Rust Macros!
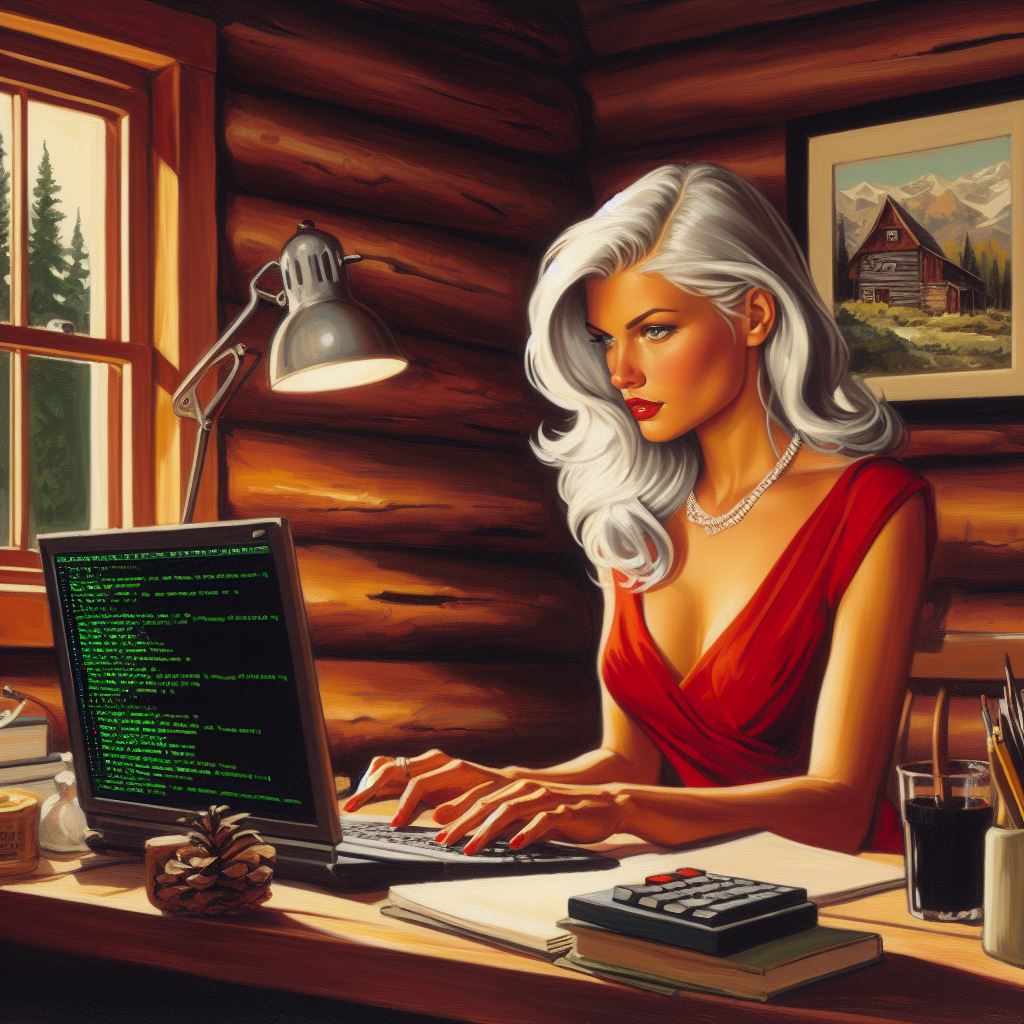
macro_rules!
Writing macros can be quite powerful, and they’re especially useful for generating repetitive code or performing complex transformations at compile time.
Step 1: Understanding Rust Macros
In Rust, macros are a way of writing code that writes other code, which is known as metaprogramming. Macros in Rust start with macro_rules!
and are followed by the name of the macro and a pattern-matching system to define its behavior.
Step 2: Basic Structure of a Macro
A simple Rust macro to convert miles to kilometers will look like this:
macro_rules! miles_to_km {
($miles:expr) => {
$miles * 1.60934
};
}
Explanation:
macro_rules! miles_to_km
: This defines a new macro calledmiles_to_km
.($miles:expr) => { ... }
: This specifies that the macro takes one argument,miles
, which is an expression (expr
). The macro will replace the expression with the code inside the braces{ ... }
.$miles * 1.60934
: This is the code that replaces the macro call. It multiplies the input by1.60934
(the conversion factor from miles to kilometers).
Step 3: Using the Macro
You can use the macro like a function in your code. Here’s a complete example:
// Define the macro
macro_rules! miles_to_km {
($miles:expr) => {
$miles * 1.60934
};
}
fn main() {
let distance_miles = 5.0;
let distance_km = miles_to_km!(distance_miles);
println!("{} miles is equal to {} kilometers", distance_miles, distance_km);
}
Additional parameters
Here we add the factor as and additional parameter
$factor:expr
macro_rules! miles_to_km {
($miles:expr, $factor:expr) => {{
let km = $miles * $factor;
println!("Converting {} miles to kilometers with factor {}: {} km", $miles, $factor, km);
km
}};
}
fn main() {
let miles = 5.0;
let factor = 1.60934; // More accurate conversion factor from miles to kilometers
let res = miles_to_km!(miles, factor);
println!("{:?}", res);
}
Using ‘ident’
Absolutely! Let’s simplify it:
Understanding ident
in Rust Macros
In Rust macros, ident
stands for identifier. An identifier is simply a name used to identify something in your code, like variable names, function names, struct names, etc. When you use ident
within a macro, you’re telling Rust to expect and match an identifier in that place when the macro is used.
Example:
Let’s say you have a macro that creates a simple function. You want to use ident
to specify the function name dynamically.
// Define a macro that creates a simple function
macro_rules! create_function {
// $func_name is an identifier (ident) parameter
($func_name:ident) => {
fn $func_name() {
println!("This is the {} function.", stringify!($func_name));
}
};
}
fn main() {
// Use the macro to create functions with different names
create_function!(hello);
create_function!(world);
// Call the generated functions
hello();
world();
}
This is the hello function.
This is the world function.
Explanation:
- Macro Definition (
create_function
):
macro_rules! create_function { ... }
defines a macro namedcreate_function
that takes one parameter.($func_name:ident)
declares a parameter named$func_name
which is expected to be an identifier (ident
).
- Macro Usage (
create_function!(...)
):
create_function!(hello);
andcreate_function!(world);
are two separate invocations of thecreate_function
macro.- In each invocation,
hello
andworld
are identifiers passed to the macro as$func_name
.
- Generated Functions:
- The macro generates functions named
hello
andworld
based on the identifiers provided. - Inside each generated function,
println!("This is the {} function.", stringify!($func_name));
prints a message indicating which function is being called.
- Output:
- When you run the program, it will print:
This is the hello function. This is the world function.
Summary:
- Identifiers: Identifiers (
ident
) in Rust macros are used to handle names dynamically. They allow you to generate code that can vary based on the identifiers passed to the macro. - Simplicity: Using
ident
in macros allows you to write more flexible and reusable code by parameterizing identifiers.
By understanding and using ident
effectively in Rust macros, you can leverage its power to generate code dynamically based on identifiers provided at compile-time. This capability enhances productivity and code clarity, especially in scenarios where you need to automate repetitive code patterns.