Creating a WebSocket Chat Room with Rust and Warp
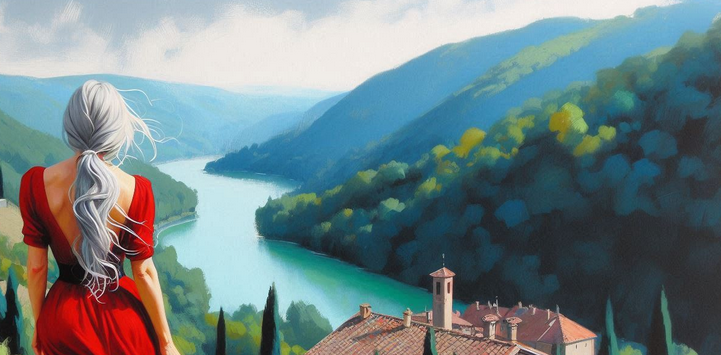
shared state
Let me break down the process of creating this chat application into three phases, explaining the shared state aspect and unique challenges compared to typical Arc/Mutex tutorials.
Phase 1: Setting Up the Basic Structure
In Plain English:
First, you’ll need to establish the foundational structure of your application. This means creating the basic server that can serve a webpage and handle WebSocket connections. The key difference from a typical Arc/Mutex tutorial is that you’re managing a long-lived service where connections come and go, rather than a one-time computation.
In Code:
- Set up your project structure with separate modules for:
- Main application entry point
- Chat state management
- Request handlers
- HTML templates
- Define your basic data structures:
- The
ChatMessage
struct to represent messages - The
ChatState
struct to manage shared state
- The
- Create routes for:
- Serving the HTML page
- Handling WebSocket connections
The shared state here is unique because it needs to:
- Persist across multiple connections
- Be accessible from any connection handler
- Be safely modifiable by concurrent connections
Phase 2: Implementing WebSocket Communication
In Plain English:
Next, you’ll set up the WebSocket communication system. This is where the real-time aspect comes in. Each connected client needs to be able to send messages that are broadcast to all other clients. The key challenge here is that you need to track active connections and manage their lifecycles.
In Code:
- Implement the WebSocket handler:
- Split the WebSocket into sender and receiver
- Register new connections in the shared state
- Set up a channel for sending messages to the client
- Create the message broadcasting system:
- Store all connected clients in the shared state
- Implement a method to broadcast messages to all connections
- Handle message serialization and deserialization
- Manage connection lifecycles:
- Add new connections to the shared state
- Remove connections when they disconnect
- Send chat history to new connections
The unique aspect here compared to basic Arc/Mutex tutorials is that you’re not just protecting a single piece of data – you’re managing a dynamic collection of connections that change over time. Each connection needs its own channel for communication, and you need to ensure that messages are correctly distributed to all active connections.
Phase 3: Front-End and User Experience
In Plain English:
Finally, you’ll create the front-end interface and improve the user experience. This means designing the HTML/CSS for the chat interface and implementing the JavaScript to interact with the WebSocket server.
In Code:
- Create the HTML template:
- Design the chat interface with CSS
- Set up the message display area
- Create the input form
- Implement the JavaScript:
- Connect to the WebSocket server
- Handle sending and receiving messages
- Display messages in the UI
- Enhance the user experience:
- Add username support
- Display connection status
- Add timestamps to messages
Deep Dive: The Shared State Aspect
The shared state in this project is fundamentally different from basic Arc/Mutex examples because:
- Dynamic Content: Unlike a static counter or simple data structure, your chat state contains a growing/shrinking collection of connections and messages.
- Lifecycle Management: You need to track when connections are established and closed, removing them from the state when they disconnect.
- Broadcast Pattern: Your state needs to actively push updates to all connections, not just respond to queries.
- Handling Concurrent Modifications: Since multiple connections might send messages simultaneously, you need to ensure that the state remains consistent.
Here’s how the shared state works:
pub struct ChatState {
// Store chat history
pub messages: Vec<ChatMessage>,
// Track active connections with their channels
pub connections: HashMap<ConnectionId, mpsc::UnboundedSender<Result<Message, warp::Error>>>,
}
When a new connection is established:
- Create a channel for sending messages to the client
- Add the sender half of the channel to the connections map
- Send the current message history to the client
When a message is received:
- Add it to the message history
- Broadcast it to all connections in the map
When a connection is closed:
- Remove it from the connections map
This approach allows you to handle many concurrent connections while maintaining a consistent view of the chat for all users. The Arc/Mutex pattern ensures that only one thread at a time can modify the state, preventing race conditions when updating the connections map or message history.
The unique challenges here involve managing the lifecycle of WebSocket connections and ensuring that messages are correctly distributed to all active connections, which goes beyond the typical Arc/Mutex patterns seen in basic tutorials.
.
├── chat.rs
├── handlers.rs
├── html
│ └── chat.html
└── main.rs
HTTP connection is upgraded to WebSocket
The WebSocket connection is established when the HTTP connection is upgraded to WebSocket, allowing for persistent, bidirectional communication.
Here’s how it works in more detail:
- Initial HTTP Request: The client (e.g., browser or app) sends a regular HTTP request to
/ws
. This request includes an Upgrade header indicating that the client wants to switch protocols (from HTTP to WebSocket). Example request:GET /ws HTTP/1.1 Host: localhost:3030 Upgrade: websocket Connection: Upgrade Sec-WebSocket-Key: <base64 key> Sec-WebSocket-Version: 13
- Server Upgrade: The server (your Warp handler) listens for requests on the
/ws
path. When it receives the WebSocket handshake request, it upgrades the connection to WebSocket usingws.on_upgrade
. This step turns the regular HTTP connection into a WebSocket connection, allowing for real-time, two-way communication. The server can then send and receive messages over this WebSocket connection, without the need for further HTTP requests. - WebSocket Connection: Once the connection is upgraded, the client and server can communicate directly and continuously through the WebSocket, allowing for low-latency, real-time interactions (e.g., chat messages, live updates).
In summary:
- The HTTP request to
/ws
starts the process. - The upgrade to WebSocket is what makes the route
ws_route
important—it is the trigger for real-time communication.
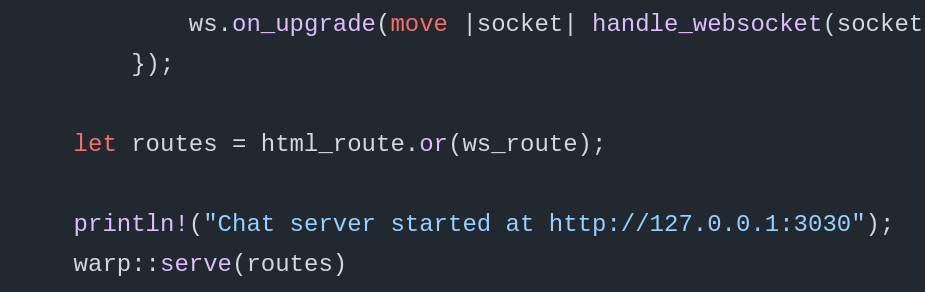