Checksum Calculator in Rust
A checksum is often used over something like an MD5 hash for speed and simplicity when you only need to quickly verify data integrity or detect errors.
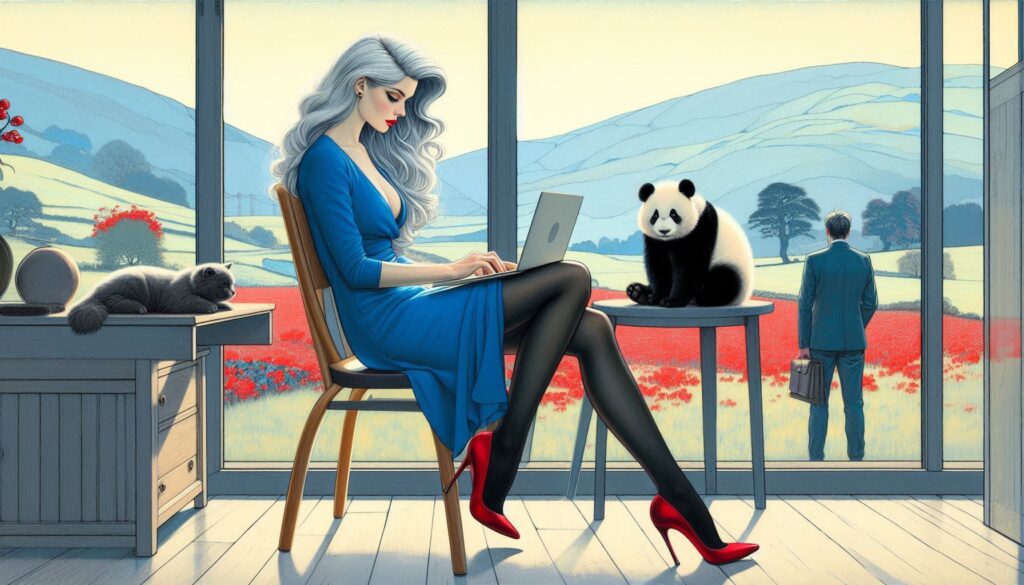
Just for some practice let’s guess (brute force) a checksum, we’ll provide the checksum and then work out what data would produce the checksum :
Note the use of if let some
If the checksum function returns some data, iteratively apply the XOR operation to each byte in the data to calculate the checksum.
Also note the use of fold – Folding is useful whenever you have a collection of something, and want to produce a single value from it.
fn guess_checksum(target_checksum: u8) -> Option<Vec<u8>> {
// Iterate through all combinations of two byte values (0x00 to 0xFF)
for i in 0..=255 {
for j in 0..=255 {
let data = vec![i, j];
// Calculate checksum using XOR operation on both bytes
let checksum = data.iter().fold(0u8, |acc, &byte| acc ^ byte);
if checksum == target_checksum {
return Some(data); // Return the first matching pair
}
}
}
None
}
fn main() {
// Let's run the function with a checksum we know (e.g., 0xd5 or 0xc7)
let target_checksum = 0xd5; // Target checksum in hexadecimal (213 in decimal)
if let Some(guessed_data) = guess_checksum(target_checksum) {
let checksum = guessed_data.iter().fold(0u8, |acc, &byte| acc ^ byte); // XOR checksum
println!("Found matching data: {:?}, with checksum: {:x}", guessed_data, checksum);
} else {
println!("Checksum not found");
}
}
#[cfg(test)]
mod tests {
use super::*;
#[test]
fn test_guess_checksum() {
let target_checksum = 0xd5; // Example checksum
let guessed_data = guess_checksum(target_checksum).expect("Checksum not found");
// Calculate checksum of guessed data using XOR to verify
let checksum = guessed_data.iter().fold(0u8, |acc, &byte| acc ^ byte);
assert_eq!(checksum, target_checksum);
assert_eq!(guessed_data.len(), 2); // Ensure the guessed data is of length 2 (two bytes)
println!("Test - Found matching data: {:?}, with checksum: {:x}", guessed_data, checksum);
}
}
Why would you use a checksum instead of a hash?
A checksum is often used over something like an MD5 hash for speed and simplicity when you only need to quickly verify data integrity or detect errors. Here’s why you might choose a checksum over MD5:
- Performance: Checksum algorithms (like XOR or simple sums) are computationally cheaper and faster than MD5. If you’re working in resource-constrained environments or need to process data quickly, a checksum is more efficient.
- Error Detection: A checksum is great for detecting simple errors (like single-bit flips) during transmission or storage. MD5, while more secure, is designed for cryptographic integrity and is better at detecting more complex data corruption but comes with a performance cost.
- Simplicity: Checksum methods are straightforward and easier to implement for tasks like quick data validation. MD5, on the other hand, requires more processing power and is more complex.
When to use MD5:
- Data Integrity: MD5 is more suitable when you need to detect even small, complex changes in data or when cryptographic security is important.
In short, checksums are faster and simpler, while MD5 is more robust and secure but slower.