Rust Enum Representation Example
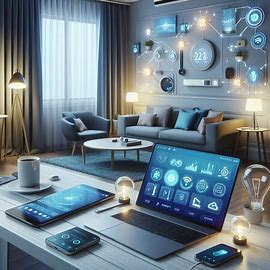
repr
Memory Efficiency with #[repr(u8)]
In Rust, enums are often used to represent a type that can take on a set of values, but by default, the underlying representation of an enum might be larger than needed. Using #[repr]
, we can specify exactly how we want the enum to be stored in memory.
Let’s explore two approaches to defining enums with a smaller representation, focusing on #[repr(u8)]
.
Example Without #[repr(u8)]
:
When we don’t specify a repr
attribute, Rust automatically selects an appropriate underlying type for the enum. By default, Rust uses a larger type like usize
, which may not be necessary if we only have a few variants.
enum Status {
Active, // Value will be 0 by default
Inactive, // Value will be 1 by default
Suspended, // Value will be 2 by default
}
fn main() {
let status = Status::Active;
println!("{:?}", status); // Prints "Active"
}
- What Happens: The compiler may choose a larger integer type (
usize
) to store the enum variants. This can be wasteful if the enum only needs a small set of values.
Example With #[repr(u8)]
(Idiomatic Approach):
Using the #[repr(u8)]
attribute, we can explicitly tell the compiler to store the enum’s discriminants as u8
(a single byte). This results in better memory efficiency.
#[repr(u8)] // Tells the compiler to store the enum discriminants as u8 (1 byte)
enum Status {
Active = 0, // Explicitly setting values for each variant
Inactive = 1,
Suspended = 2,
}
fn main() {
let status = Status::Active;
println!("{:?}", status); // Prints "Active"
}
- Why It’s Better: By specifying
#[repr(u8)]
, we ensure that each enum variant uses just 1 byte for storage. This reduces memory usage and makes the code cleaner and more predictable.
Manual Approach (Without #[repr(u8)]
):
You could manually specify the underlying type for each variant, but this approach is more error-prone and verbose.
enum Status {
Active = 0 as u8, // Manually casting to u8
Inactive = 1 as u8,
Suspended = 2 as u8,
}
fn main() {
let status = Status::Active;
println!("{:?}", status); // Prints "Active"
}
- What Happens: While this approach works, it introduces unnecessary boilerplate with manual type casting. It also makes the code harder to maintain.
Conclusion
#[repr(u8)]
is a cleaner, more idiomatic way to control how your enum is stored in memory.- It’s memory efficient and easy to read, without requiring manual casting for each variant.
- Manual casting may work but introduces unnecessary complexity and risks of mistakes.
- Publish the Page: Once you’ve added the content, click Publish to make the page live on your WordPress site.