When or why should I use a Mutex over an RwLock?
TL;DR – use the “R” in RwLock as “R” for READ – i.e when there are lots of reads/Read mostly, use RwLock
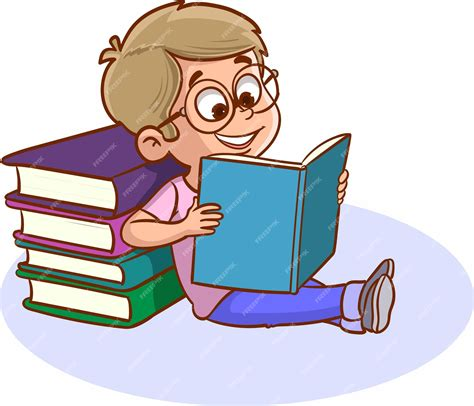
Choosing between a Mutex
and an RwLock
in Rust depends on the nature of the access patterns and the trade-offs you’re willing to make in your program’s concurrency model. Here’s a detailed breakdown of when and why to use each:
a
Mutex
does not distinguish between readers or writers that acquire the lock, therefore blocking any threads waiting for the lock to become available
When to Use a Mutex
:
- Exclusive Access Only:
- Use a
Mutex
when only one thread needs to access the data at a time, regardless of whether it is for reading or writing. - Example: If your application requires consistent, synchronized access to a resource, like updating a counter or a shared state, a
Mutex
is a simple choice.
- Use a
- Write-Heavy Workloads:
- If your workload involves frequent writes and few reads, a
Mutex
can be more efficient than anRwLock
, as it avoids the overhead of maintaining separate locks for readers and writers.
- If your workload involves frequent writes and few reads, a
- Simpler API:
- A
Mutex
provides a straightforward locking mechanism with no distinction between readers and writers, making it simpler to reason about in certain cases.
- A
- Use in Async Contexts:
- If you’re working in an async context and require synchronization, use
tokio::sync::Mutex
(an async-friendly mutex) sincestd::sync::Mutex
would block the thread.
- If you’re working in an async context and require synchronization, use
When to Use an RwLock
:
- Read-Mostly Workloads:
- If your data is read more frequently than it is written, an
RwLock
is often more performant because multiple readers can access the data simultaneously without blocking each other. - Example: A cache where reads dominate and writes are infrequent.
- If your data is read more frequently than it is written, an
- Fine-Grained Access Control:
RwLock
allows distinguishing between read and write access, making it a better choice if you need to explicitly control how threads interact with the data.
- Performance Optimization:
- In scenarios with many threads and primarily read-heavy operations, an
RwLock
minimizes contention by allowing multiple threads to read in parallel.
- In scenarios with many threads and primarily read-heavy operations, an
Key Considerations:
Feature | Mutex | RwLock |
---|---|---|
Concurrency | Only one thread can lock at a time. | Multiple readers or one writer. |
Performance | Better for write-heavy workloads. | Better for read-heavy workloads. |
API Simplicity | Simple, only one type of lock. | More complex, with separate read and write locks. |
Overhead | Lower overhead in simple cases. | Slightly higher due to managing reader/writer states. |
When to Prefer One Over the Other:
- If most operations are reads, and contention would be a concern, use an
RwLock
. - If data is frequently modified, and writes dominate the workload, stick with a
Mutex
. - When simplicity is paramount and you don’t need the distinction between readers and writers, a
Mutex
is often easier to use and reason about.
Example Use Cases:
Mutex Example:
use std::sync::Mutex;
let data = Mutex::new(0);
{
let mut lock = data.lock().unwrap();
*lock += 1; // Exclusive access for modification.
}
RwLock Example:
use std::sync::RwLock;
let data = RwLock::new(0);
// Multiple readers can acquire the read lock.
{
let read_lock = data.read().unwrap();
println!("Value: {}", *read_lock);
}
// Only one writer can acquire the write lock.
{
let mut write_lock = data.write().unwrap();
*write_lock += 1;
}
Choosing the correct lock depends heavily on understanding the expected access patterns and the potential contention in your program!
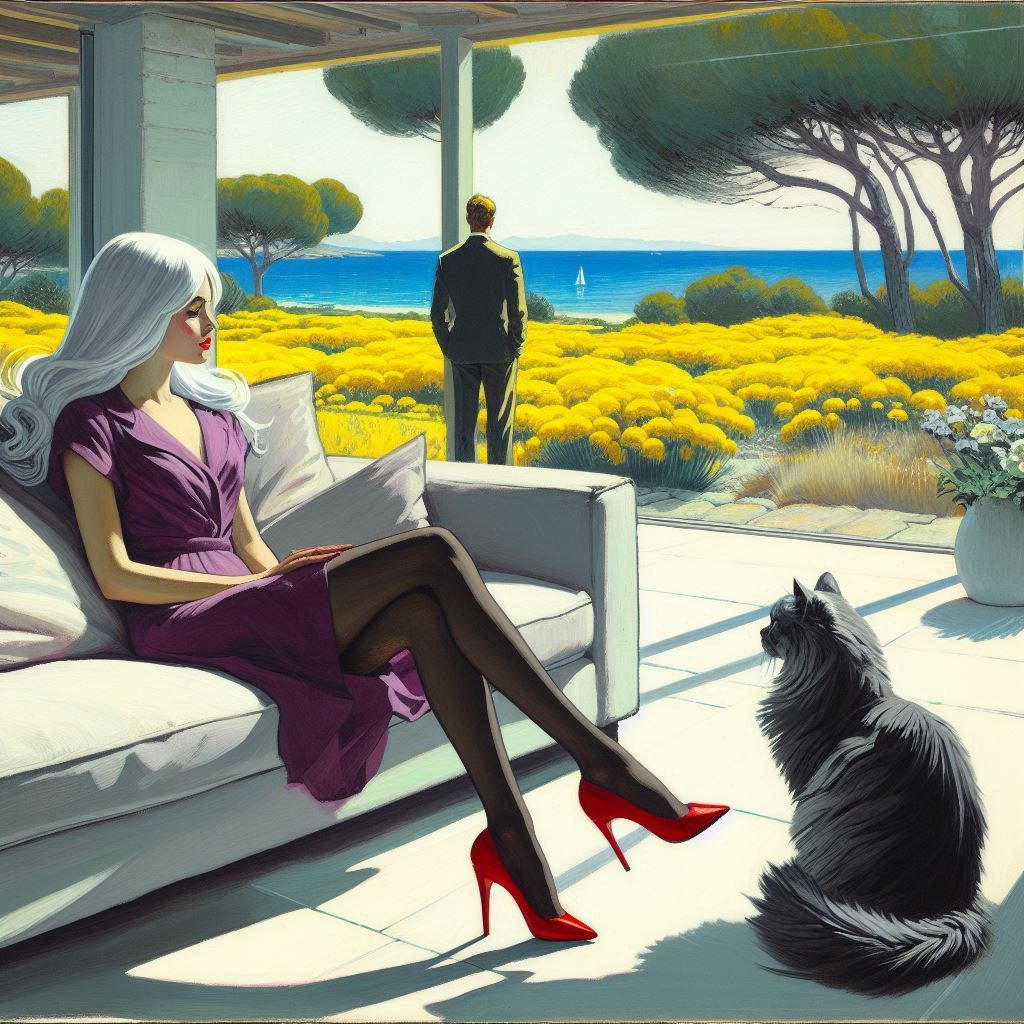
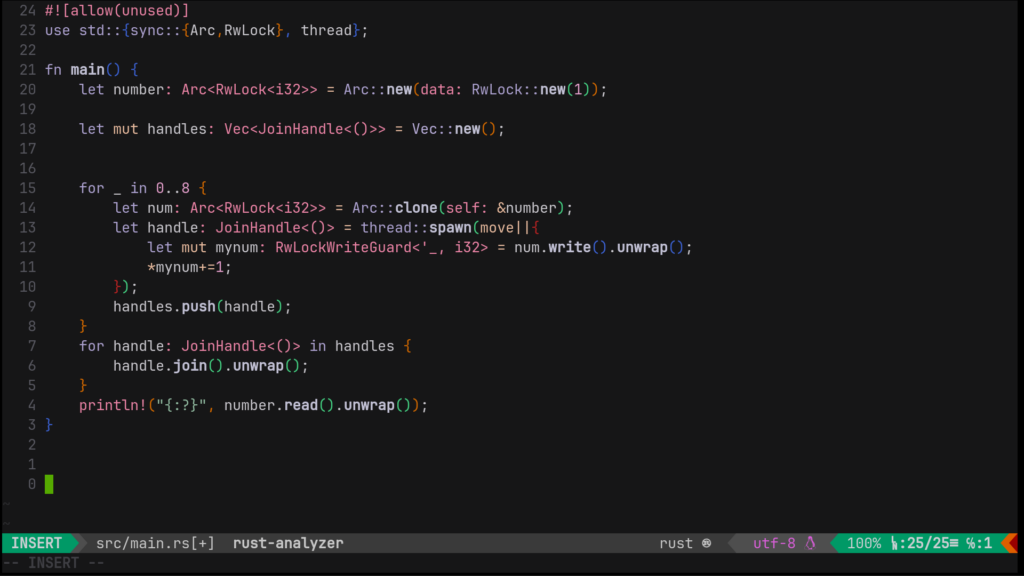
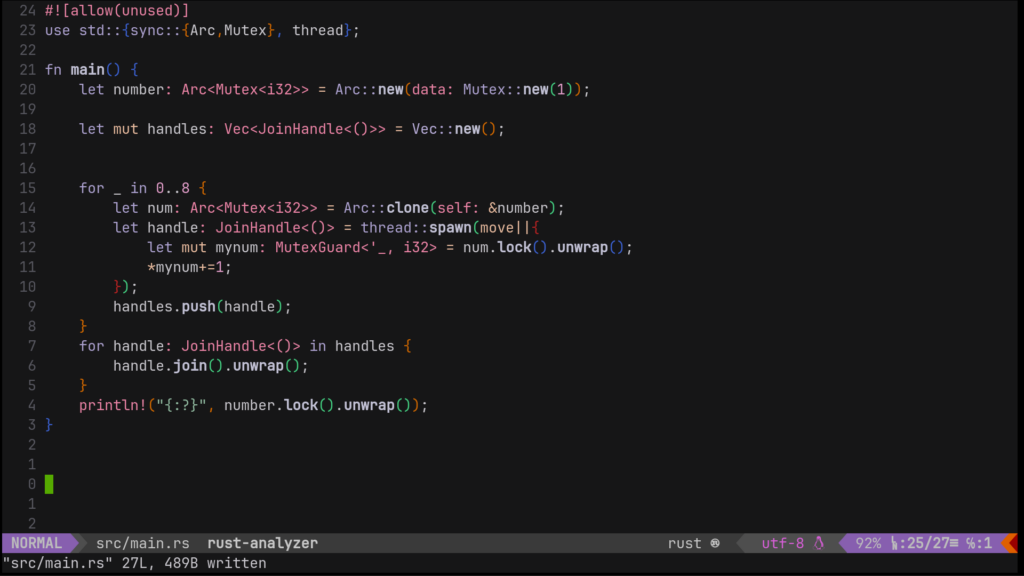
Poisoning
An RwLock
, like Mutex
, will become poisoned on a panic. Note, however, that an RwLock
may only be poisoned if a panic occurs while it is locked exclusively (write mode). If a panic occurs in any reader, then the lock will not be poisoned.