map_err challenge
Error Handling with Custom Error Types in Rust
Task/Problem Statement
You are developing a command-line application that processes a text file containing a single integer. The application should:
- Read the contents of the specified text file.
- Parse the content as an integer.
- Handle various potential errors gracefully, providing meaningful error messages to the user.
Requirements:
- If the file cannot be opened (e.g., it doesn’t exist), the application should return an informative error message indicating the I/O error.
- If the file is empty, the application should return a specific error message indicating that the file is empty.
- If the contents of the file cannot be parsed as an integer, the application should return an error message detailing the parsing issue.
- The application should print the resulting integer to the console if everything is successful.
Context
The solution provided handles the above requirements by implementing a custom error type (MyError
) that encapsulates different kinds of errors (I/O errors, parsing errors, and empty file errors). It also includes the implementation of the Display
trait to provide user-friendly error messages, ensuring the application can communicate issues clearly to the user.
When the user runs the application and provides the path to the text file, the program uses the function read_and_parse_file
, effectively managing and reporting any encountered errors through the defined error types.
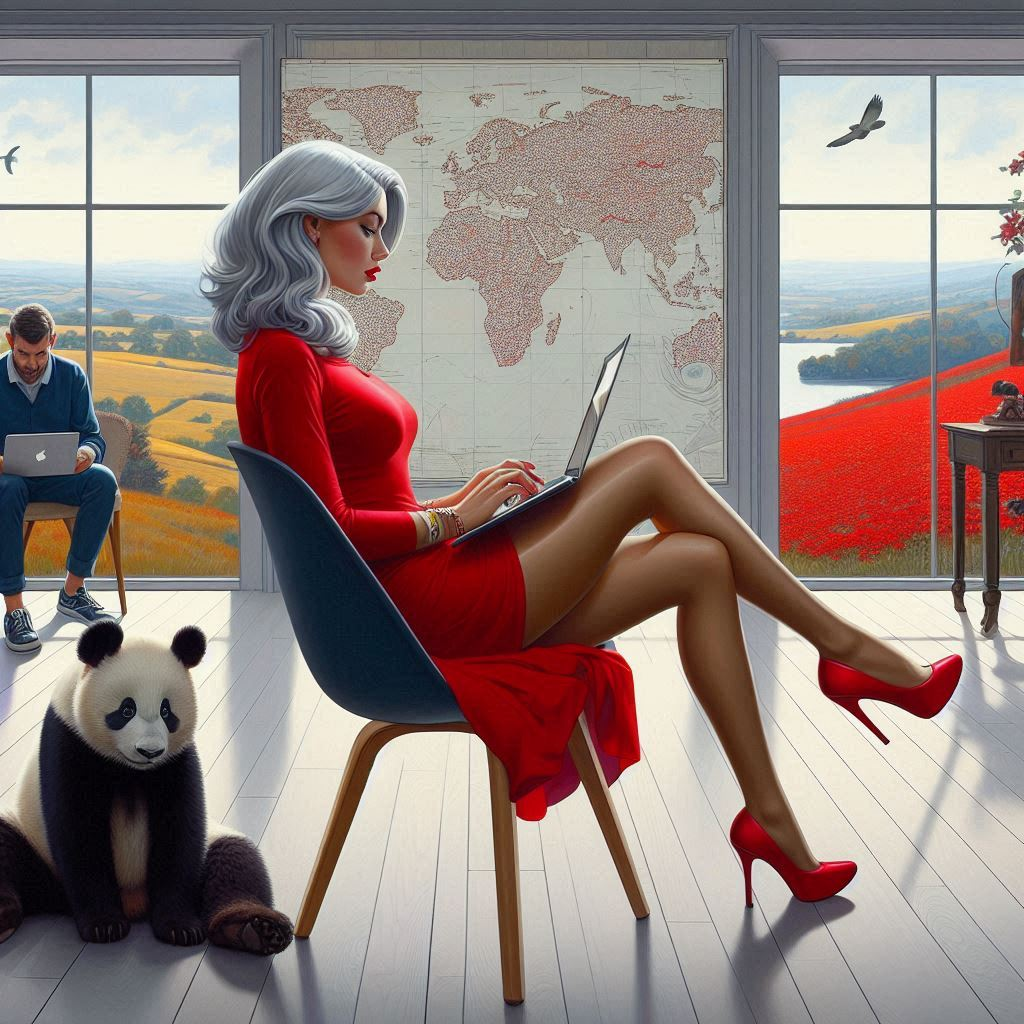
~/rust/er1/src main*
❯ cat number.txt
1
~/rust/er1/src main*
❯ tree -L 2
.
├── main.rs
└── number.txt
0 directories, 2 files
Solution
use std::fs;
use std::num::ParseIntError;
use std::io;
use std::error;
use std::fmt;
/// Custom error type to encapsulate different error kinds.
#[derive(Debug)]
enum MyError {
IO(io::Error),
Parse(ParseIntError),
EmptyFile,
}
impl fmt::Display for MyError {
fn fmt(&self, f: &mut fmt::Formatter<'_>) -> fmt::Result {
match self {
MyError::IO(err) => write!(f, "I/O Error: {}", err),
MyError::Parse(err) => write!(f, "Parse Error: {}", err),
MyError::EmptyFile => write!(f, "Error: The file is empty."),
}
}
}
impl error::Error for MyError {}
/// Reads a file and attempts to parse its content as an i32.
///
/// # Arguments
///
/// * `file_path` - A string slice that holds the path to the file.
///
/// # Returns
///
/// * `Result<i32, MyError>` - Returns the parsed integer or a custom error.
fn read_and_parse_file(file_path: &str) -> Result<i32, MyError> {
// Attempt to read the file, mapping any I/O errors
let content = fs::read_to_string(file_path).map_err(MyError::IO)?;
// Check if the file is empty and return a custom error if so
if content.trim().is_empty() {
return Err(MyError::EmptyFile);
}
// Try to parse the content as an integer, mapping any parse errors
let number = content.trim().parse::<i32>().map_err(MyError::Parse)?;
Ok(number)
}
fn main() {
match read_and_parse_file("number.txt") {
Ok(number) => println!("The number is: {}", number),
Err(e) => eprintln!("Error: {}", e),
}
}
Use ?
in functions where you want to propagate errors up to the caller. This allows the function to remain clean and focused on its main task while delegating error handling to the caller.
Implementing the Error
Trait:
impl error::Error for MyError {}
This line allows MyError
to be treated as an error type in Rust’s standard error handling framework.
By implementing this trait, MyError
can be returned from functions that expect a type that implements the std::error::Error
trait.
The Error
trait does not require you to define any additional methods when you implement it for an enum like MyError
, since it can delegate to the contained errors (like io::Error
and ParseIntError
).
~/rust/er1/src main*
❯ cargo r
Finished `dev` profile [unoptimized + debuginfo] target(s) in 0.00s
Running `/home/rag/rust/er1/target/debug/er1`
Parsed number 1
Summary
Define a Custom Error Enum: Create an enum (MyError
) to represent possible error types, such as I/O errors, parsing errors, and a custom EmptyFile
error for handling specific cases.
Implement Display
and Error
for the Enum: Add fmt::Display
for custom formatting of error messages, and implement std::error::Error
for compatibility with standard Rust error handling.
A note about the enums
In the match
expression, you’re explicitly destructuring the enum and binding the associated data to err
, so that you can directly use err
within each arm. This is standard pattern matching in Rust and requires you to specify each piece of data you want to access.
On the other hand, map_err
benefits from Rust’s syntactic sugar. When you pass an enum variant with associated data (like MyError::IO
) directly to map_err
, Rust treats it as a “single-argument constructor” for that variant, effectively creating a closure that takes the error and wraps it for you. This makes map_err(MyError::IO)
a shorthand for map_err(|e| MyError::IO(e))
, as Rust implicitly assumes you’re wrapping the error into MyError::IO
.
So:
- In
map_err
: You can useMyError::IO
directly, thanks to Rust’s shorthand. - In
match
: You need to specifyerr
(or another variable name) to bind to the data insideMyError::IO
orMyError::Parse
, becausematch
requires explicit pattern matching to access contained data.
This difference helps make your code more concise in functional-style error handling with map_err
while still giving you full control in match
.