flatten v flat_map
flatten v flat_map – what’s the difference?
In Rust, flat_map
and flatten
are both methods used for transforming and simplifying nested structures, but they serve slightly different purposes and apply to different contexts.
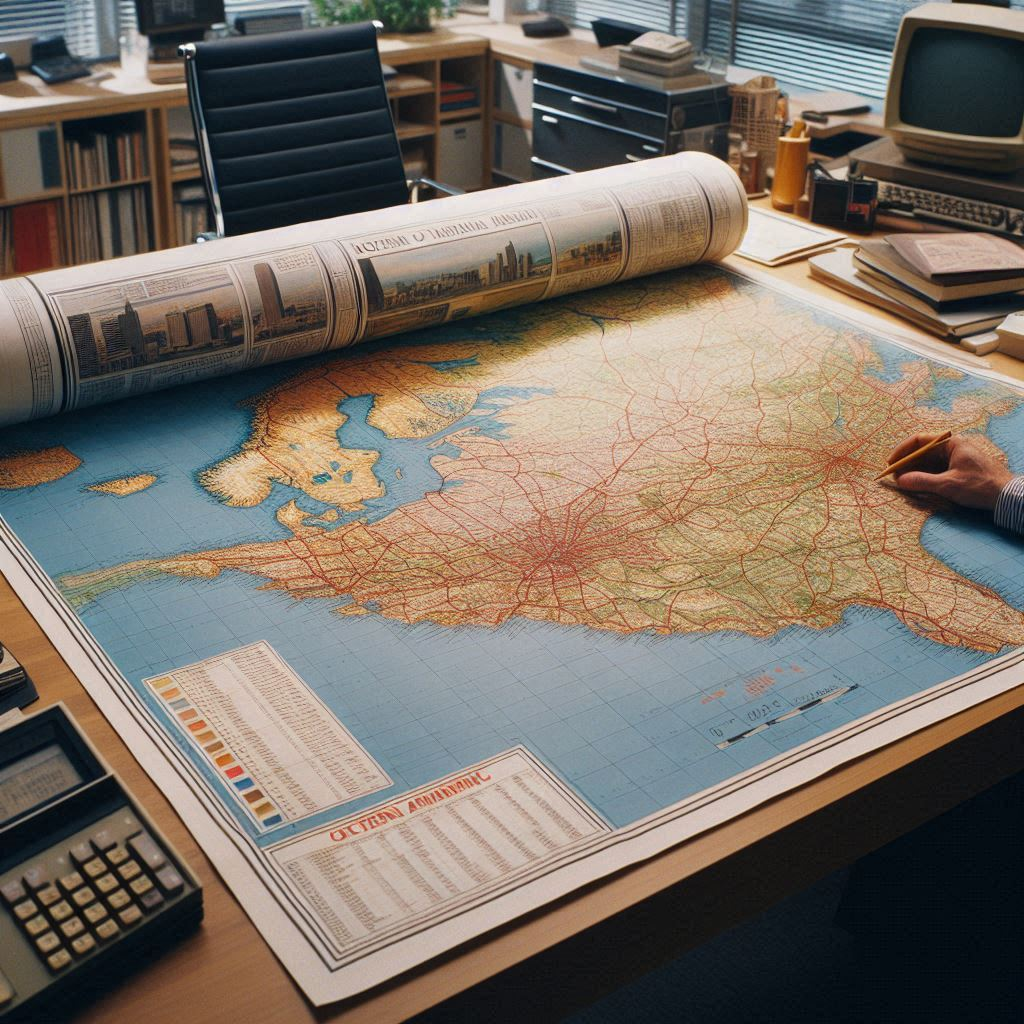
1. flat_map
- Usage:
flat_map
is an iterator method used to both map and flatten an iterable in one go. - Purpose: It applies a function to each element in an iterator, which produces another iterator for each element, and then it flattens those iterators into a single iterator.
- Common Use: When you want to apply a function that produces an iterator and then merge all the resulting iterators into one.
Example:
let numbers = vec![1, 2, 3];
let result: Vec<i32> = numbers
.into_iter()
.flat_map(|x| vec![x, x * 10]) // Multiply each number by 10 and pair it
.collect();
println!("{:?}", result); // Output: [1, 10, 2, 20, 3, 30]
Explanation:
flat_map(|x| vec![x, x * 10])
first applies the function|x| vec![x, x * 10]
, which creates a vector with two elements for eachx
.- Then,
flat_map
flattens these vectors into a single iterator, resulting in[1, 10, 2, 20, 3, 30]
.
2. flatten
- Usage:
flatten
is an iterator method that flattens one level of nested iterators orOption
/Result
types. - Purpose: It’s used when you have an iterator of iterators,
Option<Option<T>>
, orResult<Result<T, E>, E>
and want to “flatten” one level of nesting. - Common Use: When you have nested structures (like
Vec<Vec<T>>
) and want to collapse them into a single layer.
Example:
let nested = vec![vec![1, 2, 3], vec![4, 5], vec![6, 7, 8]];
let result: Vec<i32> = nested.into_iter().flatten().collect();
println!("{:?}", result); // Output: [1, 2, 3, 4, 5, 6, 7, 8]
Explanation:
- Here,
flatten
takes eachVec<i32>
inside the outerVec
, then merges them all into a single iterator, producing[1, 2, 3, 4, 5, 6, 7, 8]
.
Key Differences
flat_map
combines mapping and flattening in one step by applying a function that returns an iterator and then flattening it.flatten
only flattens; it doesn’t map or modify the elements.useflatten
to take a nested list of words and create a single flat list:
flatten v flat_map – Summary Table
Method | When to Use | Produces | Example |
---|---|---|---|
flat_map | Mapping to an iterator and flattening | Iterator<Item = T> | flat_map(|x| vec![x, x * 2]) |
flatten | Flattening nested structures | Iterator<Item = InnerType> | vec![vec![1,2], vec![3,4]].into_iter().flatten() |
These methods are helpful for transforming and simplifying data structures in Rust – flatten v flat_map – choose wisely!
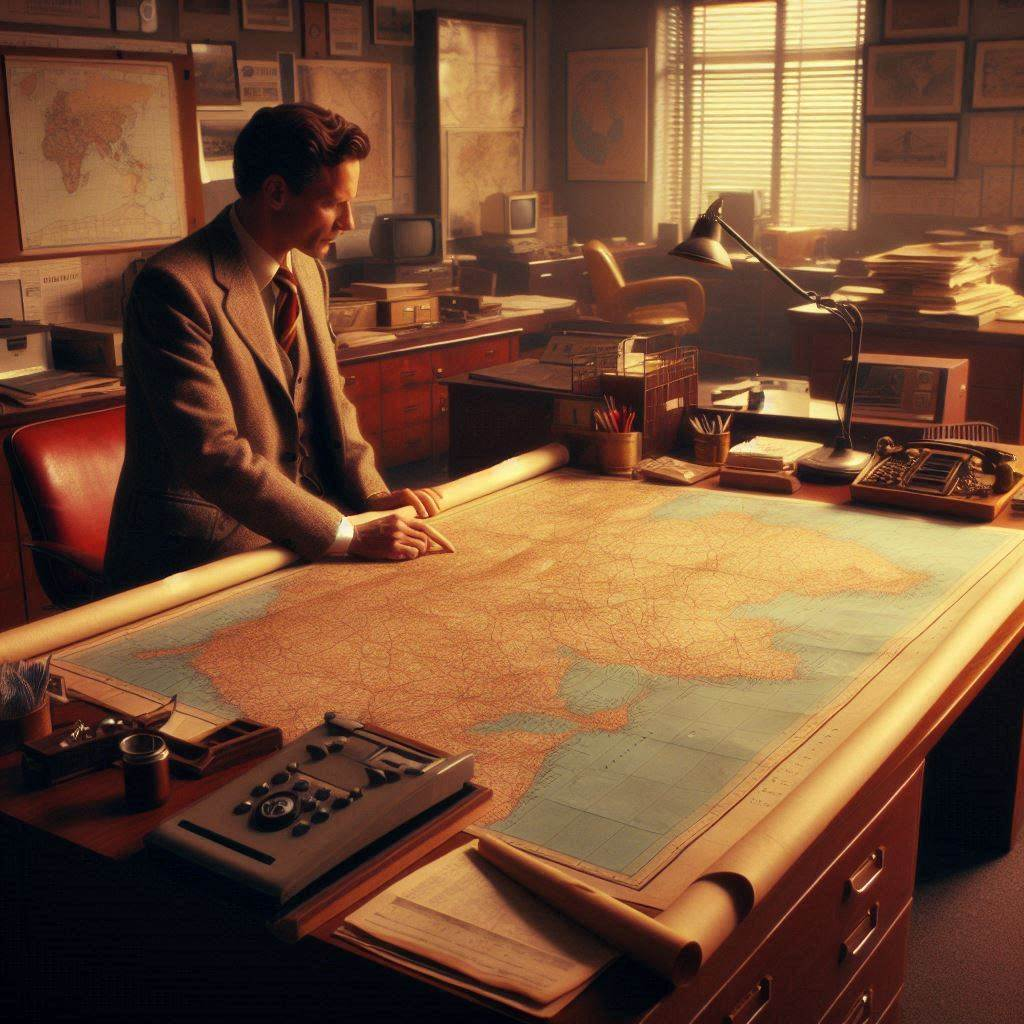
flatten v flat_map
use flatten
to take a nested list of words and create a single flat list:
fn main() {
let sentences = vec![
vec!["hello", "world"],
vec!["flatten", "is", "useful"],
vec!["in", "Rust"],
];
// Flatten the nested vector into a single iterator of words
let words: Vec<&str> = sentences
.into_iter() // Iterate over each inner vector
.flatten() // Flatten each inner vector into a single iterator of words
.collect(); // Collect all words into a Vec<&str>
println!("{:?}", words);
}
["hello", "world", "flatten", "is", "useful", "in", "Rust"]
Sure! Let’s look at how flatten
works with an example of nested collections of strings. Suppose we have a list of sentences, where each sentence is represented as a Vec<&str>
(a vector of words). By using flatten
, we can turn this nested structure into a single flat list of words.
Example Using flatten
Here’s how we could use flatten
to take a nested list of words and create a single flat list:
fn main() {
let sentences = vec![
vec!["hello", "world"],
vec!["flatten", "is", "useful"],
vec!["in", "Rust"],
];
// Flatten the nested vector into a single iterator of words
let words: Vec<&str> = sentences
.into_iter() // Iterate over each inner vector
.flatten() // Flatten each inner vector into a single iterator of words
.collect(); // Collect all words into a Vec<&str>
println!("{:?}", words);
}
Output:
["hello", "world", "flatten", "is", "useful", "in", "Rust"]
Explanation
into_iter()
goes through each inner vector (each sentence) in the outer vectorsentences
.flatten()
takes each inner vector of words and flattens it into a single iterator of words, discarding the nested structure.collect()
gathers the flattened list of words into a singleVec<&str>
.
Without flatten
If you didn’t use flatten
here, you’d end up with a Vec<Vec<&str>>
, keeping the nested structure. Here’s what it would look like without flatten
:
fn main() {
let sentences = vec![
vec!["hello", "world"],
vec!["flatten", "is", "useful"],
vec!["in", "Rust"],
];
// Without `flatten`, we get a nested vector
let words: Vec<Vec<&str>> = sentences
.into_iter()
.collect();
println!("{:?}", words);
}
Output without flatten
:
[["hello", "world"], ["flatten", "is", "useful"], ["in", "Rust"]]
Using flatten
is ideal here, as it gives us a simple, flat list of words by eliminating the nested structure!