up for Ruff.
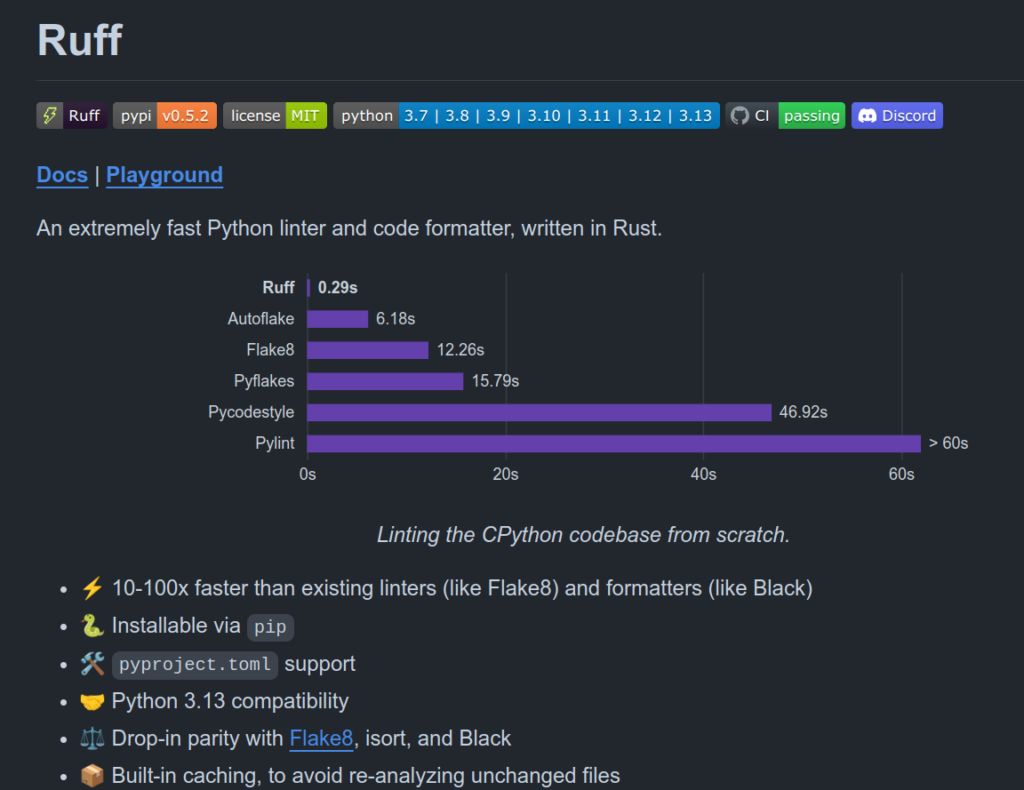
What is pyproject.toml
?
pyproject.toml
is a configuration file used by various Python tools to read project-specific settings. It is designed to provide a standardized way for specifying build and configuration settings for Python projects.
Setting Up pyproject.toml
for Ruff
Here’s how you can configure pyproject.toml
to use Ruff as your linter, with the correct structure to avoid any deprecation warnings.
Example pyproject.toml
for Ruff
[tool.ruff]
line-length = 88
[tool.ruff.lint]
select = ["E", "F", "W", "C90"]
ignore = ["E501"]
select = [“E”, “F”, “W”, “C90”] # Codes to be checked ignore = [“E501”] # Codes to be ignored
Explanation of Each Section
[tool.ruff]
: This section contains general configuration settings for Ruff. Here, you can specify the maximum line length or other general options.line-length = 88
: This sets the maximum allowed length of lines in your code to 88 characters.[tool.ruff.lint]
: This section is specifically for linting rules.select = ["E", "F", "W", "C90"]
: These are the linting rules Ruff will check for. You can specify various error, warning, and convention codes here.ignore = ["E501"]
: These are the linting rules Ruff will ignore. In this case,E501
typically corresponds to line length warnings, which are being ignored.
The Ruff linter configuration specifies the categories of linting rules that Ruff should check. Here’s a detailed explanation of what each code in select = ["E", "F", "W", "C90"]
represents:
Linting Categories
- E – Error:
- E codes generally represent errors that are detected by the linter. These errors are typically syntactical issues or potential bugs that violate the Python language rules or best practices.
- Example:
E231
– missing whitespace after a comma, semicolon, or colon.
- F – Pyflakes:
- F codes are from Pyflakes, a tool for checking Python programs for errors. It mainly focuses on logical errors such as using undefined variables or importing unused modules.
- Example:
F401
– module imported but unused.
- W – Warning:
- W codes represent warnings that indicate potential issues in the code. These might not be errors, but they highlight practices that could lead to errors or make the code less readable.
- Example:
W291
– trailing whitespace.
- C90 – McCabe Complexity:
- C90 codes come from the McCabe complexity checker. McCabe complexity is a measure of the complexity of a piece of code. High complexity can make code harder to understand and maintain.
- Example:
C901
– function is too complex.
Why Use These Categories?
- Errors (E): Catching and fixing errors helps in maintaining syntactically correct and bug-free code.
- Pyflakes (F): Ensuring logical correctness by catching undefined names and unused imports helps in avoiding runtime errors.
- Warnings (W): Addressing warnings improves code quality and readability.
- McCabe Complexity (C90): Monitoring code complexity helps in maintaining readable and maintainable code, which is easier to test and debug.
Linitng rules to check ignore = [“E501”] # Ignore specific linting rules (e.g., line length warnings)
Example Usage
Suppose you have a Python script example.py
with some issues:
import os, sys # This will trigger F401 (multiple imports in one line)
def example_function(): # This will trigger C901 if too complex
a = 1
b = 2
print(a+b)
example_function()
When you run Ruff:
ruff check example.py
Ruff will check the script for issues in the specified categories (E
, F
, W
, C90
) and report them.
Summary
The select
option in Ruff’s configuration allows you to specify which categories of linting rules to check. By using select = ["E", "F", "W", "C90"]
, you instruct Ruff to check for errors, Pyflakes issues, warnings, and McCabe complexity. This helps ensure that your code is syntactically correct, logically sound, readable, and maintainable.
How to Use pyproject.toml
with Ruff
- Create the
pyproject.toml
File:
If you don’t already have apyproject.toml
file in the root of your project, create one and add the above configuration. - Run Ruff:
Execute Ruff to check your files with the following command:
ruff check my_script.py
Ruff will read the settings from pyproject.toml
and apply the specified linting rules.
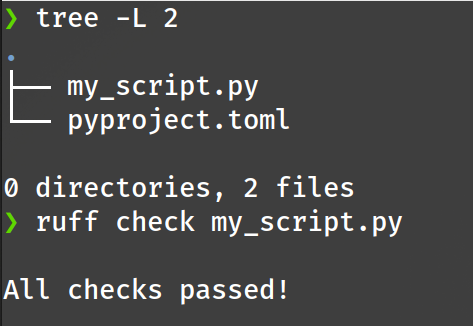
Ruff checks passed!
Summary
The pyproject.toml
file is used to configure various tools in a Python project. For Ruff, it helps to define linting rules and general settings. By structuring the file correctly, you can avoid deprecation warnings and ensure Ruff works as expected. The provided configuration sets the maximum line length and specifies which linting rules to check or ignore.