Understanding .as_slice() vs &[ ] in Rust
When working with Rust, you’ll often encounter scenarios where you need to interact with slices. Two common approaches for converting data structures to slices are using .as_slice()
and the &[ ]
syntax. While both can often achieve the same result, there are subtle differences in intent, safety, and compatibility that make each appropriate for different situations. Let’s dive into the details and explore why .as_slice()
is often the preferred choice.
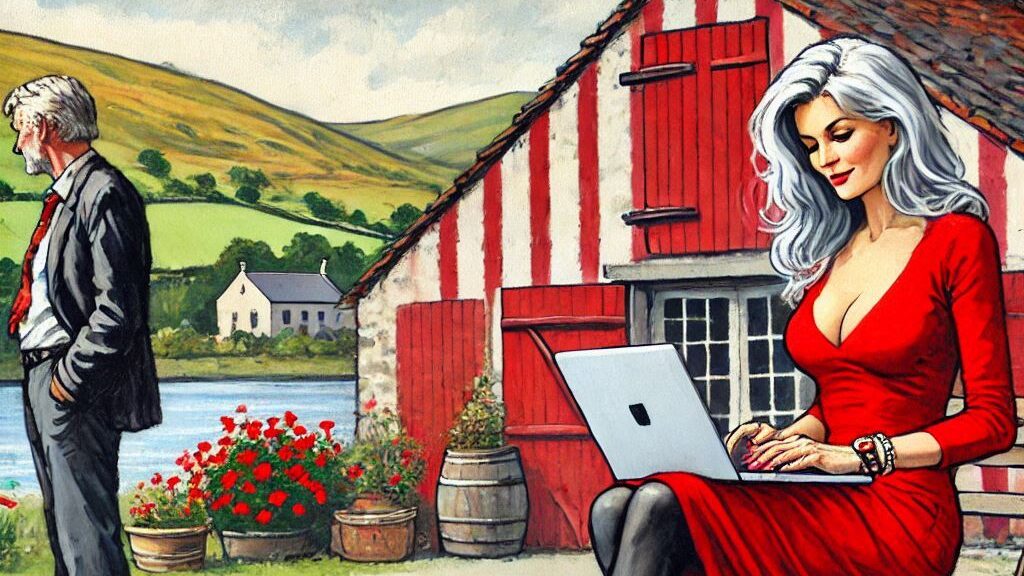
What Are Slices in Rust?
A slice (&[T]
) is a view into a contiguous sequence of elements, often used for efficient and safe access to arrays, vectors, or similar structures. Slices are widely used in Rust because they:
- Borrow data without taking ownership.
- Provide bounds-checked access to underlying elements.
- Work with many Rust standard library functions and traits.
.as_slice()
vs &[ ]
Syntax: The Basics
Using .as_slice()
The .as_slice()
method is provided by types like Vec<T>
and GenericArray<T, N>
(from crates like generic-array
). It explicitly converts the data structure into a slice. For example:
let vec = vec![1, 2, 3];
let slice = vec.as_slice(); // Convert Vec<T> to &[T]
Using &[ ]
The &[ ]
syntax can also create a slice by slicing into the data structure, like so:
let vec = vec![1, 2, 3];
let slice = &vec[..]; // Slice the entire Vec<T> as &[T]
Both approaches will produce the same result: a slice (&[1, 2, 3]
).
Key Differences Between .as_slice()
and &[ ]
1. Clarity of Intent
.as_slice()
explicitly signals that the purpose is to retrieve a slice view of the data structure. This method is self-documenting and makes the code easier to read and understand.&[ ]
syntax looks like slicing into the structure. While it works, it can be less clear to readers that you’re simply converting the entire structure into a slice.
Example:
let vec = vec![1, 2, 3];
let slice1 = vec.as_slice(); // Explicit: converting to slice
let slice2 = &vec[..]; // Works, but might look like slicing operation
2. Compatibility Across Data Structures
Some types, such as GenericArray
from the generic-array
crate, do not support slicing with &[ ]
, but they provide an .as_slice()
method. This makes .as_slice()
the more versatile option.
Example:
use generic_array::{GenericArray, typenum::U32};
let array: GenericArray<u8, U32> = GenericArray::default();
let slice = array.as_slice(); // Works
// let slice2 = &array[..]; // Error: GenericArray doesn’t implement slicing
3. Safety and Correctness
The .as_slice()
method guarantees access to the actual underlying slice of the data structure. It’s explicitly designed for types with contiguous memory layouts. On the other hand, &[ ]
relies on the slicing mechanism, which might not always be valid or supported.
4. Performance and Efficiency
Both .as_slice()
and &[ ]
are efficient and do not involve additional allocations. However, .as_slice()
avoids any ambiguity about the operation, while &[ ]
might involve implicit dereferencing in some contexts, which could lead to subtle bugs or misunderstandings.
When Should You Use .as_slice()
?
- Clarity: When you want to make it explicitly clear that you’re accessing the slice view of a data structure.
- Compatibility: When working with types like
GenericArray
or custom structs that do not implement slicing. - Consistency: For consistency across various types in your codebase, as
.as_slice()
is a standard method for many slice-compatible types.
Example:
let vec = vec![1, 2, 3];
let slice = vec.as_slice(); // Preferred for clarity and compatibility
When Should You Use &[ ]
?
- Simple Cases: For standard types like arrays or
Vec<T>
, if you’re slicing directly and the context is clear. - Slicing Subsets: If you’re not converting the entire structure but slicing a specific range,
&[ ]
is necessary.
Example:
let vec = vec![1, 2, 3, 4, 5];
let subset = &vec[1..3]; // &[2, 3]
Conclusion
While both .as_slice()
and &[ ]
can often achieve the same goal, .as_slice()
is preferred for its clarity, compatibility, and consistency across types. The &[ ]
syntax is still valuable for slicing operations, but it’s not always supported for more complex data structures like GenericArray
.
By choosing the right approach for your use case, you can write more readable and robust Rust code. Happy coding!