How to use serde_json to handle data and extract fields
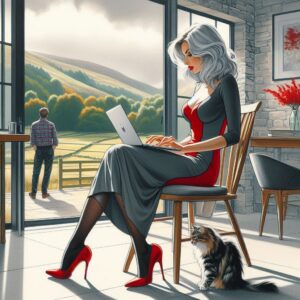
Using serde_json
to Handle Fake Data in Rust
When working with JSON data in Rust, serde_json
provides a convenient way to parse and manipulate JSON values. In this post, we’ll demonstrate how to use serde_json::Value
to create some mock data, simulate a search result, and extract specific fields from it.
Creating Fake Data and Extracting Fields
To illustrate this, we will:
- Generate Fake Data: Create some JSON objects with various fields.
- Create Mock Search Results: Simulate search results containing these JSON objects.
- Extract and Print Specific Fields: Extract and print the “description” field from the mock data.
Example Code
Here’s a full example demonstrating these steps:
use serde_json::{json, Value as JsonValue};
#[derive(Debug)]
struct ScoredPoint {
id: Option<u64>,
payload: JsonValue,
}
#[derive(Debug)]
struct SearchResult {
result: Vec<ScoredPoint>,
}
fn main() {
// Create some fake data
let fake_data = vec![
json!({
"title": "Spacious 3 bedroom house",
"price": 350000,
"address": "123 Elm Street",
"description": "A beautiful and spacious 3 bedroom house with a large garden.",
"link": "https://example.com/property/123"
}),
json!({
"title": "Cozy 2 bedroom apartment",
"price": 220000,
"address": "456 Maple Avenue",
"description": "A cozy 2 bedroom apartment located in a friendly neighborhood.",
"link": "https://example.com/property/456"
}),
];
// Create mock search results
let search_result = SearchResult {
result: fake_data
.into_iter()
.enumerate()
.map(|(i, payload)| ScoredPoint {
id: Some(i as u64),
payload,
})
.collect(),
};
// Iterate over all search results
for found_point in search_result.result {
let payload = found_point.payload;
let id = found_point.id.unwrap_or_default(); // Use `unwrap_or_default()` to handle Option
println!("Found point ID: {}", id);
if let Some(description) = payload.get("description") {
if let Some(description_str) = description.as_str() {
println!("Found description: {}", description_str);
} else {
println!("Description is not a string.");
}
} else {
println!("Description field not found.");
}
// Optionally, print the link field
if let Some(link) = payload.get("link") {
if let Some(link_str) = link.as_str() {
println!("Found link: {}", link_str);
} else {
println!("Link is not a string.");
}
} else {
println!("Link field not found.");
}
}
}
Explanation
- Generating Fake Data: Two JSON objects are created with various fields including “description”. This simulates the data we might receive from a search result.
- Creating Mock Search Results: A
SearchResult
struct is created containing a vector ofScoredPoint
objects. EachScoredPoint
includes an ID and a JSON payload. - Extracting and Printing the “Description” Field: The code iterates over all results, checking if the “description” field exists in each payload. If it does, it prints the description.
Running the Example
To run this code:
- Add Dependencies:
[dependencies]
serde = { version = "1.0", features = ["derive"] }
serde_json = "1.0"
- Save and Run: Save the Rust code to a file, e.g.,
main.rs
, and run it usingcargo run
in your project directory.
This example will print the description and optionally the link from each result in the mock data. Modify the data and structure as needed for your specific use case.