function items and function pointers in Rust
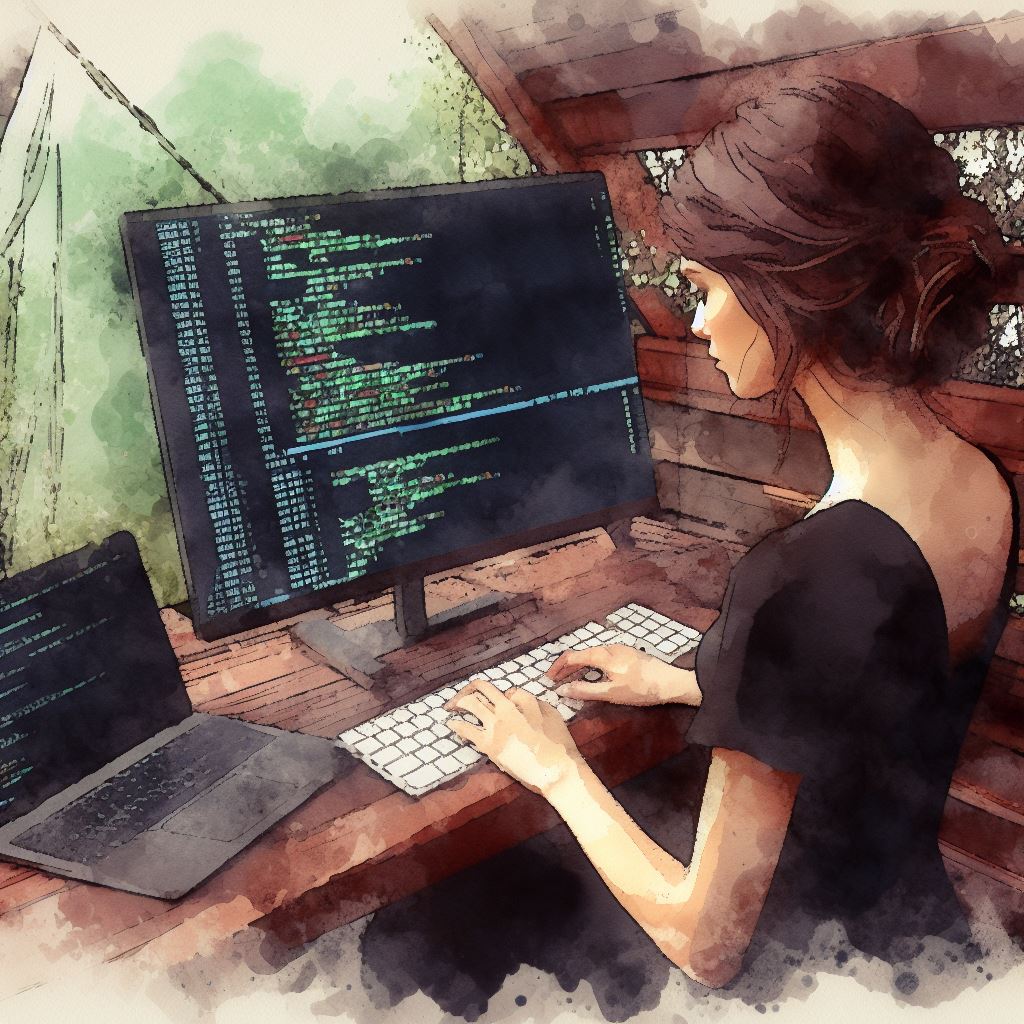
In Rust, a “function signature” refers to the unique description of a function, which includes the following components:
- Function Name: This is the name of the function that identifies it within your code. For example, in the function
fn add(a: i32, b: i32) -> i32
, the name is “add.” - Parameter Types: These specify the types of values or data that the function accepts as input. In the same example,
a
andb
are parameters, and their types arei32
. This means the function takes two 32-bit signed integers as input. - Return Type: This indicates the type of value that the function will produce as its output or result. In the example,
-> i32
indicates that the function will return a 32-bit signed integer as its result.
Webdock – Fast Cloud VPS Hosting
Function Items in Rust:
So, when we talk about a “function item” in Rust, we are essentially referring to the function’s name along with its signature, which includes the name, parameter types, and return type. This combination uniquely identifies the function within your codebase.
Here’s the breakdown using the add
function example:
- Function Name:
add
- Parameter Types:
a: i32
,b: i32
- Return Type:
i32
Putting it all together, the function item for add
would be described as follows: “The function named add
that takes two i32
parameters and returns an i32
.” This description provides all the necessary information to understand how the function works and how it can be used in your code.
- First-Class Functions: In Rust, functions are first-class citizens, meaning they can be assigned to variables, passed as arguments to other functions, and returned from functions.
- Function Signature: A function item is a function’s identifier along with its signature, which includes its name, parameter types, and return type.
- Declaring Functions: Functions can be declared using the
fn
keyword followed by a name, parameters, and a return type, likefn add(a: i32, b: i32) -> i32
. - Variable Assignment: You can assign a function item to a variable, making it easy to reuse or pass around. For example,
let add_func = add;
. - Function Calls: Calling a function item is similar to calling a regular function. You use the variable name followed by parentheses and provide arguments.
Function Pointers in Rust:
- Pointer to Functions: A function pointer is a variable that can hold the memory address of a function, allowing you to call that function indirectly.
- Declaration: Function pointers are declared using the
fn
keyword followed by a pointer type, such asfn(i32, i32) -> i32
. - Assigning Functions: You can assign function items or functions to function pointers. For instance,
let add_ptr: fn(i32, i32) -> i32 = add;
. - Calling via Pointers: To call a function through a pointer, use the dereference operator (
*
) on the pointer variable, followed by parentheses and arguments:let result = (*add_ptr)(5, 3);
. - Flexibility: Function pointers provide flexibility in choosing which function to call at runtime, making them useful for implementing callbacks, dynamic dispatch, or pluggable algorithms.
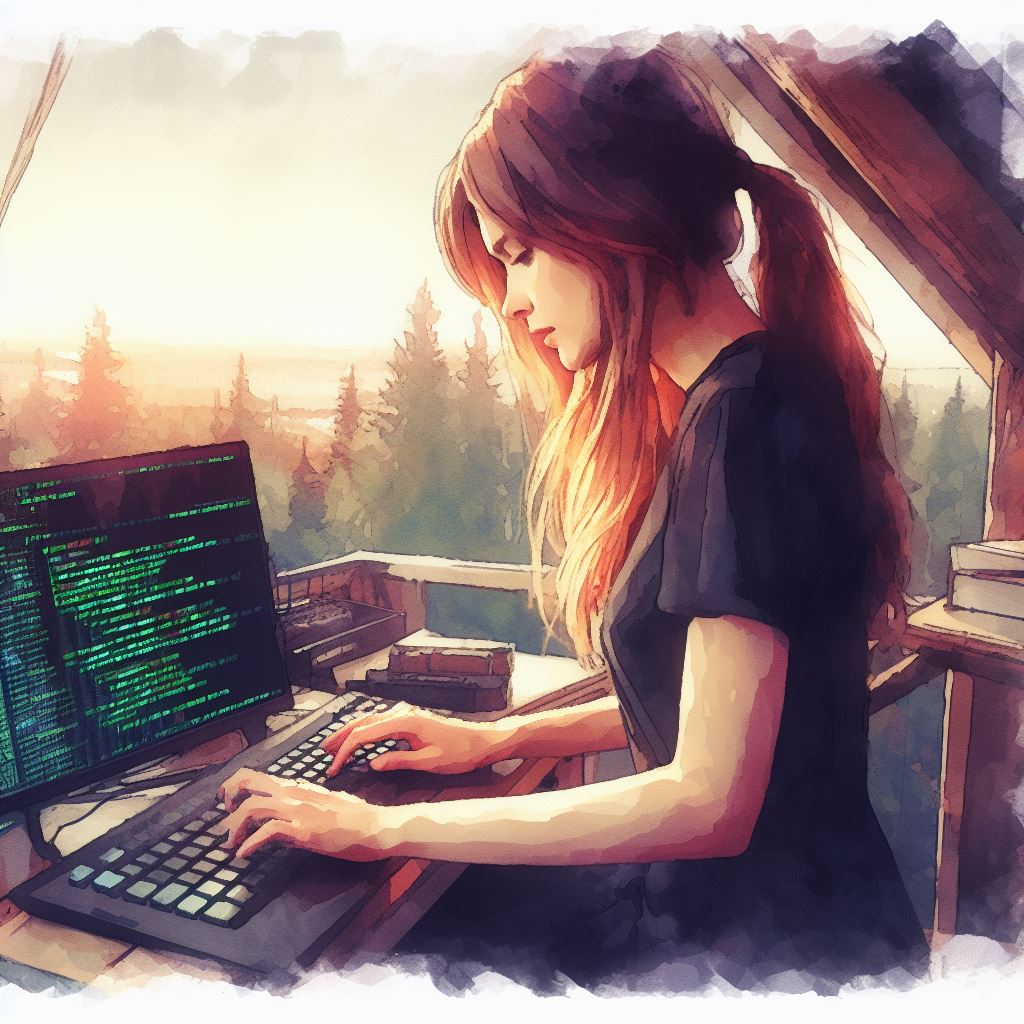
Code:
In Rust, you can use pointers to functions to create more flexible and dynamic code. Here’s the most simple example of using a pointer to a function in Rust:
fn add(x: i32, y: i32) -> i32 {
x + y
}
fn subtract(x: i32, y: i32) -> i32 {
x - y
}
fn main() {
// Declare a function pointer that can point to a function taking two i32 arguments and returning an i32.
let operation: fn(i32, i32) -> i32;
// Assign the function pointer to point to the "add" function.
operation = add;
// Use the function pointer to call the "add" function.
let result = operation(10, 5);
println!("Result of add: {}", result);
// Change the function pointer to point to the "subtract" function.
operation = subtract;
// Use the function pointer to call the "subtract" function.
let result = operation(10, 5);
println!("Result of subtract: {}", result);
}
In this example, we define two simple functions, add
and subtract
, which take two i32
arguments and return an i32
result. We then declare a function pointer operation
that can point to functions with the same signature. Initially, we set it to point to the add
function and later change it to point to the subtract
function. Finally, we use the function pointer to call the pointed-to function with different operations.
Remember that Rust’s type system is strict, so the function pointer’s signature must match the functions it points to.
Summary:
- In Rust, functions are first-class citizens, which means they can be treated like any other data type.
- Function items are function names along with their parameter types and return types. They can be assigned to variables and called directly.
- Function pointers are variables that hold memory addresses of functions. They allow for indirect function calls.
- Function pointers are declared with the
fn
keyword followed by a pointer type, and you can assign functions or function items to them. - Using function pointers offers flexibility and is essential for scenarios where you need to choose which function to call dynamically, such as callbacks and dynamic dispatch.
- Understanding function items and function pointers is valuable for Rust developers, especially when dealing with complex, data-driven, or dynamically determined function invocations.
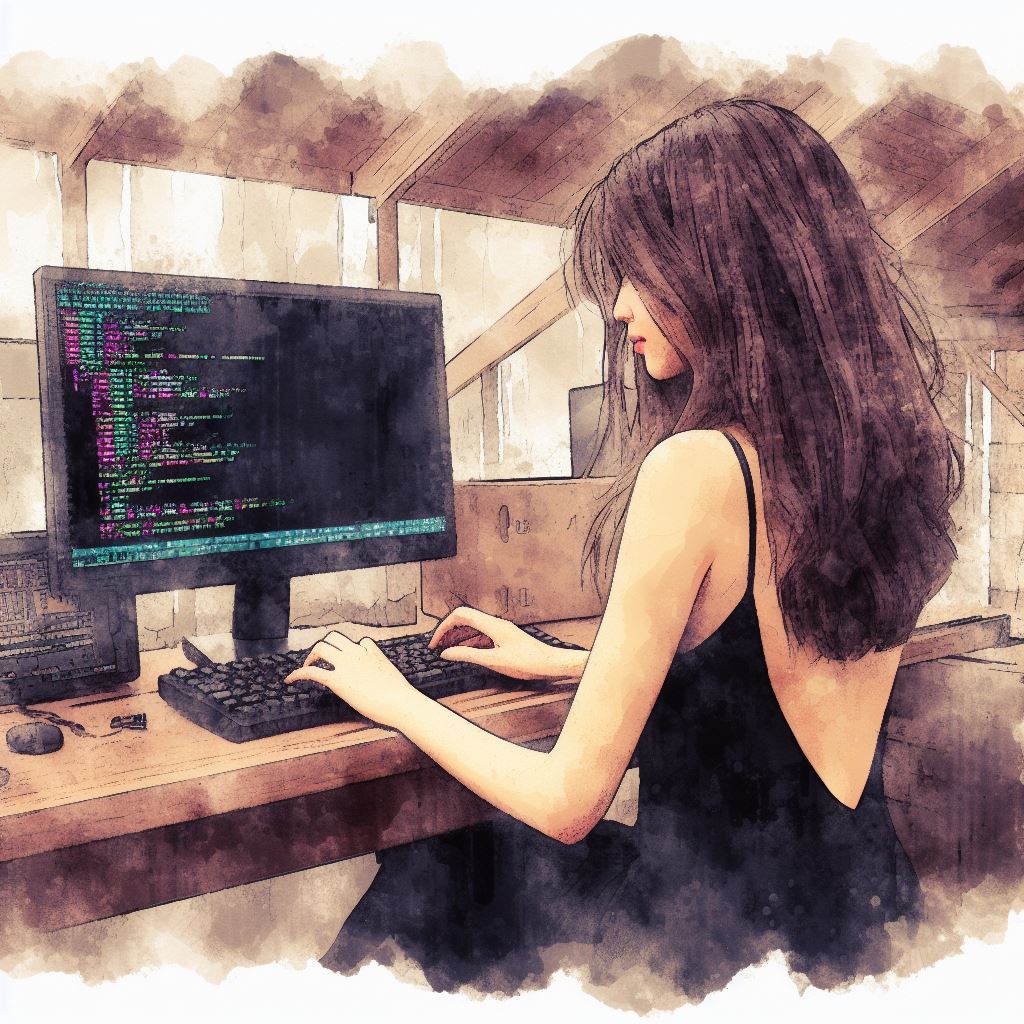