Implicit vs Manual Type Conversion in Rust: A Comprehensive Guide
When I’m programming in Rust, one of the core challenges revolves around efficiently managing types and ensuring clean, error-free conversions between them.
Why is this? Why even bother converting types? In this article we will study some examples.
Rust offers several ways to convert types, and two of the most common approaches are implicit and manual type conversions. Let’s look at these two techniques understand when and why to use them.
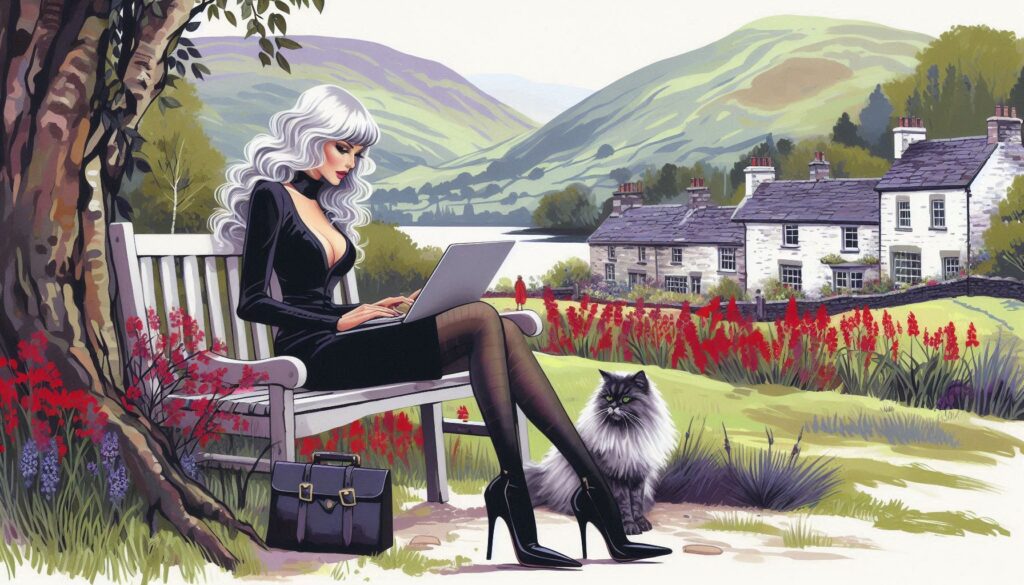
type conversion in rust
What Is Type Conversion?
Type conversion in Rust is the process of transforming a value of one type into another. Rust provides various mechanisms to handle this conversion, each with its own advantages. The two most common types of conversions are implicit (automatic) and manual (explicit) conversions.
Implicit Type Conversion (Using From
and Into
)
What is Implicit Conversion?
In Rust, implicit conversion is enabled through the From
and Into
traits. These traits allow Rust to automatically convert types without requiring “the programmer” to manually specify the conversion logic each time.
From
: TheFrom
trait is implemented to define how a type can be converted into another type.Into
: TheInto
trait is automatically implemented for types that implementFrom
, allowing you to convert a value into the target type using the.into()
method.
When you use implicit conversion, Rust does most of the work for you, allowing for cleaner, more concise code.
Example of Implicit Conversion – into()
struct UserId(String);
impl From<String> for UserId {
fn from(value: String) -> Self {
UserId(value)
}
}
fn main() {
let user: UserId = "user_123".to_string().into(); // Implicit conversion using `.into()`
println!("User ID: {}", user.0);
}
In the example above, we use From
to define how to convert a String
into a UserId
. The into()
method, provided by the Into
trait, makes this conversion implicit. You don’t need to call UserId::from()
directly. Rust handles it behind the scenes, making the code simpler and cleaner.
When to Use Implicit Conversion?
- Convenience: Use implicit conversion when you expect to perform conversions frequently and want to keep your code concise.
- Cleaner Code: When you want to make your code more readable by avoiding repetitive conversion logic.
- Flexibility: When you need to handle conversions from multiple types without writing a custom conversion function for each one.
Manual Type Conversion (Using impl
with new()
)
What is Manual Conversion?
In contrast to implicit conversion, manual conversion involves explicitly defining how to create and interact with custom types. You typically implement a constructor method (e.g., new()
) for your custom types, which allows you to manually convert one type into another. This approach gives you full control over the conversion process.
Example of Manual Conversion:
struct UserId(String);
impl UserId {
fn new(value: String) -> Self {
UserId(value)
}
fn get_value(&self) -> &str {
&self.0
}
}
fn main() {
let user = UserId::new("user_123".to_string()); // Manual conversion using `new()`
println!("User ID: {}", user.get_value());
}
Here, instead of relying on the From
trait, we explicitly use the new()
method to create a UserId
. This approach requires you to manually specify how the conversion is done, giving you more control over the logic and how you handle the input.
When to Use Manual Conversion?
- Explicit Control: Use manual conversion when you want more explicit control over the conversion logic, like adding validation or custom behavior.
- Less Frequent Conversions: If type conversions are relatively rare in your code, manual methods like
new()
are perfectly fine. - Custom Logic: When you need to include custom logic in the conversion (e.g., validation, sanitization) before creating the new type.
Implicit vs Manual Conversion: When to Use Which?
1. Convenience vs Control
- Implicit Conversion: Use it when you want convenience. It simplifies your code by automatically handling conversions, making it ideal for scenarios where the logic is straightforward and doesn’t need extra customization.
- Manual Conversion: Use it when you want control over the conversion process. This is ideal for cases where you need to add validation or more complex behaviors when creating your custom types.
2. Code Readability
- Implicit Conversion: Results in cleaner, more concise code. With
From
andInto
, conversions are handled automatically, making your code more readable and maintainable in cases where conversions are common. - Manual Conversion: Provides clarity on how types are created and ensures that custom logic is explicitly written, which is helpful for debugging and maintaining more complex conversions.
3. Performance
Both implicit and manual conversion mechanisms have negligible performance overhead in most cases, especially since they are resolved at compile time. However, manual conversions might be slightly more efficient if you need to do more than just a simple conversion, like performing checks or transformations.
Conclusion: Which One Should You Choose?
- Use Implicit Conversion when the conversion logic is simple, and you want your code to be as concise and readable as possible.
- Use Manual Conversion when you need more control over how types are created, especially if you want to add custom behavior or validation during the conversion process.
In many cases, implicit conversion is the better choice due to its simplicity, but there are always situations where manual conversion provides the necessary control and clarity.
By mastering both approaches, you’ll be able to choose the right conversion strategy based on your project’s requirements. Rust’s flexibility with types ensures you can create code that is both clean and efficient, no matter the approach you choose.