Llama3.2 local LLM query
> curl -s http://localhost:11434/api/generate -d '{ "model": "llama3.2", "prompt": "How are you today?", "stream": false }' | jq -r '.response'
I'm just a language model, so I don't have emotions or feelings like humans do. However, I'm functioning properly and ready to assist you with any questions or tasks you may have! How can I help you today?
> python3 llm.py
I'm just a language model, so I don't have emotions or feelings like humans do. However, I'm functioning properly and ready to help with any questions or tasks you may have! How can I assist you today?
> cat llm.py
import requests
import json
# URL and the payload for the request
url = "http://localhost:11434/api/generate"
data = {
"model": "llama3.2",
"prompt": "How are you today?",
"stream": False
}
# Send the POST request
response = requests.post(url, json=data)
# Parse the response JSON
response_json = response.json()
# Extract the 'response' field and print it
print(response_json.get('response'))
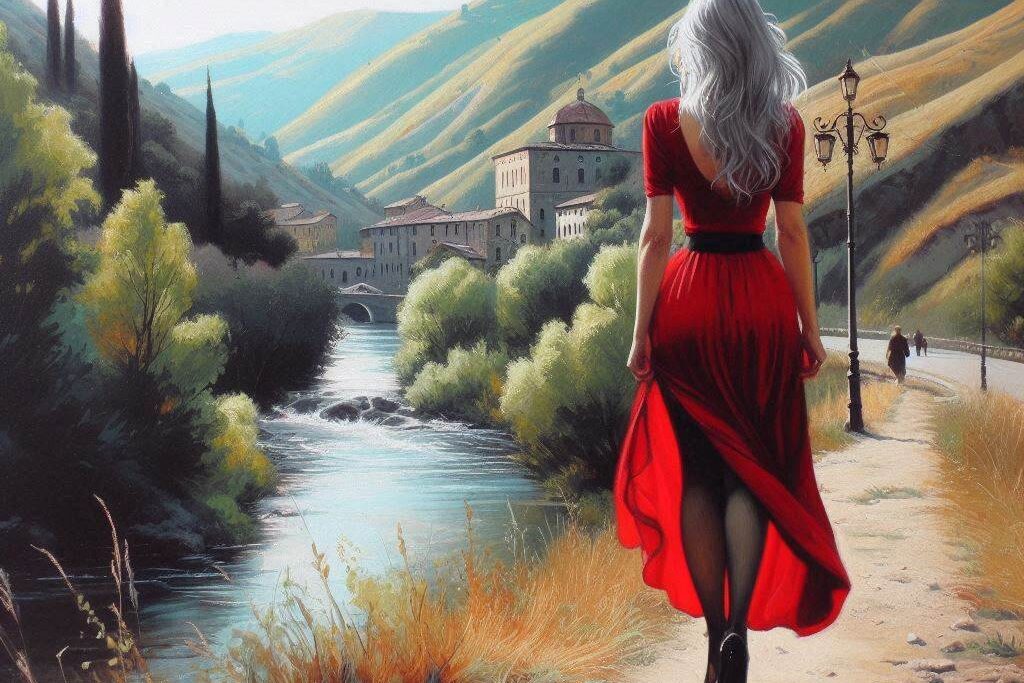
1. gossipsub
- Type: PubSub (Publish-Subscribe) Protocol
- Purpose: Efficient message propagation in P2P networks.
- Details:
- An advanced gossip-based pub-sub (publish-subscribe) protocol.
- Helps peers in a network subscribe to topics and receive messages without needing direct connections to all publishers.
- Uses mesh networks to optimize message distribution.
- Used in Ethereum, IPFS, and other decentralized applications.
2. mdns (Multicast DNS)
- Type: Peer Discovery Mechanism
- Purpose: Local peer discovery without centralized servers.
- Details:
- Uses local network broadcasting to find other nodes.
- Works well in LAN environments where nodes need to discover each other automatically.
- Not suitable for large-scale or public P2P networks.
3. noise
- Type: Encryption Protocol
- Purpose: Secure communication between peers.
- Details:
- Based on the Noise Protocol Framework.
- Provides authentication and encryption for secure peer connections.
- Used in Libp2p as a transport security layer.
- Ensures confidentiality, integrity, and forward secrecy.
4. swarm::{NetworkBehaviour, SwarmEvent}
- Type: Core Libp2p Networking Framework
- Purpose: Handles peer connections and events.
- Details:
NetworkBehaviour
:- Trait used to define how a node interacts with the network.
- Can include pub-sub, peer discovery, and custom behaviors.
SwarmEvent
:- Events generated by the Swarm (Libp2p’s networking layer).
- Includes new connections, disconnections, and incoming messages.
5. tcp
- Type: Transport Protocol
- Purpose: Reliable communication between peers.
- Details:
- Standard TCP transport for Libp2p.
- Provides ordered, reliable, and connection-oriented communication.
- Often used alongside yamux for multiplexing.
6. yamux
- Type: Multiplexing Protocol
- Purpose: Efficiently manage multiple streams over a single TCP connection.
- Details:
- Allows multiple logical connections (streams) over one physical TCP connection.
- Reduces overhead compared to opening multiple TCP connections.
- Alternative to other multiplexing protocols like mplex.
Summary Table
Name | Type | Purpose |
---|---|---|
gossipsub | PubSub | Message propagation in P2P networks. |
mdns | Discovery | Finds peers in local networks. |
noise | Encryption | Secure communication between peers. |
NetworkBehaviour, SwarmEvent | Core API | Defines P2P behavior and handles events. |
tcp | Transport | Reliable peer communication. |
yamux | Multiplexing | Multiple streams over one connection. |
This code sets up a basic libp2p networking configuration in Rust. It generates a unique peer identity, configures a secure transport layer using TCP, Noise encryption, and Yamux multiplexing. The code defines a custom network behavior combining Gossipsub (for pub/sub messaging) and mDNS (for local peer discovery), preparing the groundwork for a decentralized peer-to-peer network application with secure, encrypted communication.
#![allow(unused)]
use std::{
collections::hash_map::DefaultHasher,
error::Error,
hash::{Hash, Hasher},
time::Duration,
};
use futures::stream::StreamExt;
use libp2p::{
identity,
Transport,
PeerId,
gossipsub, mdns, noise,
swarm::{NetworkBehaviour, SwarmEvent},
tcp, yamux,
core::transport::Boxed,
};
use tokio::{io, io::AsyncBufReadExt, select};
use tracing_subscriber::EnvFilter;
// custom network behaviour struct that combines Gossipsub and Mdns.
#[derive(NetworkBehaviour)]
struct MyBehaviour {
gossipsub: gossipsub::Behaviour,
mdns: mdns::tokio::Behaviour,
}
#[tokio::main]
async fn main()->Result<(),Box<dyn std::error::Error>>{
let id_keys = identity::Keypair::generate_ed25519();
let peer_id = PeerId::from(id_keys.public());
println!("Local peer id: {:?}", peer_id);
// Configure the transport
let transport = build_transport(id_keys.clone());
Ok(())
}
// Function to build the transport layer
fn build_transport(id_keys: identity::Keypair) -> Boxed<(PeerId, libp2p::core::muxing::StreamMuxerBox)> {
// TCP transport with noise encryption and yamux multiplexing
let tcp_transport = tcp::tokio::Transport::new(tcp::Config::default());
let noise_config = noise::Config::new(&id_keys).expect("Signing libp2p-noise static key");
tcp_transport
.upgrade(libp2p::core::upgrade::Version::V1)
.authenticate(noise_config)
.multiplex(yamux::Config::default())
.boxed()
}
[package]
name = "libp2p2"
version = "0.1.0"
edition = "2021"
[dependencies]
tokio = { version = "1", features = ["full"] }
libp2p = { version = "0.53", features = ["tokio", "gossipsub", "mdns", "noise", "macros", "tcp", "yamux", "quic"] }
tracing-subscriber = { version = "0.3", features = ["env-filter"] }
futures = "0.3.31"
Typically, the next steps would involve:
- Creating the Gossipsub and mDNS behaviors with proper configuration
- Building a complete Swarm with the transport and behaviors
- Setting up a message topic for communication
- Adding a main event loop to handle network events
- Implementing user input handling for sending messages