as_ref and Cow
Despite serving different roles, as_ref
and Cow
share a common goal: enabling flexibility in how data is handled, especially with respect to ownership and borrowing. Both allow your code to work with a broader range of types—as_ref
by accepting inputs that can be referenced as a common type, and Cow
by returning data that may be either borrowed or owned. In doing so, they help reduce unnecessary cloning and allocation, promoting zero-cost abstractions where possible. Each tool lets you write more generic and efficient code without sacrificing safety, making them valuable in performance-conscious Rust programs.
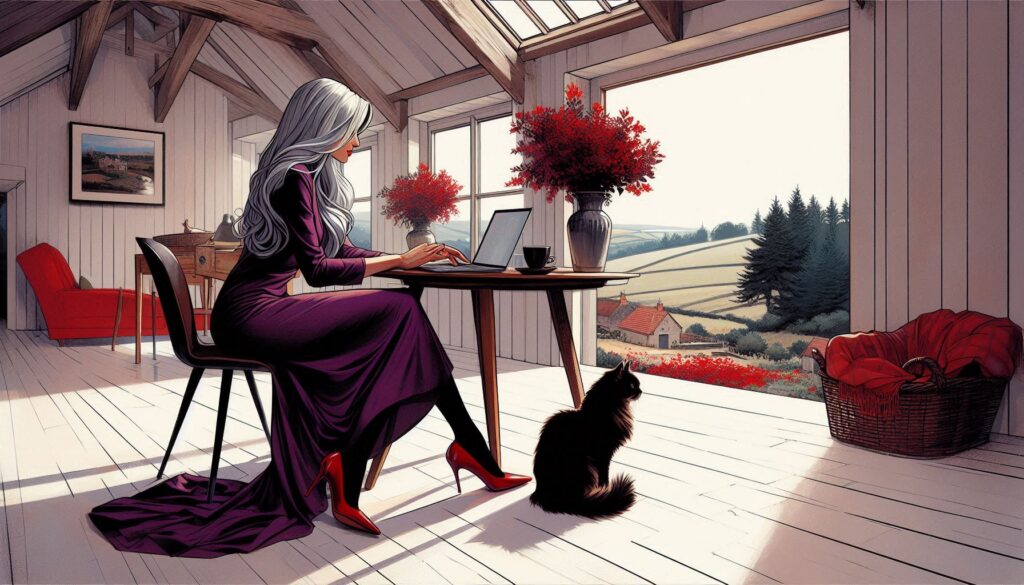
Introduction
Rust’s ownership system is powerful but sometimes requires flexibility. Two tools that help manage references and ownership efficiently are:
AsRef
trait/as_ref()
method: Converts a value into a reference of another typeCow
(Clone on Write): Provides a smart pointer for borrowed or owned data
This guide explores both concepts with practical examples showing when and how to use each.
Part 1: Understanding AsRef
and as_ref()
What is AsRef
?
AsRef
is a trait that allows converting a value into a reference of another type. It’s defined as:
pub trait AsRef<T: ?Sized> {
fn as_ref(&self) -> &T;
}
The as_ref()
method takes a reference to self
and returns a reference to type T
.
Basic Example
fn main() {
let s = String::from("Hello, world!");
// Using as_ref() to convert &String to &str
let str_ref: &str = s.as_ref();
println!("Original: {}", s);
println!("Reference: {}", str_ref);
}
Why Use AsRef
?
The main advantage is writing functions that can accept multiple types that can be referenced as the same type.
Generic Functions with AsRef
// A function that works with anything that can be referenced as &str
fn print_length<T: AsRef<str>>(text: T) {
let text_ref = text.as_ref();
println!("Length: {}", text_ref.len());
}
fn main() {
// Works with String
let owned = String::from("Hello");
print_length(owned);
// Works with &str
let borrowed = "World";
print_length(borrowed);
// Works with &String too
let another = String::from("Testing");
print_length(&another);
}
Common AsRef
Implementations
Rust provides many built-in implementations:
String
implementsAsRef<str>
Vec<T>
implementsAsRef<[T]>
String
implementsAsRef<[u8]>
(view as bytes)PathBuf
implementsAsRef<Path>
Real-World Example: File Operations
use std::fs::File;
use std::path::Path;
use std::io::{self, Read};
// Function works with anything that can be converted to &Path
fn read_file<P: AsRef<Path>>(path: P) -> io::Result<String> {
let mut file = File::open(path)?;
let mut contents = String::new();
file.read_to_string(&mut contents)?;
Ok(contents)
}
fn main() -> io::Result<()> {
// Works with &str
let contents1 = read_file("Cargo.toml")?;
// Works with String
let path_string = String::from("Cargo.toml");
let contents2 = read_file(path_string)?;
// Works with PathBuf
let path_buf = std::path::PathBuf::from("Cargo.toml");
let contents3 = read_file(path_buf)?;
println!("All methods returned the same content: {}",
contents1 == contents2 && contents2 == contents3);
Ok(())
}
Part 2: Understanding Cow
(Clone on Write)
What is Cow
?
Cow
(Clone on Write) is an enum that allows you to work with either borrowed or owned data:
pub enum Cow<'a, B: ?Sized + 'a>
where
B: ToOwned,
{
Borrowed(&'a B),
Owned(<B as ToOwned>::Owned),
}
Basic Cow
Example
use std::borrow::Cow;
fn main() {
// Borrowed variant
let borrowed: Cow<str> = Cow::Borrowed("hello");
println!("Borrowed: {}", borrowed);
// Owned variant
let owned: Cow<str> = Cow::Owned(String::from("world"));
println!("Owned: {}", owned);
// Cow behaves like the underlying data
println!("Combined: {} {}", borrowed, owned);
}
Why Use Cow
?
Cow
is useful when:
- You might need to modify data, but often just read it
- You want to avoid unnecessary cloning
- Your function needs to return either borrowed or owned data
Demonstrating Cow
‘s Clone-on-Write Behavior
use std::borrow::Cow;
fn remove_spaces<'a>(input: &'a str) -> Cow<'a, str> {
if input.contains(' ') {
// Only allocate and clone if we need to modify
Cow::Owned(input.replace(' ', ""))
} else {
// No spaces? Just borrow the original
Cow::Borrowed(input)
}
}
fn main() {
let text1 = "Hello world";
let text2 = "HelloWorld";
let result1 = remove_spaces(text1);
let result2 = remove_spaces(text2);
println!("Original 1: '{}', Result 1: '{}'", text1, result1);
println!("Original 2: '{}', Result 2: '{}'", text2, result2);
match result1 {
Cow::Borrowed(_) => println!("Result 1 is borrowed (this won't happen)"),
Cow::Owned(_) => println!("Result 1 is owned (had to modify)"),
}
match result2 {
Cow::Borrowed(_) => println!("Result 2 is borrowed (no modification needed)"),
Cow::Owned(_) => println!("Result 2 is owned (this won't happen)"),
}
}
Real-World Example: Data Processing
use std::borrow::Cow;
struct User<'a> {
name: Cow<'a, str>,
}
impl<'a> User<'a> {
fn new<S>(name: S) -> User<'a>
where
S: Into<Cow<'a, str>>,
{
User { name: name.into() }
}
fn display_uppercase(&self) -> String {
self.name.to_uppercase()
}
// This modifies the name if it's not already normalized
fn normalize_name(&mut self) {
if self.name.contains(' ') {
// This will convert to Owned if it was Borrowed
self.name.to_mut().retain(|c| !c.is_whitespace());
}
}
}
fn main() {
// User with borrowed name
let mut user1 = User::new("John Doe");
println!("User 1: {}", user1.name);
// User with owned name
let mut user2 = User::new(String::from("Jane Smith"));
println!("User 2: {}", user2.name);
// Display without modifying the original
println!("Uppercase display: {}", user1.display_uppercase());
println!("Original still: {}", user1.name);
// Now modify - this will convert borrowed to owned if needed
user1.normalize_name();
user2.normalize_name();
println!("Normalized user 1: {}", user1.name); // JohnDoe
println!("Normalized user 2: {}", user2.name); // JaneSmith
}
Part 3: Comparing AsRef
and Cow
Similarities
- Both support zero-cost abstractions when possible
- Both handle flexible input/output types
- Both help avoid unnecessary allocations
Key Differences
Feature | AsRef | Cow |
---|---|---|
Purpose | Converting between reference types | Managing ownership/borrowing |
Direction | Input flexibility | Output flexibility |
Modification | Doesn’t support modification | Supports lazy modification |
Ownership | Doesn’t take ownership | Can take or borrow ownership |
Common use | Function parameters | Return values |
When to Use Each
- Use
AsRef
when:- You need a function to accept multiple input types
- You only need to read the data, not modify it
- You’re working with references
- Use
Cow
when:- You need to maybe modify data, but often just read it
- You want to delay allocation until needed
- Your function might need owned data or borrowed data
Part 4: Combined Example
use std::borrow::Cow;
use std::path::Path;
// Function using AsRef for flexible input
fn process_path<P: AsRef<Path>>(path: P) -> Cow<'static, str> {
let path_ref = path.as_ref();
// Get the filename as a string, with a fallback
let filename = path_ref
.file_name()
.and_then(|os_str| os_str.to_str())
.unwrap_or("unknown");
// If the filename is "config.toml", return a static string (borrowed)
// Otherwise, create a custom message (owned)
if filename == "config.toml" {
Cow::Borrowed("Found the config file!")
} else {
Cow::Owned(format!("Found another file: {}", filename))
}
}
fn main() {
// Test with different path types
let path1 = "config.toml";
let path2 = String::from("data.json");
let path3 = std::path::PathBuf::from("user.yaml");
println!("{}", process_path(path1));
println!("{}", process_path(path2));
println!("{}", process_path(path3));
// Demonstrating variance in return types
let result1 = process_path("config.toml");
let result2 = process_path("other.txt");
match result1 {
Cow::Borrowed(_) => println!("Result 1 is borrowed (no allocation)"),
Cow::Owned(_) => println!("Result 1 is owned (not expected)"),
}
match result2 {
Cow::Borrowed(_) => println!("Result 2 is borrowed (not expected)"),
Cow::Owned(_) => println!("Result 2 is owned (allocated)"),
}
}
Conclusion
AsRef
and Cow
are powerful tools in Rust’s ownership system:
AsRef
provides input flexibility, allowing functions to accept multiple types that can be referenced as a common type.Cow
provides output flexibility, delaying allocation until absolutely necessary.
Together, they enable writing more generic, efficient, and flexible code while maintaining Rust’s safety guarantees. By using these abstractions wisely, you can write code that is both more ergonomic and more performant.
Why do we see P ?
The use of P
in AsRef
examples is not specifically tied to Path
, though it can appear that way due to common conventions.
The letter P
is simply a generic type parameter name chosen by convention, and its meaning depends on context:
- In file/path handling: When you see
P: AsRef<Path>
, theP
often stands for “Path-like” because the function is accepting anything that can be referenced as a Path. This is extremely common in the standard library’s file operations:
fn open<P: AsRef<Path>>(path: P) -> Result<File>
- Generic type parameter: In other contexts,
P
might just be following the convention of using single uppercase letters for generic types (likeT
,U
, etc.). For example:
// P here could be any type that implements AsRef<str>
fn process_string<P: AsRef<str>>(text: P) { ... }
- Parameter naming convention: Some developers choose parameter names that align with their generic types, so you might see:
fn do_something<P>(p: P) where P: AsRef<SomeType> { ... }
The Rust standard library and community have developed certain patterns:
T
andU
for completely generic typesP
often for path-like or parameter typesS
often for string-like typesF
for function typesE
for error types
But there’s no hard requirement – you could just as easily write:
// Using X instead of P
fn read_file<X: AsRef<Path>>(path: X) -> Result<String> { ... }
It would work exactly the same, but might be less immediately clear to other Rust developers familiar with the conventions.
So while P
is very common with Path
due to the mnemonic association, it’s not reserved for paths – it’s just a naming convention that happened to catch on.