BytesN in a Rust Smart Contract
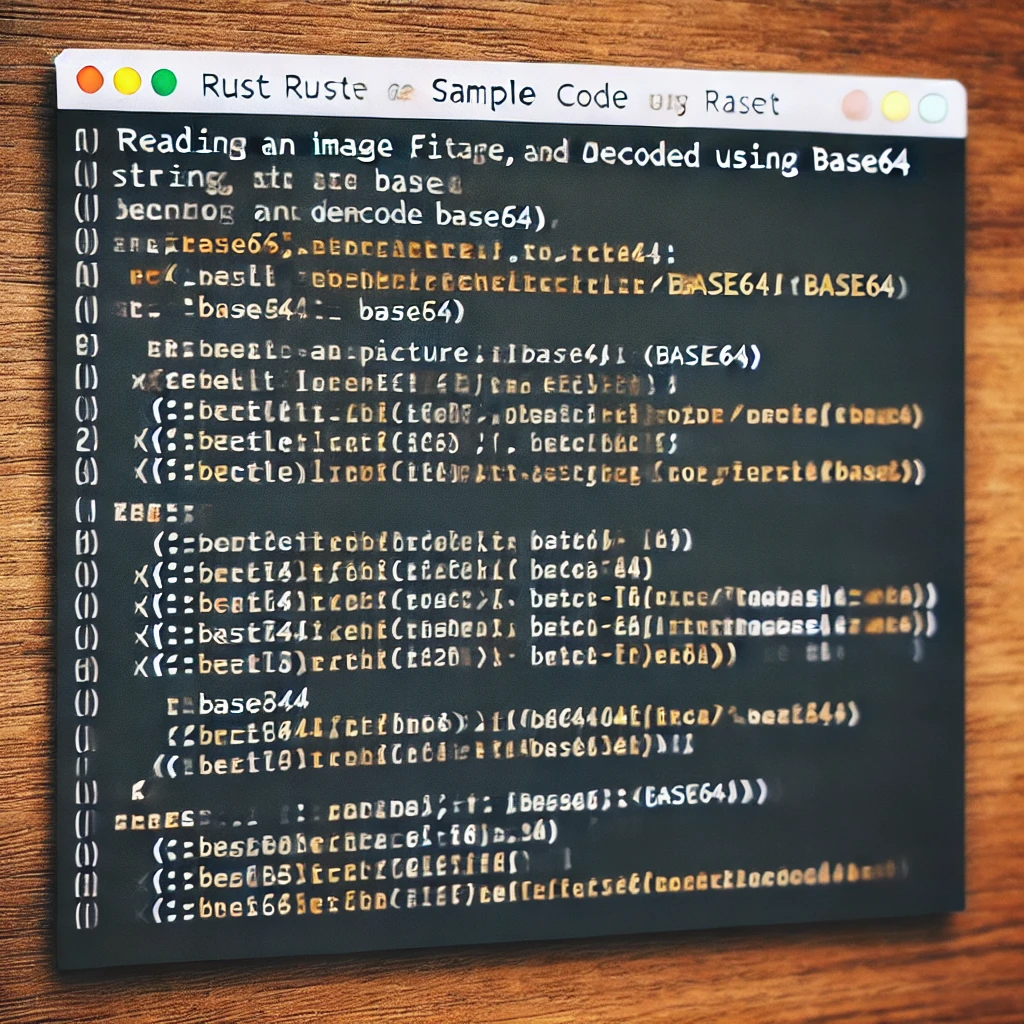
Smart contract in Rust – Stellar’s BytesN
Example code demo using BytesN
If you’re used to using symbol_short! macro, note how for more than 9 characters we need to use Symbol::new
We’ll use a fixed length 32 bytes array here so we need BytesN in Stellar :
#![no_std]
use soroban_sdk::{contract, contractimpl, Env, Symbol,BytesN};
#[contract]
pub struct MyContract;
#[contractimpl]
impl MyContract {
// Store a fixed-length byte array (BytesN) in contract storage
pub fn set_data(env: Env, data: BytesN<32>) {
let key = Symbol::new(&env,"stored__data");
env.storage().instance().set(&key, &data);
}
// Retrieve the stored fixed-length byte array (BytesN)
pub fn get_data(env: Env) -> Option<BytesN<32>> {
let key = Symbol::new(&env,"stored__data");
env.storage().instance().get(&key)
}
}
#[cfg(test)]
mod tests {
use super::*;
#[test]
fn test_set_and_get_data() {
let env = Env::default(); // Create an environment instance for testing
// Define a 32-byte array to use as test data
let bytes: [u8; 32] = [
1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16,
17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32
];
let data = BytesN::<32>::from(bytes); // Convert the array into a BytesN
// Set the data in the contract storage
MyContract::set_data(env.clone(), data.clone());
// Retrieve the data from the contract storage
let retrieved_data = MyContract::get_data(env);
// Check if the retrieved data is equal to the original data
assert_eq!(retrieved_data, Some(data));
}
}
You could also use base64 encoded :
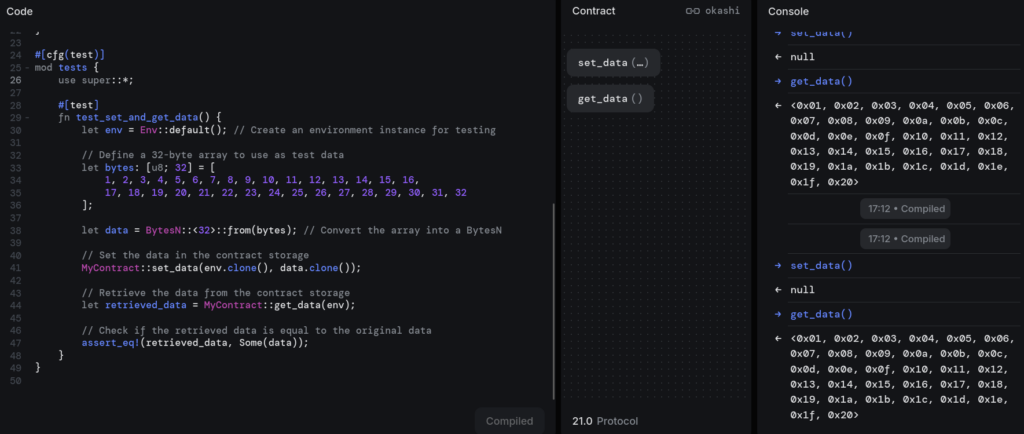
use base64::{encode};
fn main() {
// 32-byte array (can represent any data you want)
let bytes: [u8; 32] = [
1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16,
17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32
];
// Encode to Base64
let encoded = encode(&bytes);
// Print the Base64 encoded string
println!("Base64 Encoded: {}", encoded);
}