Packet capture with pcap
Introduction to Packet Capture in Rust
If you have worked on IP networks you’ll know that packet capturing is a vital tool for network analysis, troubleshooting, and security monitoring.
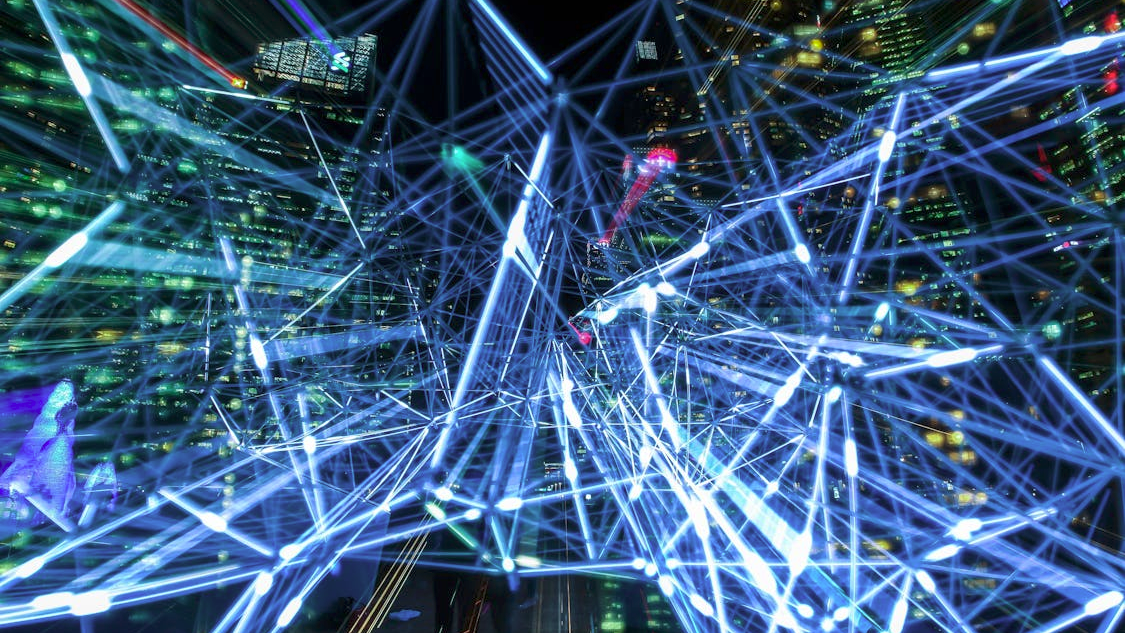
With Rust you can efficiently capture and analyze packets using libraries like pcap
.
This article explores creating a simple Rust application to capture network packets, filter specific traffic, and process data for “real-time” insights. The Cargo.toml file shows the set up, and the main.rs uses pcap
library, selecting the appropriate network interface, and applying filters for targeted analysis. Let’s look at the actual code!
The directory tree below is what your Rust project will look like, note the packets.log will only appear once you have run the code.
To gain access to low level Linux permissions with network interfaces run your code with sudo.
sudo cargo run
.
├── Cargo.lock
├── Cargo.toml
├── packets.log
├── src
│ └── main.rs
└── target
├── CACHEDIR.TAG
└── debug
[package]
name = "packcap"
version = "0.1.0"
edition = "2021"
[dependencies]
pcap = "2.2.0"
use std::fs::File;
use std::io::Write;
fn main() -> Result<(), Box<dyn std::error::Error>> {
let device = pcap::Device::lookup()?.expect("No device found");
println!("Using device: {}", device.name);
let mut cap = pcap::Capture::from_device(device)?
.promisc(true)
.snaplen(65535)
.timeout(1000)
.open()?;
cap.filter("tcp port 443", true)?;
let mut file = File::create("packets.log")?;
println!("Listening for packets...");
let mut packet_count = 0;
let max_packets = 10;
while let Ok(packet) = cap.next_packet() {
writeln!(file, "Packet {}: {:02x?}", packet_count, packet.data)?;
packet_count += 1;
if packet_count >= max_packets {
println!("Captured {} packets. Logged to packets.log.", max_packets);
break;
}
}
Ok(())
}
With this out of the way, so to speak, we can move on to look at how we can embed this in a larger program, as a “tool” ☺️
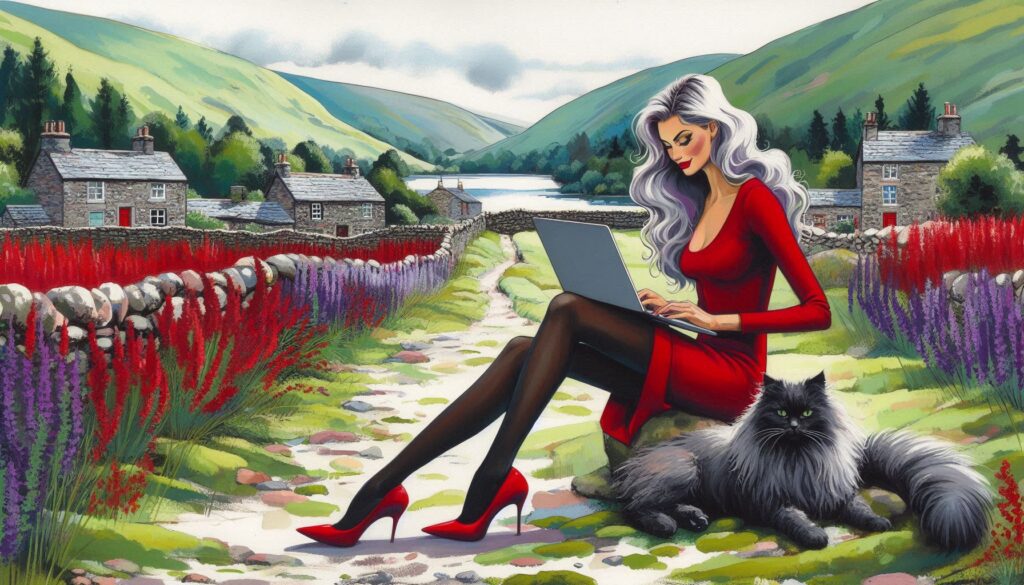